Description
The custom indicator 'William's Accumulation Distribution Starc' displays the accumulation distribution component (black) and utilizes this component to create bands for use as a filter to identify momentum pressure (black component outside the top and bottom bands).
For long-term traders, the smooth moving average component (dodger blue) of the accumulation distribution component is used as a trend filter. When the accumulation distribution component is above the top band, trade only long, and vice versa.
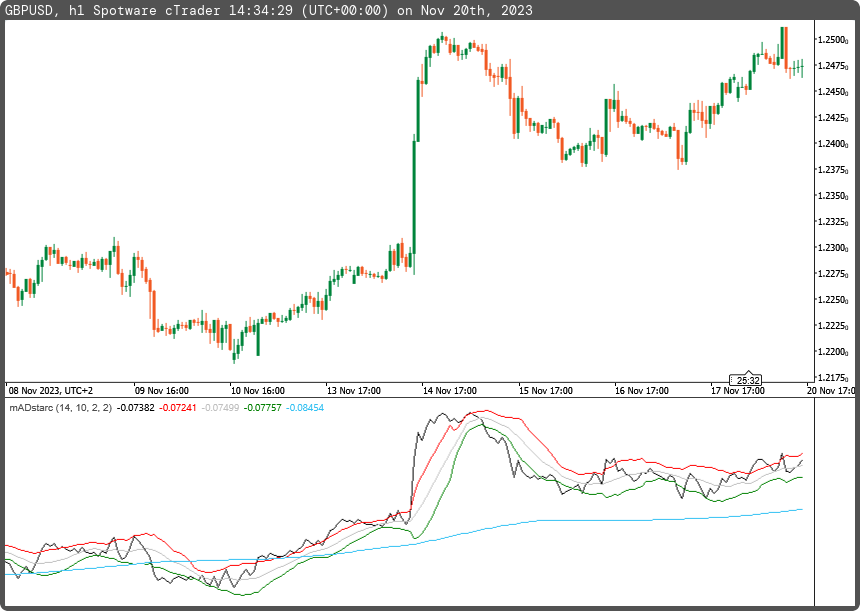
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Cloud("Filter", "Level100", FirstColor = "Orange", SecondColor = "Orange", Opacity = 0.1)]
[Levels(-100)]
[Indicator(AccessRights = AccessRights.None)]
public class mADstarc : Indicator
{
[Parameter("Smooth Periods (14)", DefaultValue = 14)]
public int inpPeriodsSmooth { get; set; }
[Parameter("ATR Periods (10)", DefaultValue = 10)]
public int inpPeriodsATR { get; set; }
[Parameter("Multiplier Top (2.0)", DefaultValue = 2.0)]
public double inpMultiplierTop { get; set; }
[Parameter("Multiplier Bottom (2.0)", DefaultValue = 2.0)]
public double inpMultiplierBottom { get; set; }
[Output("William's Accumulation Distribution Starc", LineColor = "Black", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outDPO { get; set; }
[Output("Top", LineColor = "Red", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outTop { get; set; }
[Output("Middle", LineColor = "Silver", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outMid { get; set; }
[Output("Bottom", LineColor = "Green", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outBottom { get; set; }
[Output("Filter", LineColor = "FF33C1F3", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outFilter200 { get; set; }
[Output("Level100", LineColor = "Transparent", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outLevel100 { get; set; }
private WilliamsAccumulationDistribution _ad;
private MovingAverage _center, _atrma, _sma200;
private IndicatorDataSeries _tr, _top, _bottom;
protected override void Initialize()
{
_ad = Indicators.WilliamsAccumulationDistribution();
_center = Indicators.MovingAverage(_ad.Result, inpPeriodsSmooth, MovingAverageType.Simple);
_tr = CreateDataSeries();
_atrma = Indicators.MovingAverage(_tr, inpPeriodsATR, MovingAverageType.Simple);
_top = CreateDataSeries();
_bottom = CreateDataSeries();
_sma200 = Indicators.MovingAverage(_ad.Result, 200, MovingAverageType.Simple);
}
public override void Calculate(int i)
{
_tr[i] = i>1 ? Math.Abs(_ad.Result[i] - _ad.Result[i-1]) : Math.Abs(_ad.Result[i] - 0.0);
_top[i] = _center.Result[i] + inpMultiplierTop * _atrma.Result[i];
_bottom[i] = _center.Result[i] - inpMultiplierBottom * _atrma.Result[i];
outDPO[i] = _ad.Result[i];
outTop[i] = _top[i];
outMid[i] = _center.Result[i];
outBottom[i] = _bottom[i];
outFilter200[i] = _sma200.Result[i];
}
}
}
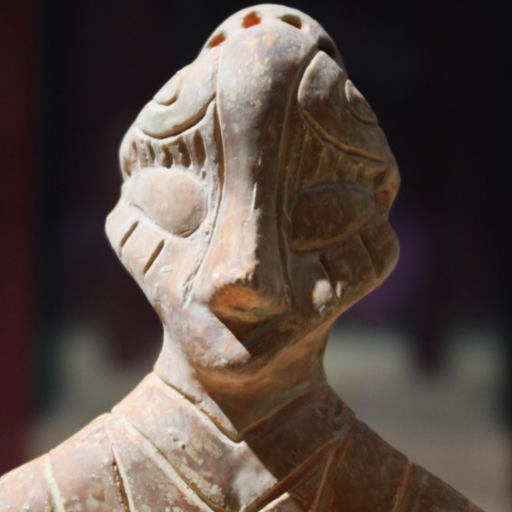
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mADstarc.algo
- Rating: 0
- Installs: 332
- Modified: 20/11/2023 14:35
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.