Description
Before beginning:
This project is an experimental project aimed at implementing a neural network cBot by using the Relative Vigor Index indicator as the input feed data generator for the neural network.
This cBot is not recommended for real trading, and before using it, you need to optimize the weights and input parameters for a particular financial instrument and trade timeframe. After optimization, the cBot can trade based on the optimization results, but many of us are not satisfied with trading blindly without knowing the type, level, and quality of trading opportunities.
As an additional part of this project, a custom indicator has been developed to visualize the results and fulfill the request of manual traders to verify the trading step before deciding to trade. This custom indicator has the same weighted input parameters as this cBot and should be the same (optimized by the cBot).
You can find the NeuroRVI visualization custom indicator from the provided link.
To compile and utilize this cBot, you will need to acquire the RVI indicator from the provided link.
The goal of this experimental project is to visualize the neural network trading system in a custom indicator.
Before use:
First of all, optimize the weights and input parameters for the cBot for a particular financial instrument and trade timeframe.
To use the NeuroRVI indicator for visualization, copy and paste the same optimized weights and input parameters calculated from the cBot for a particular financial instrument and trade timeframe.
In this link you have a sample procedure how to optimise a cBot.
Introduction:
Nowadays, every trader must have heard of neural networks and knows how cool it is to use them in trading.
In this article, I will try to explain the neural network architecture, describe its applications, and provide examples of practical use.
The Concept of Neural Networks:
Artificial neural networks are one of the areas in artificial intelligence research that is based on attempts to simulate the human nervous system in its ability to learn and adapt. This allows us to build a rough simulation of the human brain's operation.
Curiously enough, artificial neural networks are made up of artificial neurons.
The structure of a neuron can be represented as a composition of the following units: Inputs, Weights, Transfer Function, Activation Function, and Output.
The inputs unit represents the array data to be analyzed for further results. The weights unit represents the optimized array data for weighting the array data. The transfer function unit includes the method and normalization of array data for further processing. The Activation Function represents a multiplying weight value of data and weighted array by multiplying it with a coefficient value, implemented through a normalization formula (sigmoid, hyperbolic tangent, etc.). The Output is the result that contains the output value.
Neural networks have many properties, with the ability to learn being the most significant one. The learning process comes down to changing the weights.
Conclusion:
This article has covered the main points you need to know when designing a cBot using neural networks. It has shown us the structure of a neuron and neural network architecture, outlined the activation functions and the methods for changing the activation function shape, as well as the process of optimization and input data normalization. Furthermore, we have compared a cBot based on the standard logic with a neural network-driven cBot and visualized the trade system through a custom indicator.
Request:
I kindly request you to provide optimized weight data for a specific financial instrument in the comments section below, including information about the optimized time period, among other relevant details.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(AccessRights = AccessRights.None)]
public class mNeuroRVI_bot : Robot
{
[Parameter("RVI Period (10)", DefaultValue = 10)]
public int inpPeriodsRVI { get; set; }
[Parameter("Weight 0", DefaultValue = 1.0)]
public double inpWeight0 { get; set; }
[Parameter("Weight 1", DefaultValue = 0.1)]
public double inpWeight1 { get; set; }
[Parameter("Weight 2", DefaultValue = -0.8)]
public double inpWeight2 { get; set; }
[Parameter("Weight 3", DefaultValue = -0.5)]
public double inpWeight3 { get; set; }
[Parameter("Weight 4", DefaultValue = -0.7)]
public double inpWeight4 { get; set; }
[Parameter("Weight 5", DefaultValue = -0.4)]
public double inpWeight5 { get; set; }
[Parameter("Weight 6", DefaultValue = -0.6)]
public double inpWeight6 { get; set; }
[Parameter("Weight 7", DefaultValue = -0.4)]
public double inpWeight7 { get; set; }
[Parameter("Weight 8", DefaultValue = 0.9)]
public double inpWeight8 { get; set; }
[Parameter("Weight 9", DefaultValue = 0.5)]
public double inpWeight9 { get; set; }
[Parameter("Weight 10", DefaultValue = 1.0)]
public double inpWeight10 { get; set; }
[Parameter("Weight 11", DefaultValue = 0.1)]
public double inpWeight11 { get; set; }
[Parameter("Weight 12", DefaultValue = -0.8)]
public double inpWeight12 { get; set; }
[Parameter("Weight 13", DefaultValue = -0.5)]
