Description
The RelativeStrengthRank custom indicator, appears to be a momentum-based measure that takes into account both short-term and long-term price changes relative to the 10-day Average True Range (ATR). Here's how you can use it and a potential trading strategy:
Indicator Formula:
Relative Strength Rank = ((Long Term Price Change + Short Term Price Change) / 2) / 10-Day ATR
Calculation:
- Calculate the 10-day ATR (Average True Range). This measures market volatility over the past 10 trading days.
- Calculate both short-term and long-term price changes. These changes can be calculated using various methods (e.g., simple price differences, moving averages).
- Apply the RSR formula: ((Long Term Price Change + Short Term Price Change) / 2) / 10-Day ATR.
Interpretation:
- The RSR value represents the relative strength of the asset compared to its own volatility (ATR). A higher RSR suggests stronger momentum relative to volatility, while a lower RSR indicates weaker momentum relative to volatility.
Trading Strategy:
Here's a basic trading strategy you could consider using the RSR indicator:
Long Entry:
- Look for RSR values significantly above 1.0, indicating strong positive momentum relative to volatility.
- Consider entering a long (buy) position when RSR crosses above a certain threshold, such as 1.2 or 1.5.
- Use additional technical analysis tools or price confirmation to support your long entry decision.
- Implement risk management techniques like setting stop-loss orders to limit potential losses.
Short Entry:
- Look for RSR values significantly below 1.0, indicating strong negative momentum relative to volatility.
- Consider entering a short (sell) position when RSR crosses below a certain threshold, such as 0.8 or 0.7.
