Category Oscilators Published on 02/08/2023
Price Polarity of Positive&Negative Volume Index
An update for this algorithm is currently pending moderation. Please revisit this page shortly to access the algorithm's latest version.
Description
"Price Polarity of Positive & Negative Volume Index" is a custom indicator. In general, this indicator smooths price polarity for bullish and bearish sentiment, using the positive volume with price confluence into negative volume size.
In other words, when the indicator value is above the zero level, the bar sentiment is bullish. Likewise, when the indicator value is below the zero level, the bar sentiment is bearish.
In another way, this custom indicator is a synthetic price smoothing method using volume.
This indicator is developed based on my mentor Iba's documentation.
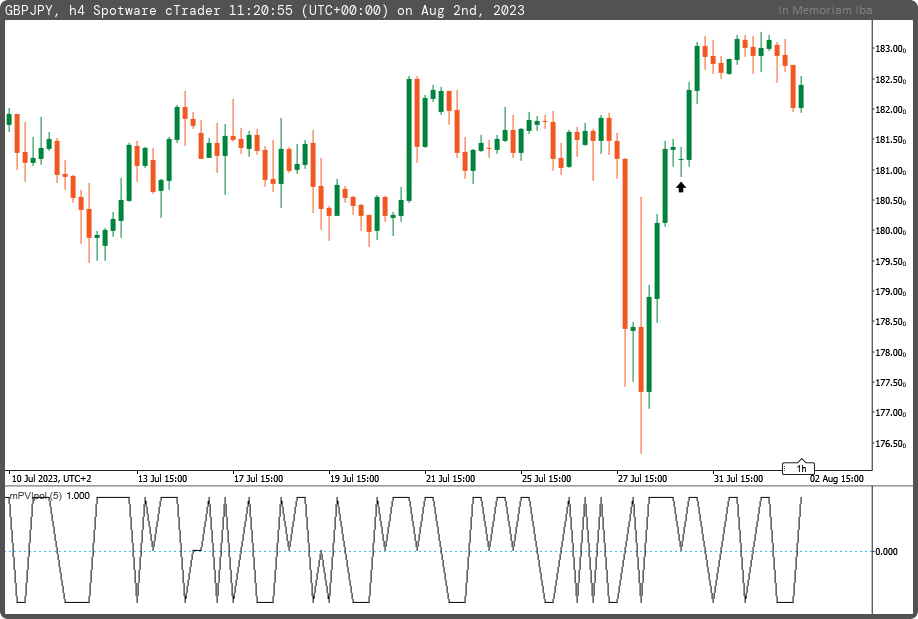
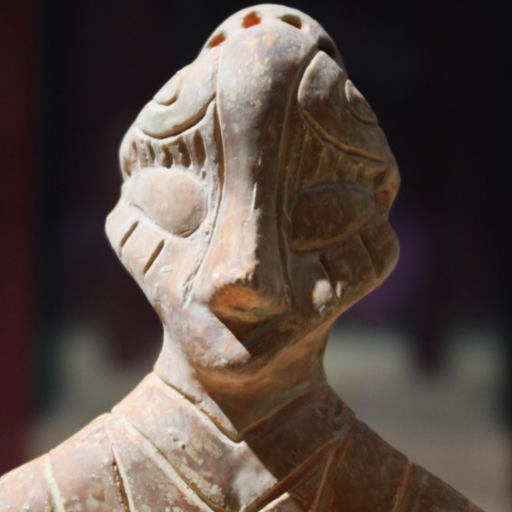
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mPNVIpol.algo
- Rating: 5
- Installs: 511
- Modified: 02/08/2023 11:27
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
MF
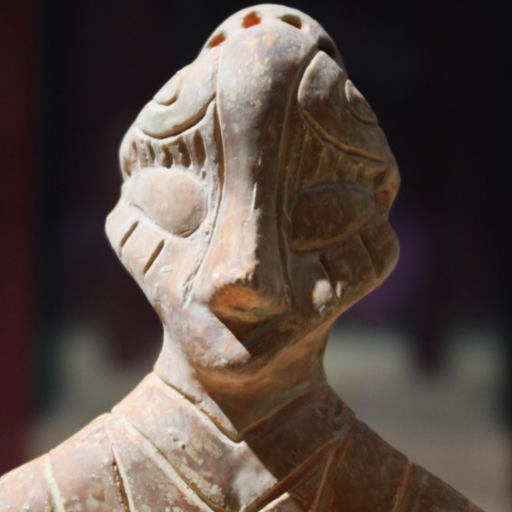
mfejza
·
1 year ago