Description
The HyperTrend indicator aims to offer a real-time estimate of the underlying linear trend in the price. Support and resistance levels are constructed from this estimate, which can provide trading opportunities within the overall trend while predicting the next price level within the channel.
Many tools that display lines on a chart are either prone to backpainting or repainting. This indicator aims to provide a dependable real-time method for estimating linear trends in price, thereby enhancing traders' decision-making processes when trading price trends. This is why it's called 'HyperTrend'.
For further ideas, please consider exploring the 'Angle Trend Force' indicator. This indicator includes a novel approach to understanding price angles from various perspectives.
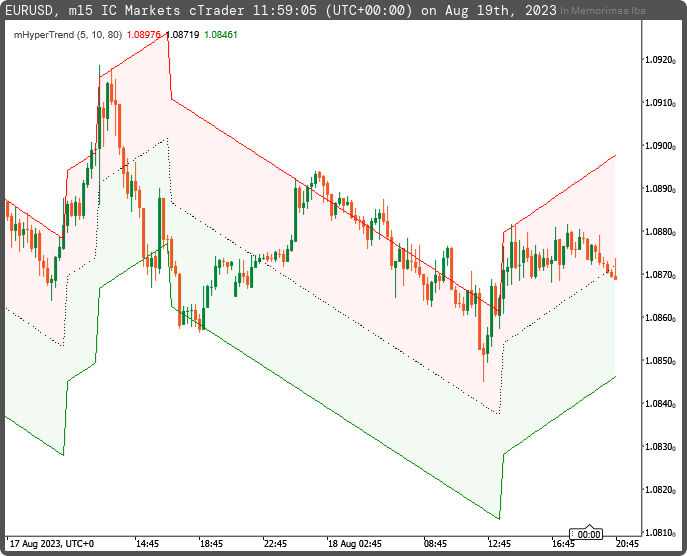
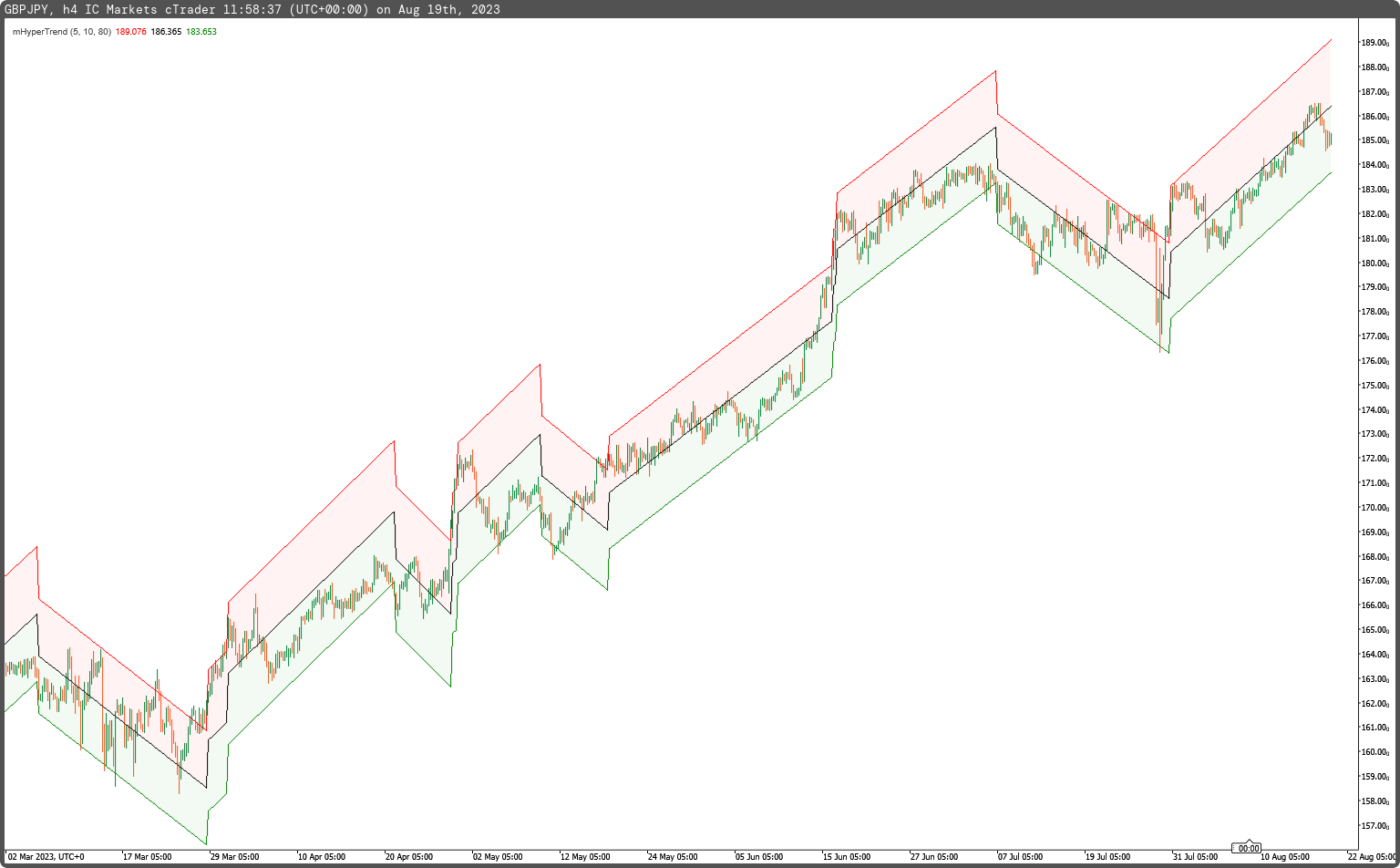
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Cloud("Upper Channel", "Middle Channel", FirstColor = "Red", Opacity = 0.05)]
[Cloud("Middle Channel", "Lower Channel", FirstColor = "Green", Opacity = 0.05)]
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class mHyperTrend : Indicator
{
[Parameter("Multiplicative Factor (5)", DefaultValue = 5, MinValue = 1)]
public int inpMultiplicativeFactor { get; set; }
[Parameter("Slope (10)", DefaultValue = 10, MinValue = 0)]
public int inpSlope { get; set; }
[Parameter("Width % (80)", DefaultValue = 80, MinValue = 0, MaxValue = 100)]
public int inpWidth { get; set; }
[Output("Upper Channel", LineColor = "Red", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outUpper { get; set; }
[Output("Middle Channel", LineColor = "Black", PlotType = PlotType.Line, LineStyle = LineStyle.Dots, Thickness = 1)]
public IndicatorDataSeries outMiddle { get; set; }
[Output("Lower Channel", LineColor = "Green", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outLower { get; set; }
private AverageTrueRange _iatr;
private IndicatorDataSeries _delta, _hold, _atr, _avg, _upper, _lower;
protected override void Initialize()
{
_iatr = Indicators.AverageTrueRange(200, MovingAverageType.Simple);
_delta = CreateDataSeries();
_hold = CreateDataSeries();
_atr = CreateDataSeries();
_avg = CreateDataSeries();
_upper = CreateDataSeries();
_lower = CreateDataSeries();
}
public override void Calculate(int i)