- Again, use additional technical analysis or price confirmation to support your short entry decision.
- Implement risk management strategies to protect your capital.
Additional Considerations:
- Always consider the broader market context, including trend direction and key support/resistance levels.
- Be aware that no indicator is foolproof, and it's essential to use proper risk management and position sizing to protect your capital.
- Consider using the RSR indicator in conjunction with other technical indicators or trading strategies to improve the accuracy of your trades.
Remember that this is a basic strategy outline. Actual trading strategies should be thoroughly backtested and adjusted to fit your risk tolerance, time frame, and specific trading goals. Additionally, market conditions can change, so it's essential to stay adaptable and continuously evaluate the effectiveness of your strategy.
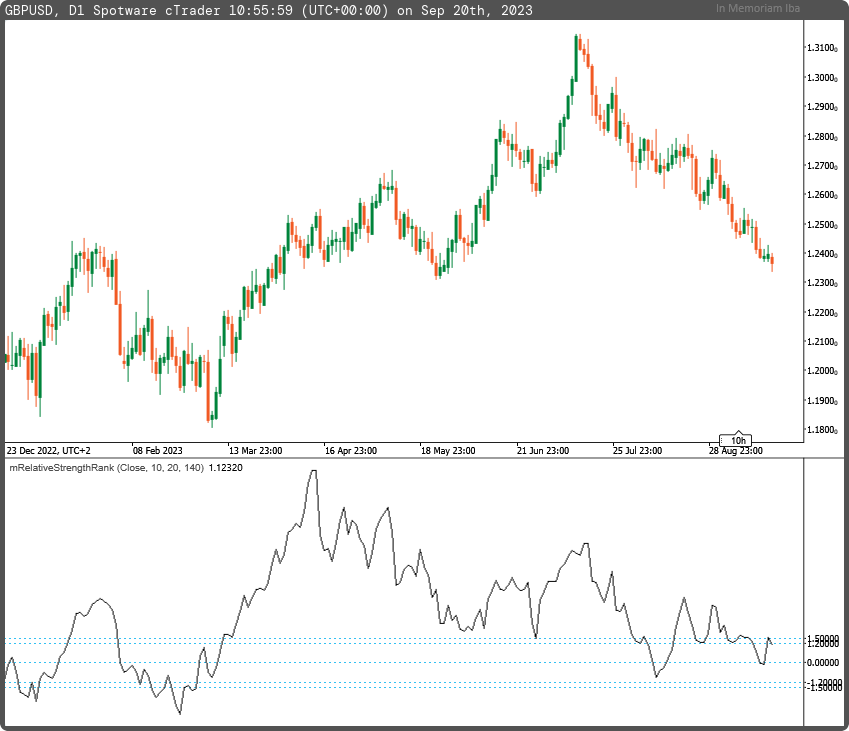
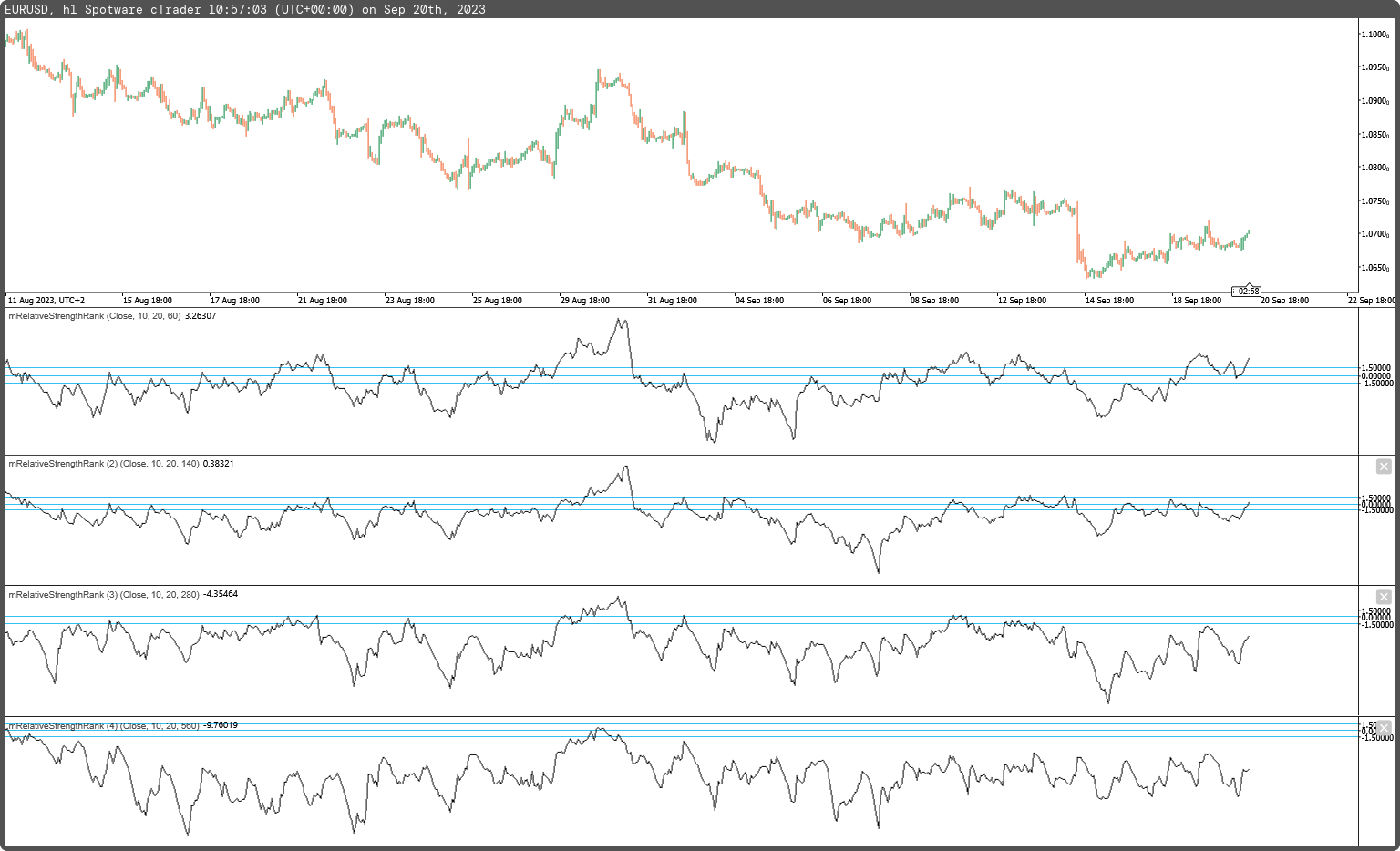
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Levels(-1.5, -1.2, 0, +1.2, +1.5)]
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class mRelativeStrengthRank : Indicator
{
[Parameter("Data Source (close)", DefaultValue = enumPriceTypes.Close)]
public enumPriceTypes inpPriceType { get; set; }
[Parameter("Range Period (10)", DefaultValue = 10)]
public int inpPeriodRange { get; set; }
[Parameter("Fast Period (20)", DefaultValue = 20)]
public int inpPeriodFast { get; set; }
[Parameter("Slow Period (140)", DefaultValue = 140)]
public int inpPeriodSlow { get; set; }
[Output("Relative Strength Rank", LineColor = "Black", PlotType = PlotType.Line, Thickness = 1)]
