Description
The Adaptive Price Zone (APZ) represents an adaptive price channel. The indicator was described in the article 'Trading With An Adaptive Price Zone' by Lee Leibfarth in 'Stocks and Commodities' in September 2006.
This indicator is sensitive to price, and such sensitivity produces information about volatility by expanding the channel components. However, there is a problem when feeding the indicator with input data only from bar information.
In this version, as an input filter, you can smooth the price by applying the FIR filter for more stable output values.
An unwritten strategy for trading with this indicator is to apply three indicators and trade by respecting the order of channel components. For long trades, the faster up component must be above the medium up component, and the medium up component must be above the slow up component. For short trades, the faster down component must be below the medium down component, and the medium down component must be below the slow down component. Otherwise, the strategy information is considered as a price range. See Fig. 2.
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Indicator(IsOverlay = true, AccessRights = AccessRights.None)]
public class mAdaptivePriceZone : Indicator
{
[Parameter("Period (20)", DefaultValue = 20, MinValue = 2)]
public int inpPeriod { get; set; }
[Parameter("Width (0.02)", DefaultValue = 2)]
public double inpWidth { get; set; }
[Parameter("Smooth Type (sma)", DefaultValue = MovingAverageType.Simple)]
public MovingAverageType inpSmoothType { get; set; }
[Parameter("Use FIR Smooth (no)", DefaultValue = false)]
public bool inpUseFIRsmooth { get; set; }
[Parameter("Use Mid Zone (no)", DefaultValue = false)]
public bool inpShowMidZone { get; set; }
[Output("AdaptivePriceZoneUp", LineColor = "Red", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outAdaptivePriceZoneUp { get; set; }
[Output("AdaptivePriceZoneDn", LineColor = "Green", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outAdaptivePriceZoneDn { get; set; }
[Output("AdaptivePriceZoneMid", LineColor = "Gray", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outAdaptivePriceZoneMid { get; set; }
private IndicatorDataSeries _range, _price, _apzup, _apzdn;
private MovingAverage _rangesmooth, _pricesmooth;
protected override void Initialize()
{
_range = CreateDataSeries();
_price = CreateDataSeries();
_apzup = CreateDataSeries();
_apzdn = CreateDataSeries();
_rangesmooth = Indicators.MovingAverage(_range, inpPeriod, inpSmoothType);
_pricesmooth = Indicators.MovingAverage(_price, inpPeriod, inpSmoothType);
}
public override void Calculate(int i)
{
_range[i] = i>3 ? ((Bars.HighPrices[i] + 2.0 * Bars.HighPrices[i-1] + 2.0 * Bars.HighPrices[i-2] + Bars.HighPrices[i-3]) / 6.0)
- ((Bars.LowPrices[i] + 2.0 * Bars.LowPrices[i-1] + 2.0 * Bars.LowPrices[i-2] + Bars.LowPrices[i-3]) / 6.0)
: Bars.HighPrices[i] - Bars.LowPrices[i];
_price[i] = i>3 && inpUseFIRsmooth == true ? (Bars.ClosePrices[i] + 2.0 * Bars.ClosePrices[i-1] + 2.0 * Bars.ClosePrices[i-2] + Bars.ClosePrices[i-3]) / 6.0 : Bars.ClosePrices[i];
_apzup[i] = _pricesmooth.Result[i] + inpWidth * _rangesmooth.Result[i];
_apzdn[i] = _pricesmooth.Result[i] - inpWidth * _rangesmooth.Result[i];
outAdaptivePriceZoneUp[i] = _apzup[i];
outAdaptivePriceZoneDn[i] = _apzdn[i];
outAdaptivePriceZoneMid[i] = inpShowMidZone == true ? (_apzup[i] + _apzdn[i]) /2 : double.NaN;
}
}
}
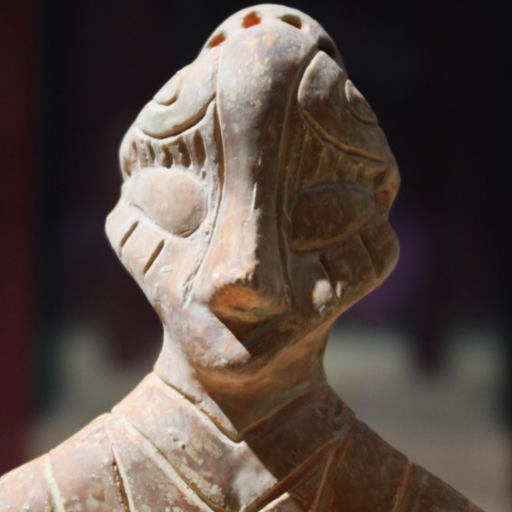
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mAdaptivePriceZone.algo
- Rating: 5
- Installs: 426