public double inpWeight13 { get; set; }
[Parameter("Weight 14", DefaultValue = -0.7)]
public double inpWeight14 { get; set; }
[Parameter("Weight 15", DefaultValue = -0.4)]
public double inpWeight15 { get; set; }
[Parameter("Weight 16", DefaultValue = -0.6)]
public double inpWeight16 { get; set; }
[Parameter("Weight 17", DefaultValue = -0.4)]
public double inpWeight17 { get; set; }
[Parameter("Weight 18", DefaultValue = 0.9)]
public double inpWeight18 { get; set; }
[Parameter("Weight 19", DefaultValue = 0.5)]
public double inpWeight19 { get; set; }
[Parameter("Quantity (Lots)", Group = "Volume", DefaultValue = 0.01, MinValue = 0.01, Step = 0.01)]
public double Quantity { get; set; }
[Parameter("Stop Loss (pips)", Group = "Protection", DefaultValue = 30, MinValue = 1)]
public int StopLossInPips { get; set; }
[Parameter("Take Profit (pips)", Group = "Protection", DefaultValue = 60, MinValue = 1)]
public int TakeProfitInPips { get; set; }
private mRVI _rvi;
private strNeuroInputs[] _neuroinputs = new strNeuroInputs[20];
private double _d1, _d2, _rvimin, _rvimax, _outneuron;
protected override void OnStart()
{
_neuroinputs[0].weight = inpWeight0;
_neuroinputs[1].weight = inpWeight1;
_neuroinputs[2].weight = inpWeight2;
_neuroinputs[3].weight = inpWeight3;
_neuroinputs[4].weight = inpWeight4;
_neuroinputs[5].weight = inpWeight5;
_neuroinputs[6].weight = inpWeight6;
_neuroinputs[7].weight = inpWeight7;
_neuroinputs[8].weight = inpWeight8;
_neuroinputs[9].weight = inpWeight9;
_neuroinputs[10].weight = inpWeight10;
_neuroinputs[11].weight = inpWeight11;
_neuroinputs[12].weight = inpWeight12;
_neuroinputs[13].weight = inpWeight13;
_neuroinputs[14].weight = inpWeight14;
_neuroinputs[15].weight = inpWeight15;
_neuroinputs[16].weight = inpWeight16;
_neuroinputs[17].weight = inpWeight17;
_neuroinputs[18].weight = inpWeight18;
_neuroinputs[19].weight = inpWeight19;
_rvi = Indicators.GetIndicator<mRVI>(inpPeriodsRVI);
}
protected override void OnBar()
{
_d1 = -1.0; //lower limit of the normalization range
_d2 = +1.0; //upper limit of the normalization range
_rvimin = Math.Min(_rvi.outRVIMain.Minimum(20), _rvi.outRVISignal.Minimum(20));
_rvimax = Math.Max(_rvi.outRVIMain.Maximum(20), _rvi.outRVISignal.Maximum(20));
if (_rvi.outRVIMain.Count < 10)
return;
for (int j = 0; j < 20 / 2; j++)
{
_neuroinputs[j * 2].inputs = (((_rvi.outRVIMain.Last(j) - _rvimin) * (_d2 - _d1)) / (_rvimax - _rvimin)) + _d1;
_neuroinputs[j * 2 + 1].inputs = (((_rvi.outRVISignal.Last(j) - _rvimin) * (_d2 - _d1)) / (_rvimax - _rvimin)) + _d1;
}
_outneuron = CalculateNeuron(); //neural network logic
bool _rvibullishlogic = _rvi.outRVIMain.LastValue > 0 && _rvi.outRVIMain.LastValue > _rvi.outRVISignal.LastValue;
bool _rvibearishlogic = _rvi.outRVIMain.LastValue < 0 && _rvi.outRVIMain.LastValue < _rvi.outRVISignal.LastValue;
if (_outneuron < -0)
//if(_outneuron < -0 && _rvibullishlogic)
{
//buy
var position = Positions.Find("neurorvi", Symbol.Name, TradeType.Buy);
if (position == null)
ExecuteMarketOrder(TradeType.Buy, SymbolName, VolumeInUnits, "neurorvi", StopLossInPips, TakeProfitInPips);
}
if (_outneuron >= +0)
//if(_outneuron > -0 && _rvibearishlogic)
{
//sell
var position = Positions.Find("neurorvi", Symbol.Name, TradeType.Sell);
if (position == null)
ExecuteMarketOrder(TradeType.Sell, SymbolName, VolumeInUnits, "neurorvi", StopLossInPips, TakeProfitInPips);
}
}
protected override void OnTick()
{
//
}
protected override void OnError(Error error)
{
//
}
protected override void OnStop()
{
//
}
private double CalculateNeuron()
{
double neuron = 0.0;
for (int n = 0; n < 20; n++) //--- Using a loop we obtain the weighted sum of inputs based on the number of inputs
{
neuron += _neuroinputs[n].inputs * _neuroinputs[n].weight;
}
neuron *= 0.1; //--- multiply the weighted sum of inputs by the additional coefficient
return (ActivateNeuron(neuron));
}
private double ActivateNeuron(double x)
{
return (Math.Exp(x) - Math.Exp(-x)) / (Math.Exp(x) + Math.Exp(-x)); //--- return the activation function value
}
private struct strNeuroInputs
{
public double weight;
public double inputs;
}
private double VolumeInUnits
{
get { return Symbol.QuantityToVolumeInUnits(Quantity); }
}
}
}
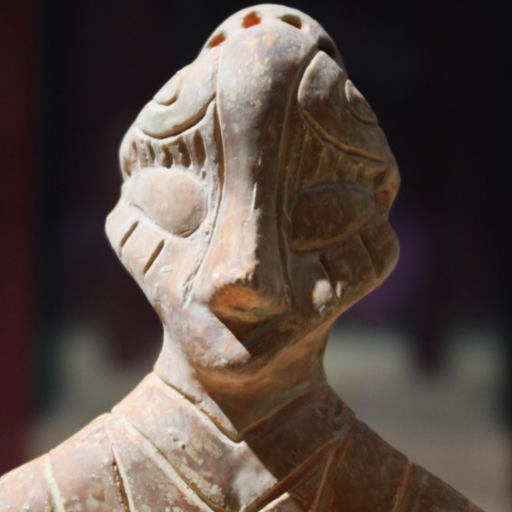
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mNeuroRVI_bot.algo
- Rating: 5
- Installs: 840
- Modified: 03/07/2023 11:53
Comments
Mate thanks heaps for all your indicator now and I missed this! Actually I would never want to run NNs on financial data, NNs are really only good for well established consistent phenomena, although what they are doing with LLMs is amazing but with LLMs you have the advantage of variable interpretation as is the nature of language so they have more data to work with but also as you know they hallucinate. I have built a generic ML framework based on ML.NET and applied it to trading, I would love to show you. Traders can select features and train and test models themselves without any need for a data scientist. Also their features remain proprietary to them. acrigney at gmail com. I can send you my paper?
não consegue criar um cbot para o indicador seu mATRLevelStopReverse.algo ?