Description
Hanning FIR Filter
The Hanning Finite Impulse Response (FIR) Filter is a fundamental digital signal processing tool used to manipulate and enhance signals in various applications. As an end user, understanding the basic concepts of the Hanning FIR Filter can help you make informed decisions when working with digital signal processing tasks.
What is the Hanning FIR Filter?
The Hanning FIR Filter is a type of digital filter used to modify the characteristics of a signal. It falls under the category of Finite Impulse Response filters, which means its output is determined by a finite number of input samples.
Key Characteristics:
Window Function: The Hanning FIR Filter uses a Hanning window function, also known as the Hann window or raised cosine window, to shape the impulse response of the filter. This windowing technique helps mitigate the issue of spectral leakage in frequency analysis.
Frequency Response: The filter's frequency response exhibits a main lobe with lower side lobes. This characteristic makes the Hanning FIR Filter useful for applications where attenuation of side lobes is important.
Passband and Stopband: The Hanning FIR Filter is designed to pass certain frequency components (passband) while attenuating others (stopband), according to the desired filter specifications.
User Benefits:
Improved Signal Quality: By applying the Hanning FIR Filter, you can enhance the quality of a signal by removing unwanted noise or unwanted frequency components.
Frequency Analysis: The Hanning FIR Filter can be used to isolate specific frequency ranges of a signal, aiding in tasks such as signal separation and analysis.
Customizable Design: As an end user, you can adjust the filter parameters to tailor its behavior according to your specific signal processing requirements.
Limitations:
Finite Impulse Response: The Hanning FIR Filter has a finite memory, which means its ability to capture long-duration effects in a signal is limited compared to Infinite Impulse Response (IIR) filters.
Design Complexity: Designing an optimal Hanning FIR Filter requires some understanding of filter design principles, including trade-offs between filter order, passband ripple, and stopband attenuation.
Applications:
Audio Processing: The Hanning FIR Filter is used in audio applications to remove noise, enhance speech clarity, and create audio effects.
Image Processing: In image processing, the filter can be employed for edge enhancement and noise reduction.
Communication Systems: The Hanning FIR Filter helps in improving the quality of transmitted signals and reducing interference in communication systems.
Conclusion:
In conclusion, the Hanning FIR Filter is a valuable tool in the realm of digital signal processing. As an end user, understanding its basic principles, characteristics, and applications can empower you to effectively manipulate and improve signals in various domains.
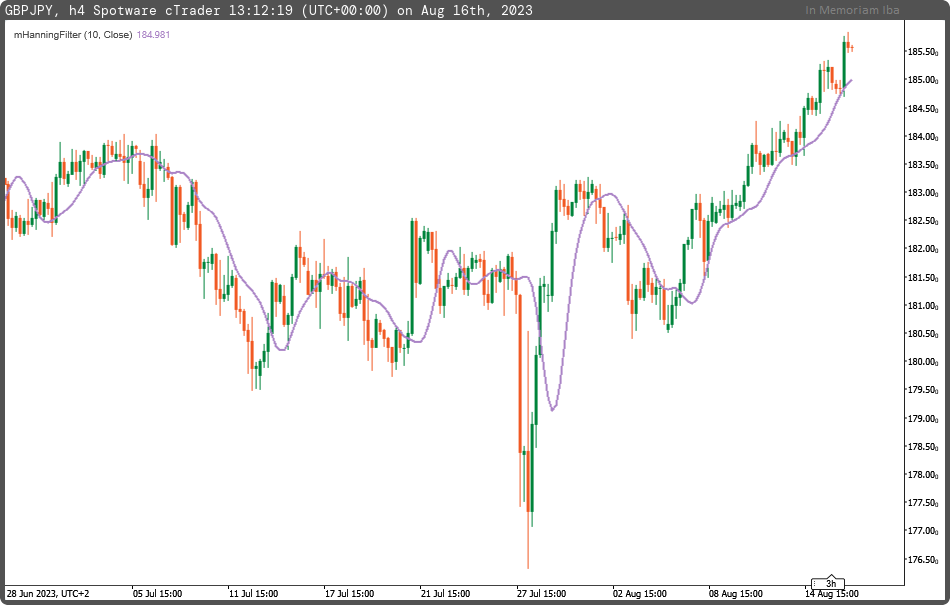
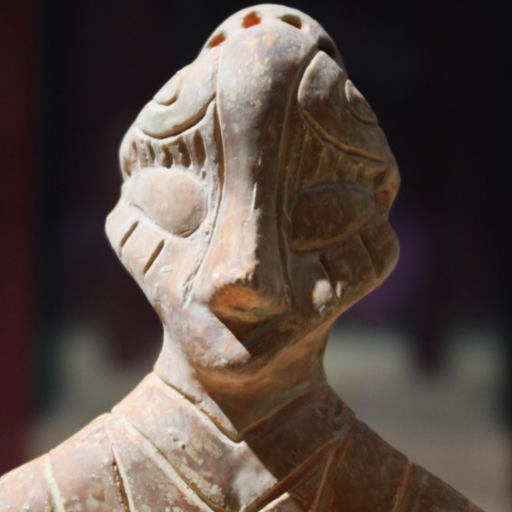
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mHanningFilter.algo
- Rating: 5
- Installs: 433
- Modified: 16/08/2023 13:20
Comments
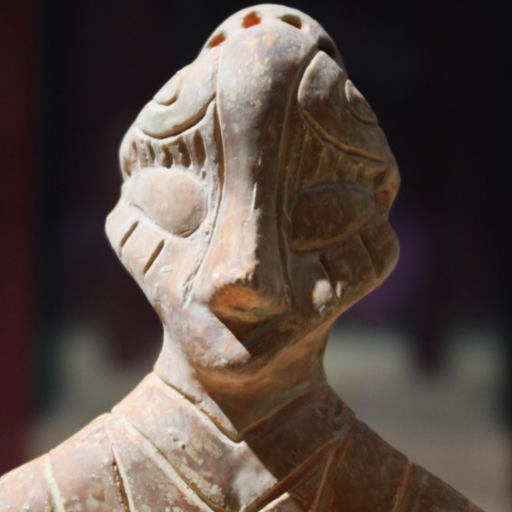