An update for this algorithm is currently pending moderation. Please revisit this page shortly to access the algorithm's latest version.
Description
This indicator can show you breakout.
He make the Stop Loss on BarsBack and you can defind it.
Have fun, and for any collaboration, contact me !!!
On telegram : https://t.me/nimi012 (direct messaging)
On Discord: https://discord.gg/jNg7BSCh (I advise you to come and see, Money management strategies and Signals Strategies !)
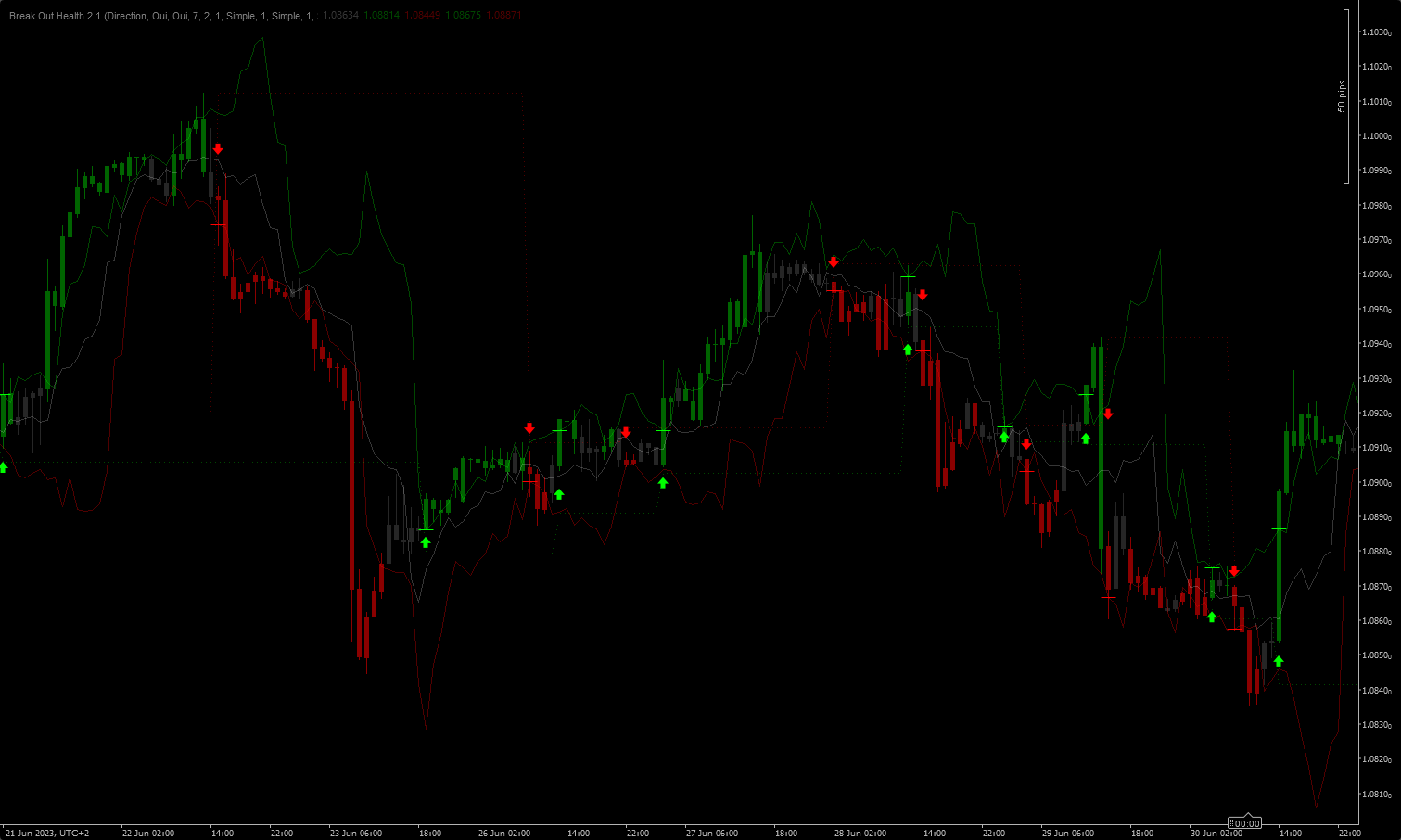
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Indicator(IsOverlay = true, AccessRights = AccessRights.None)]
public class BreakoutPivotPoints : Indicator
{
[Parameter("SL BarsBack ", DefaultValue = EnumEnterType.Direction, Group = "Base Setting ")]
public EnumEnterType EnterType { get; set; }
[Parameter("Show Bars Colors", DefaultValue = true, Group = "Base Setting ")]
public bool ShowBarsColors { get; set; }
[Parameter("Show Enter Colors", DefaultValue = true, Group = "Base Setting ")]
public bool ShowEnterColors { get; set; }
[Parameter("Periods Break Line", DefaultValue = 7, Group = "Base Setting ")]
public int PeriodsBreakLine { get; set; }
[Parameter("SL BarsBack ", DefaultValue = 2, Group = "Base Setting ")]
public int LookBackTriangleSL { get; set; }
[Parameter("Smooth Resistance", DefaultValue = 1, Group = "Smooth Break Out Setting ")]
public int SmoothResistance { get; set; }
[Parameter("Smooth Resistance Type ", DefaultValue = MovingAverageType.Simple, Group = "Smooth Break Out Setting ")]
public MovingAverageType SmoothResistanceType { get; set; }
[Parameter("Smooth Support", DefaultValue = 1, Group = "Smooth Break Out Setting ")]
public int SmoothSupport { get; set; }
[Parameter("Smooth Support Type ", DefaultValue = MovingAverageType.Simple, Group = "Smooth Break Out Setting ")]
public MovingAverageType SmoothSupportType { get; set; }
[Parameter("Smooth Support", DefaultValue = 1, Group = "Smooth Break Out Setting ")]
public int SmoothPivot { get; set; }
[Parameter("Smooth Support Type ", DefaultValue = MovingAverageType.Simple, Group = "Smooth Break Out Setting ")]
public MovingAverageType SmoothPivotType { get; set; }
[Output("PivotPoint", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, LineColor = "36FFFFFF")]