public IndicatorDataSeries outRSR { get; set; }
private AverageTrueRange _atr;
private IndicatorDataSeries _price, _deltafast, _deltaslow, _rsr;
protected override void Initialize()
{
_atr = Indicators.AverageTrueRange(inpPeriodRange, MovingAverageType.Exponential);
_price = CreateDataSeries();
_deltafast = CreateDataSeries();
_deltaslow = CreateDataSeries();
_rsr = CreateDataSeries();
}
protected override void OnDestroy()
{
_rsr = null;
_deltaslow = null;
_deltafast = null;
_price = null;
_atr = null;
base.OnDestroy();
}
public override void Calculate(int i)
{
switch(inpPriceType)
{
case enumPriceTypes.Open:
_price[i] = Bars.OpenPrices[i];
break;
case enumPriceTypes.Close:
_price[i] = Bars.ClosePrices[i];
break;
case enumPriceTypes.High:
_price[i] = Bars.HighPrices[i];
break;
case enumPriceTypes.Low:
_price[i] = Bars.LowPrices[i];
break;
case enumPriceTypes.Median:
_price[i] = Bars.MedianPrices[i];
break;
case enumPriceTypes.Typical:
_price[i] = Bars.TypicalPrices[i];
break;
case enumPriceTypes.Weighted:
_price[i] = Bars.WeightedPrices[i];
break;
default:
_price[i] = Bars.ClosePrices[i];
break;
}
_deltafast[i] = i>inpPeriodFast ? _price[i] - _price[i-inpPeriodFast] : _price[i] - (Bars.ClosePrices[i] + Bars.TypicalPrices[i]) * 0.5;
_deltaslow[i] = i>inpPeriodSlow ? _price[i] - _price[i-inpPeriodSlow] : _price[i] - (Bars.ClosePrices[i] + Bars.TypicalPrices[i]) * 0.5;
_rsr[i] = ((_deltaslow[i] + _deltafast[i]) / 2) / _atr.Result[i];
outRSR[i] = _rsr[i];
}
}
public enum enumPriceTypes
{
Open,
Close,
High,
Low,
Median,
Typical,
Weighted
}
}
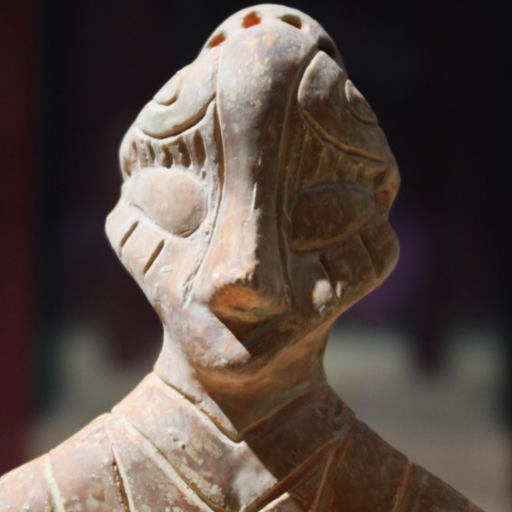
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mRelativeStrengthRank.algo
- Rating: 5
- Installs: 621
- Modified: 20/09/2023 10:59