An update for this algorithm is currently pending moderation. Please revisit this page shortly to access the algorithm's latest version.
Description
Normalized Volume is an indicator showing the normalized volume value.
It displays the average volume within the bars range, in the form of a colored histogram with a threshold value.
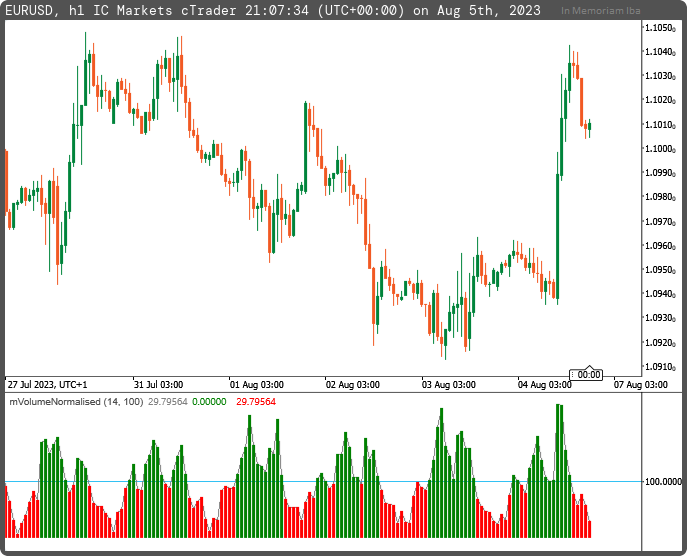
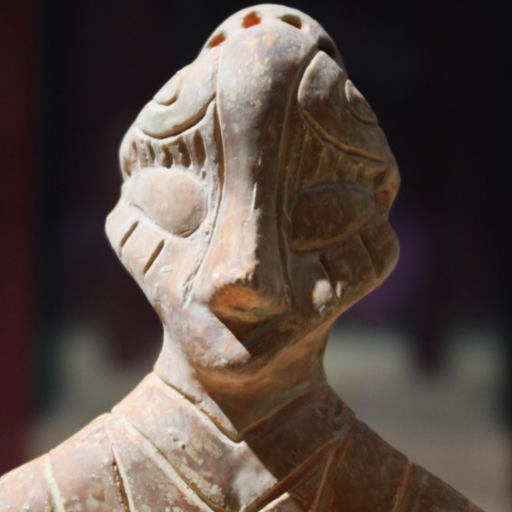
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mVolumeNormalised.algo
- Rating: 5
- Installs: 865
- Modified: 05/08/2023 21:09
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
MF
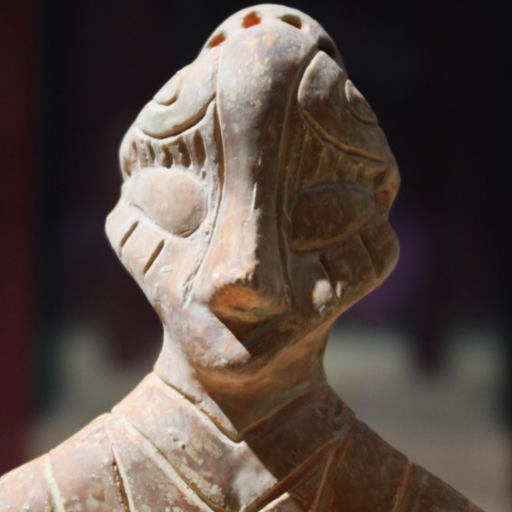
mfejza
·
1 year ago