public IndicatorDataSeries PivotPoint { get; set; }
[Output("Resistance", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, LineColor = "4701FF01")]
public IndicatorDataSeries Resistance { get; set; }
[Output("Support", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, LineColor = "50FE0000")]
public IndicatorDataSeries Support { get; set; }
[Output("Buy Stop Loss ", PlotType = PlotType.DiscontinuousLine, LineStyle = LineStyle.DotsRare, LineColor = "4701FF01")]
public IndicatorDataSeries SlResistance { get; set; }
[Output("Sell Stop Loss", PlotType = PlotType.DiscontinuousLine, LineStyle = LineStyle.DotsRare, LineColor = "50FE0000")]
public IndicatorDataSeries SlSupport { get; set; }
private IndicatorDataSeries ResR, ResS, Pivot;
private MovingAverage SmoothR, SmoothS, SmoothP;
private int direction;
public enum EnumEnterType
{
Direction,
Renforcement_position
}
protected override void Initialize()
{
ResR = CreateDataSeries();
ResS = CreateDataSeries();
Pivot = CreateDataSeries();
SmoothR = Indicators.MovingAverage(ResR, SmoothResistance, SmoothResistanceType);
SmoothS = Indicators.MovingAverage(ResS, SmoothSupport, SmoothSupportType);
SmoothP = Indicators.MovingAverage(Pivot, SmoothPivot, SmoothPivotType);
direction = 0;
}
public override void Calculate(int index)
{
if (index < PeriodsBreakLine)
return;
double previousLowestLow = double.MaxValue;
double previousHighestHigh = double.MinValue;
for (int i = 1; i <= PeriodsBreakLine; i++)
{
double low = Bars.LowPrices[index - i];
double high = Bars.HighPrices[index - i];
previousLowestLow = Math.Min(previousLowestLow, low);
previousHighestHigh = Math.Max(previousHighestHigh, high);
}
Pivot[index] = (previousLowestLow + previousHighestHigh + Bars.OpenPrices[index - PeriodsBreakLine]) / 3;
PivotPoint[index] = SmoothP.Result.Last(0);
ResR[index] = (2 * PivotPoint[index]) - previousLowestLow;
ResS[index] = (2 * PivotPoint[index]) - previousHighestHigh;
Resistance[index] = SmoothResistance < 2 ? ResR[index - 1] : SmoothR.Result.Last(0);
Support[index] = SmoothSupport < 2 ? ResS[index - 1] : SmoothS.Result.Last(0);
if (Bars.HighPrices.Last(0) > Resistance[index])
{
if (EnterType == EnumEnterType.Direction && direction == 0)
direction = -1;
if (ShowBarsColors)
Chart.SetBarColor(index, Color.DarkGreen);
