An update for this algorithm is currently pending moderation. Please revisit this page shortly to access the algorithm's latest version.
Description
In the article The Compare Price Momentum Oscillator (CPMO), author Vitali Apirine reintroduces us to the DecisionPoint PMO, originally developed by Carl Swenlin, and presents a new way to use it for comparing the relative momentum of two different securities.
Trading signals can be derived in several ways, including momentum, signal line, and zero-line crossovers.
One of the most advanced and statistically productive strategies is to utilize the cross components for going Long when above the zero value, and for going Short when below the zero value.
You can also find the Volume Price Momentum indicator at the following link: https://ctrader.com/algos/indicators/show/3543
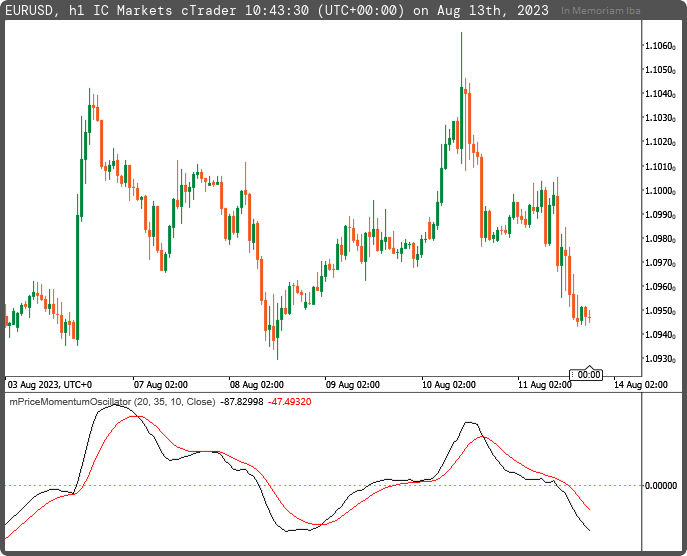
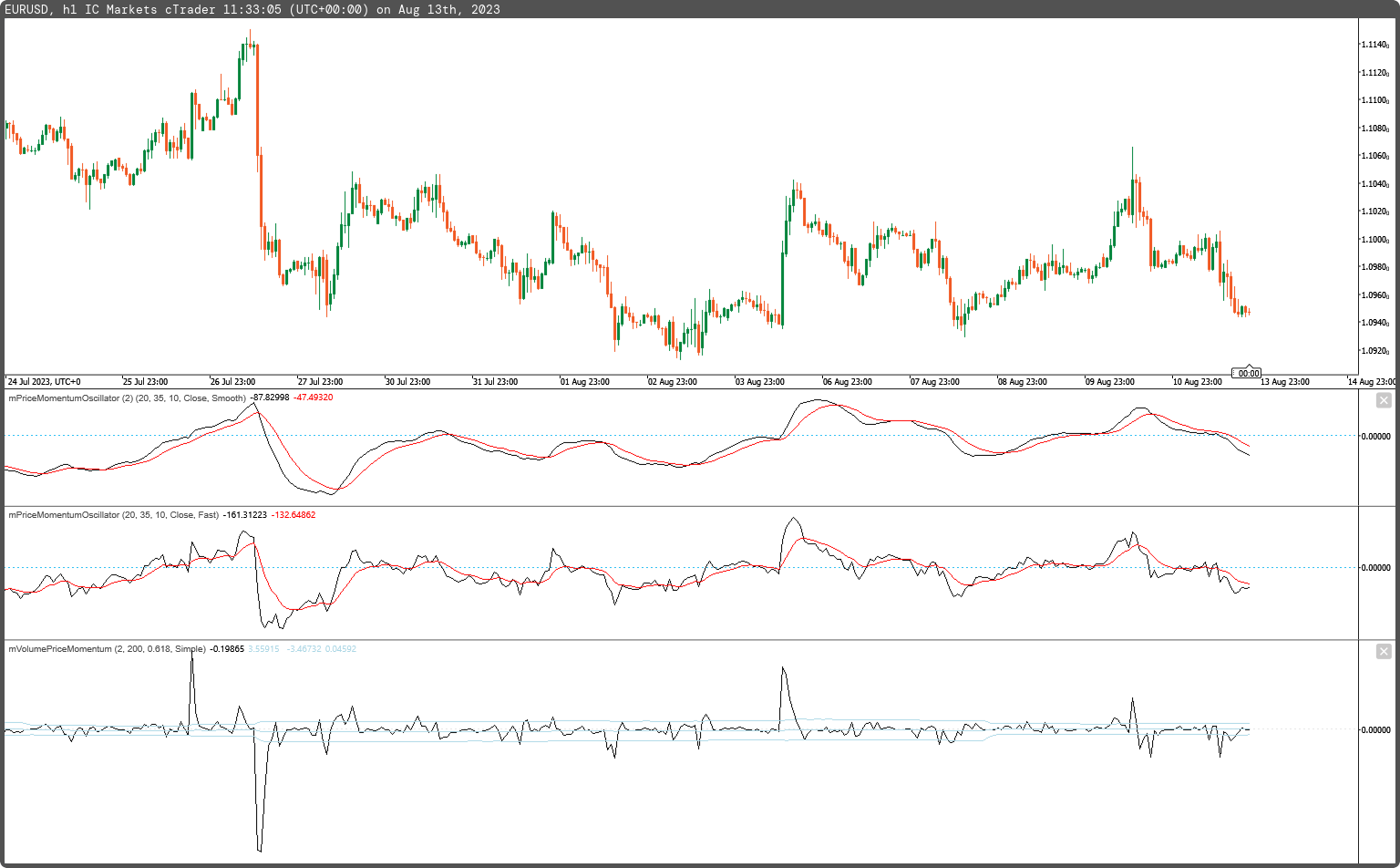
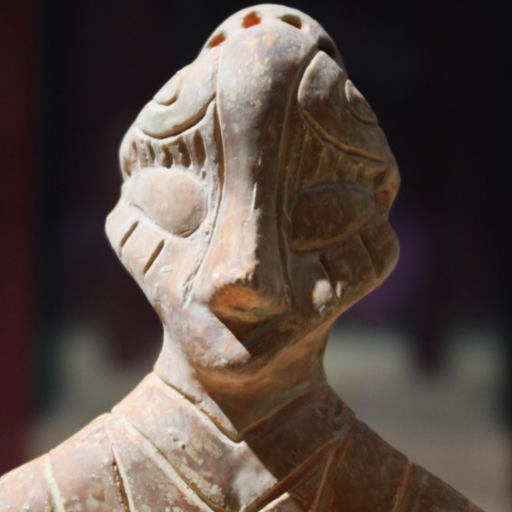
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mComparePriceMomentumOscillator.algo
- Rating: 5
- Installs: 471
- Modified: 13/08/2023 11:30
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
MF
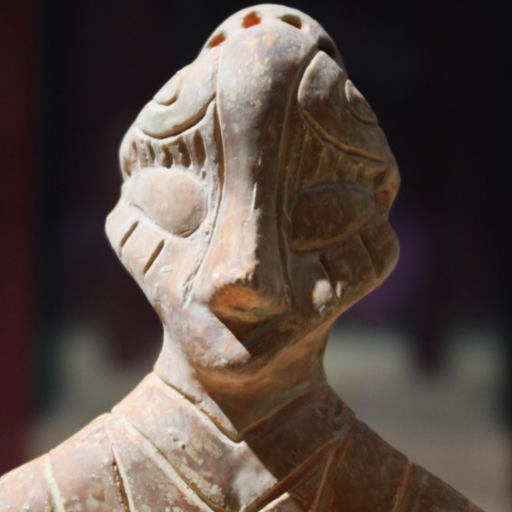
mfejza
·
1 year ago