Description
This technical indicator, in general, establishes upper and lower boundaries that are calculated by adding or subtracting a volatility component from a central tendency estimator. Channel indicators are widely used in technical analysis due to their ability to provide a wealth of information.
Many indicators present a central tendency estimator, which can be derived from various filters. However, the intriguing aspect lies in evaluating the efficiency of these tendencies and developing an efficient channel indicator using recursion based on the average values of the upper and lower boundaries of the indicator. This results in a new efficient filter that resembles calculating the average of the highest and lowest values.
Efficiency often entails recursion, which enables us to utilize past output values as input. This, in turn, allows for further price projection by incorporating additional price properties.
In this version of the custom indicator, we can derive various output results to identify price range values. In general, this indicator proves useful in identifying minimum price properties:
By monitoring the direction of the indicator's 'Average' component, we can determine the price direction and consider the 'Average' component as potential price support or resistance (refer to Figure 01).
By utilizing two indicators based on high and low price inputs, we can identify the upward price range (the zone between the two maximum components) and the downward price range (the zone between the two minimum components) (refer to Figure 02).
By calculating the indicator for the closing price over four or five different periods, we can identify potential breakouts of support or resistance and additional price momentum pressure for the new price direction (refer to Figure 03).
After analyzing the various benefits offered by this indicator, we recommend including it in your custom indicator toolkit.
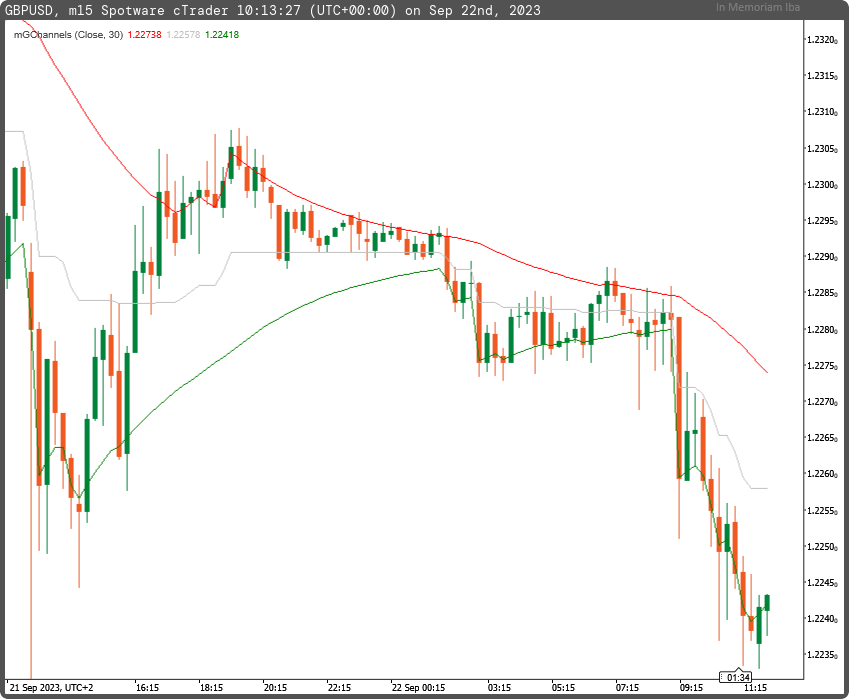
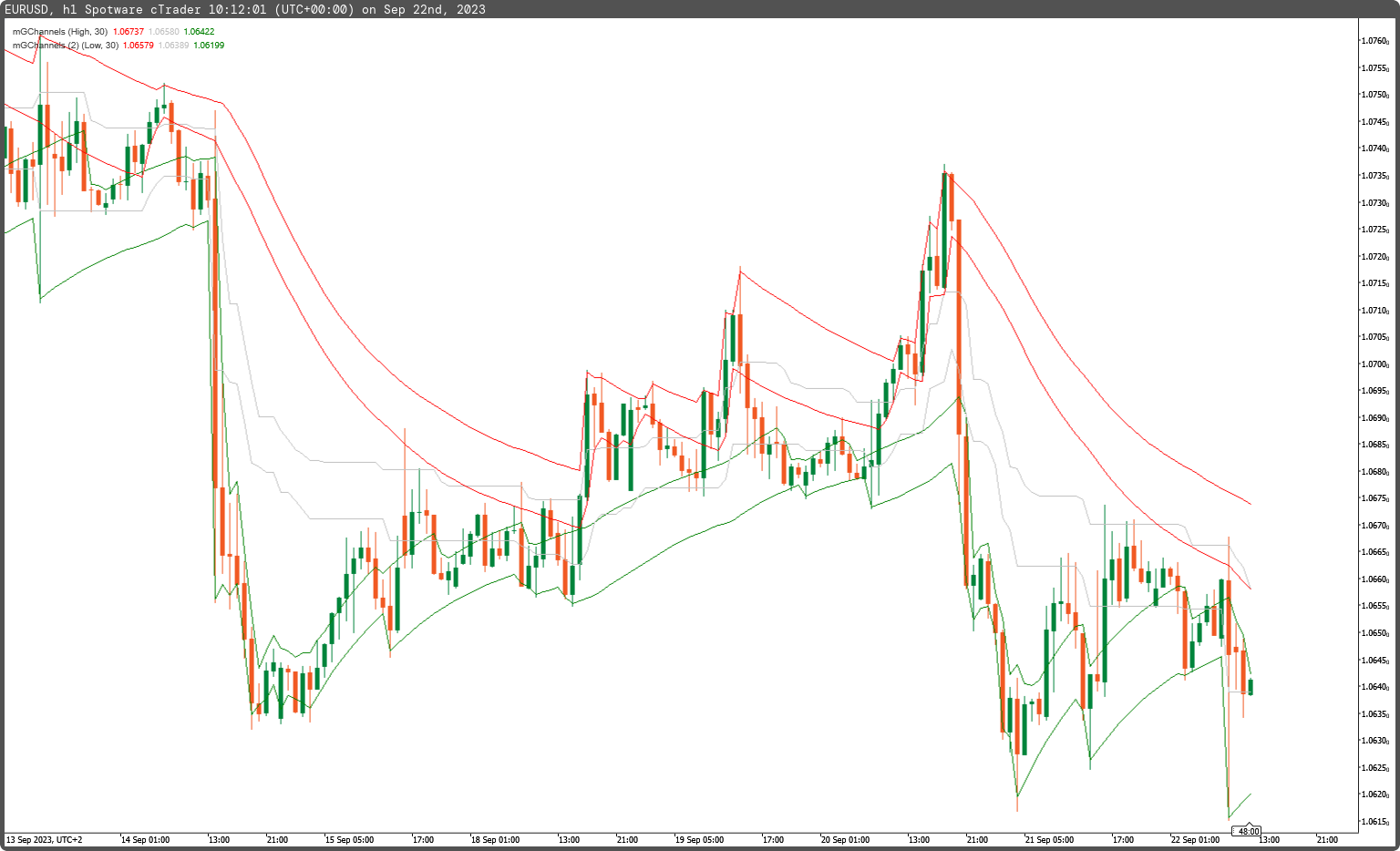
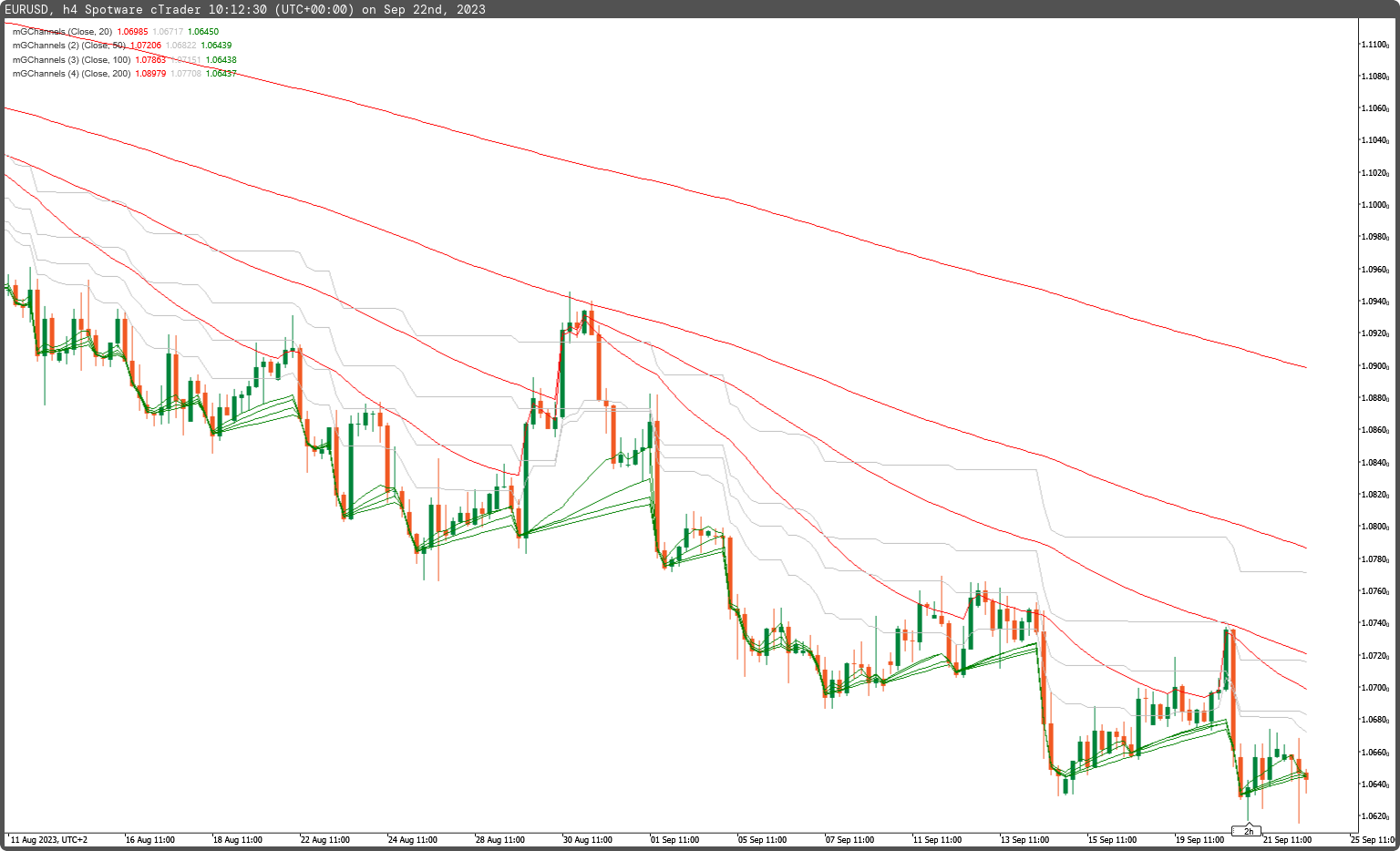
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class mGChannels : Indicator
{
[Parameter("Data Source (close)", DefaultValue = enumPriceTypes.Close)]
public enumPriceTypes inpPriceType { get; set; }
[Parameter("Peiods (20)", DefaultValue = 30)]
public int inpPeriods { get; set; }
[Output("Maximum", LineColor = "Red", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outGChannelMax { get; set; }
[Output("Average", LineColor = "Silver", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outGChannelAvg { get; set; }
[Output("Minimum", LineColor = "Green", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outGChannelMin { get; set; }
private IndicatorDataSeries _price, _delta, _gmax, _gmin, _gavg;
protected override void Initialize()
