Description
The Finite Impulse Response Filter (FIR) indicator is a symmetrically weighted filter.
The indicator calculates the result for fast components by smoothing the price using FIR and displays the difference between FIR and FastFIR. Similarly, the slow components are calculated by smoothing FastFIR using FIR and displaying the difference between FastFIR and SlowFIR.
In principle, the indicator shows the smoothing differences using the FIR filter.
In this version, the indicator shows price sentiment: bullish sentiment when both indicator components are above zero, and bearish sentiment when both indicator components are below zero.
For more information about FIR indicator click here
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Indicator(AccessRights = AccessRights.None)]
public class mFIRmomentum : Indicator
{
[Parameter("Data Source (close)", DefaultValue = enumPriceTypes.Close)]
public enumPriceTypes inpPriceType { get; set; }
[Output("FIR Momentum Fast", LineColor = "Black", PlotType = PlotType.Line, Thickness = 1)]
public IndicatorDataSeries outFast { get; set; }
[Output("FIR Momentum Slow", LineColor = "Red", PlotType = PlotType.Line, Thickness = 1)]
public IndicatorDataSeries outSlow { get; set; }
private IndicatorDataSeries _price, _fir0, _fir1, _fir2;
protected override void Initialize()
{
_price = CreateDataSeries();
_fir0 = CreateDataSeries();
_fir1 = CreateDataSeries();
_fir2 = CreateDataSeries();
}
public override void Calculate(int i)
{
switch(inpPriceType)
{
case enumPriceTypes.Open:
_price[i] = Bars.OpenPrices[i];
break;
case enumPriceTypes.Close:
_price[i] = Bars.ClosePrices[i];
break;
case enumPriceTypes.High:
_price[i] = Bars.HighPrices[i];
break;
case enumPriceTypes.Low:
_price[i] = Bars.LowPrices[i];
break;
case enumPriceTypes.Median:
_price[i] = Bars.MedianPrices[i];
break;
case enumPriceTypes.Typical:
_price[i] = Bars.TypicalPrices[i];
break;
case enumPriceTypes.Weighted:
_price[i] = Bars.WeightedPrices[i];
break;
default:
_price[i] = Bars.ClosePrices[i];
break;
}
_fir0[i] = i>3 ? (_price[i] + 2.0 * _price[i-1] + 2.0 * _price[i-2] + _price[i-3]) / 6.0 : Bars.ClosePrices[i];
_fir1[i] = i>3 ? (_fir0[i] + 2.0 * _fir0[i-1] + 2.0 * _fir0[i-2] + _fir0[i-3]) / 6.0 : Bars.ClosePrices[i];
_fir2[i] = i>3 ? (_fir1[i] + 2.0 * _fir1[i-1] + 2.0 * _fir1[i-2] + _fir1[i-3]) / 6.0 : Bars.ClosePrices[i];
outFast[i] = _fir0[i] - _fir2[i];
outSlow[i] = _fir1[i] - _fir2[i];
}
}
public enum enumPriceTypes
{
Open,
Close,
High,
Low,
Median,
Typical,
Weighted
}
}
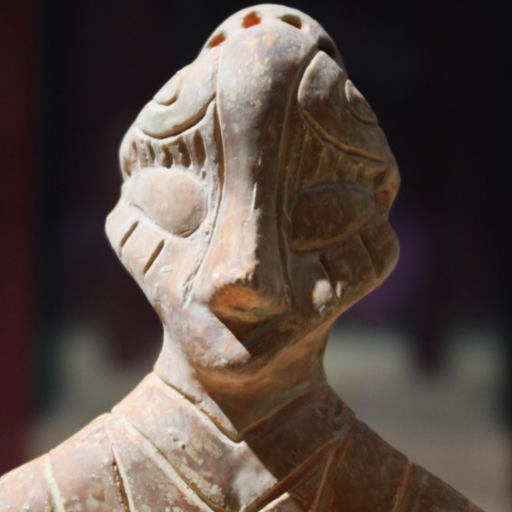
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mFIRmomentum.algo
- Rating: 5
- Installs: 421
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.