if ((EnterType == EnumEnterType.Direction && direction == -1) || (EnterType == EnumEnterType.Renforcement_position && direction == -1 || direction == 0))
{
SlResistance[index] = LookBackTriangleSL == 0 ? Bars.LowPrices.Last(0) : Bars.LowPrices.Minimum(LookBackTriangleSL + 1);
if (ShowEnterColors)
Chart.DrawIcon("Up" + index, ChartIconType.UpArrow, index, Bars.LowPrices.Last(0) - ((Bars.HighPrices.Last(1) - Bars.LowPrices.Last(1)) / 2), "Lime");
if (ShowEnterColors)
Chart.DrawTrendLine("Enter Up" + index, index - 1, Bars.OpenPrices.Last(0) > Resistance[index] ? Bars.OpenPrices.Last(0) : Resistance[index], index + 1, Bars.OpenPrices.Last(0) > Resistance[index] ? Bars.OpenPrices.Last(0) : Resistance[index], "Lime");
direction = 1;
}
if (direction == 1)
{
SlResistance[index + 1] = SlResistance[index];
}
}
else if (Bars.LowPrices.Last(0) < Support[index])
{
if (EnterType == EnumEnterType.Direction && direction == 0)
direction = 1;
if (ShowBarsColors)
Chart.SetBarColor(index, Color.DarkRed);
if ((EnterType == EnumEnterType.Direction && direction == 1) || (EnterType == EnumEnterType.Renforcement_position && direction == 1 || direction == 0))
{
SlSupport[index] = LookBackTriangleSL == 0 ? Bars.HighPrices.Last(0) : Bars.HighPrices.Maximum(LookBackTriangleSL + 1);
if (ShowEnterColors)
Chart.DrawIcon("Down" + index, ChartIconType.DownArrow, index, Bars.HighPrices.Last(0) + ((Bars.HighPrices.Last(1) - Bars.LowPrices.Last(1)) / 2), "Red");
if (ShowEnterColors)
Chart.DrawTrendLine("Enter Down" + index, index - 1, Bars.OpenPrices.Last(0) < Support[index] ? Bars.OpenPrices.Last(0) : Support[index], index + 1, Bars.OpenPrices.Last(0) < Support[index] ? Bars.OpenPrices.Last(0) : Support[index], "Red");
direction = -1;
}
if (direction == -1)
{
SlSupport[index + 1] = SlSupport[index];
}
}
else
{
if (ShowBarsColors)
Chart.SetBarColor(index, "FF262626");
if (EnterType == EnumEnterType.Renforcement_position)
direction = 0;
}
SlResistance[index + 1] = SlResistance[index];
SlSupport[index + 1] = SlSupport[index];
}
}
}
YE
YesOrNot
Joined on 10.10.2022 Blocked
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Break Out Health 2.1.algo
- Rating: 5
- Installs: 739
- Modified: 18/08/2023 22:48
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.