{
_delta[i] = i>1 ? _delta[i-1] : 1.0;
_hold[i] = i>1 ? _hold[i-1] : 0.0;
_atr[i] = (i>200 ? _iatr.Result[i] : Bars.HighPrices[i] - Bars.LowPrices[i]) * (double)inpMultiplicativeFactor;
_avg[i] = Math.Abs(Bars.ClosePrices[i] - (i>1 ? _avg[i-1] : 1)) > _atr[i]
? (Bars.ClosePrices[i] + (i>1 ? _avg[i-1] : 1)) / 2
: (i>1 ? _avg[i-1] : 1) + _delta[i] * (_hold[i] / (double)inpMultiplicativeFactor / (double)inpSlope);
_delta[i] = Math.Sign(_avg[i] - _avg[i-1]);
_hold[i] = _delta[i] != _delta[i-1] ? _atr[i] : _hold[i];
_upper[i] = _avg[i] + ((double)inpWidth / 100) * _hold[i];
_lower[i] = _avg[i] - ((double)inpWidth / 100) * _hold[i];
outUpper[i] = _upper[i];
outMiddle[i] = _avg[i];
outLower[i] = _lower[i];
}
}
}
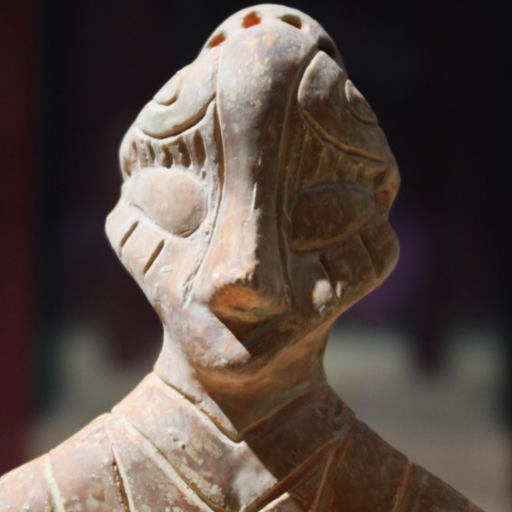
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mHyperTrend.algo
- Rating: 5
- Installs: 826
This HyperTrend indicator seems to offer a promising way to estimate real-time linear trends in price. By deriving support and resistance levels from this estimation, traders can potentially identify opportunities within the broader trend and even predict future price levels within the established channel.
One of the standout features of this indicator is its focus on providing a reliable real-time method for estimating linear trends in price. This could be a game-changer, as many other tools often struggle with backpainting or repainting, compromising their accuracy and effectiveness.
The 'HyperTrend' name is quite fitting considering its aim to offer a dependable approach to trend estimation. It seems to be a step towards enhancing traders' decision-making processes when it comes to riding price trends.
For those intrigued by this indicator's approach, there's an additional suggestion to check out the 'Angle Trend Force' indicator. This could open up new perspectives on price angles and further enrich your trading toolkit.
Keep in mind that this code snippet appears to be a part of the indicator's implementation using cAlgo. If you're a trader interested in leveraging this indicator, it might be worth diving into the code to understand how it works under the hood.
Remember, trading involves risks, and it's always a good idea to thoroughly test any indicator or strategy before using it in your live trading activities.