{
_price = CreateDataSeries();
_delta = CreateDataSeries();
_gmax = CreateDataSeries();
_gmin = CreateDataSeries();
_gavg = CreateDataSeries();
}
public override void Calculate(int i)
{
switch(inpPriceType)
{
case enumPriceTypes.Open:
_price[i] = Bars.OpenPrices[i];
break;
case enumPriceTypes.Close:
_price[i] = Bars.ClosePrices[i];
break;
case enumPriceTypes.High:
_price[i] = Bars.HighPrices[i];
break;
case enumPriceTypes.Low:
_price[i] = Bars.LowPrices[i];
break;
case enumPriceTypes.Median:
_price[i] = Bars.MedianPrices[i];
break;
case enumPriceTypes.Typical:
_price[i] = Bars.TypicalPrices[i];
break;
case enumPriceTypes.Weighted:
_price[i] = Bars.WeightedPrices[i];
break;
default:
_price[i] = Bars.ClosePrices[i];
break;
}
_delta[i] = i>1 ? (_gmax[i-1] - _gmin[i-1]) / inpPeriods : (Bars.HighPrices[i] - Bars.LowPrices[i]) / inpPeriods;
_gmax[i] = Math.Max(_price[i], (i>1 ? _gmax[i-1] : Bars.HighPrices[i])) - _delta[i];
_gmin[i] = Math.Min(_price[i], (i>1 ? _gmin[i-1] : Bars.LowPrices[i])) + _delta[i];
_gavg[i] = (_gmax[i] + _gmin[i]) / 2;
outGChannelMax[i] = _gmax[i];
outGChannelMin[i] = _gmin[i];
outGChannelAvg[i] = _gavg[i];
}
}
public enum enumPriceTypes
{
Open,
Close,
High,
Low,
Median,
Typical,
Weighted
}
}
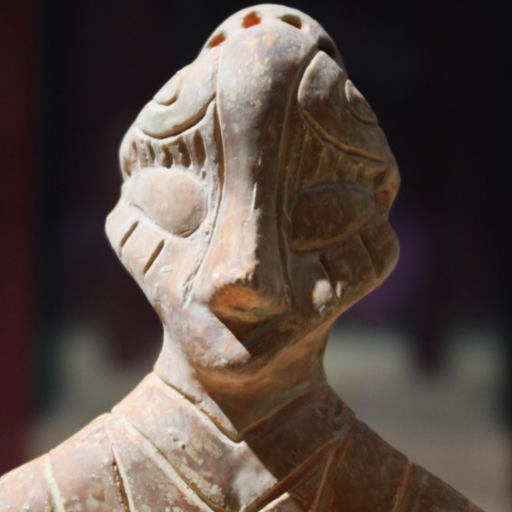
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mGChannels.algo
- Rating: 5
- Installs: 626
- Modified: 22/09/2023 10:19