Description
Relative Moving Average It's like the EMA but it uses alpha = 1/length instead of 2/(length + 1) It gives a bit less weight (about half of EMA) to the most recent data point
If you've found this useful or have suggestions let me know.
You can support this and more tools I'm making via Ko-Fi
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Diagnostics;
using System.Linq;
using System.Text;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using RelativeMovingAverage.Properties;
/*
This is the pineScript code for the Relative Moving Average
It's like the EMA but it uses alpha = 1/length instead of 2/(length + 1)
It gives a bit less weight (about half of EMA) to the most recent data point
//https://www.tradingview.com/pine-script-reference/v5/#fun_ta.rma
//@version=5
indicator("ta.rma")
plot(ta.rma(close, 15))
//the same on pine
pine_rma(src, length) =>
alpha = 1/length
sum = 0.0
sum := na(sum[1]) ? ta.sma(src, length) : alpha * src + (1 - alpha) * nz(sum[1])
plot(pine_rma(close, 15))
*/
//You can support/contact me via Ko-Fi
//https://ko-fi.com/waxycodes
namespace cAlgo;
[Indicator(AccessRights = AccessRights.FullAccess, IsOverlay = true)]
public class RelativeMovingAverage : Indicator
{
[Parameter("Source", DefaultValue = "Close")]
public DataSeries InputSource { get; set; }
[Parameter("Length", DefaultValue = 15)]
public int InputLength { get; set; }
[Output("Main", LineColor = "FF00FFFF", Thickness = 2)]
public IndicatorDataSeries Result { get; set; }
private double _alpha;
private SimpleMovingAverage _smaSrc;
protected override void Initialize()
{
_alpha = 1.0 / InputLength;
_smaSrc = Indicators.SimpleMovingAverage(InputSource, InputLength);
//Remove if needed
//Support these and more tools for trading at
//https://ko-fi.com/waxycodes
//Thanks
SetKofi();
}
public override void Calculate(int index)
{
Result[index] = double.IsNaN(Result[index - 1]) ? _smaSrc.Result[index] : _alpha * InputSource[index] + (1 - _alpha) * Nz(Result[index - 1]);
}
public double Nz(double value) => double.IsNaN(value) ? 0 : value;
public DataSeries Open => Bars.OpenPrices;
public DataSeries High => Bars.HighPrices;
public DataSeries Low => Bars.LowPrices;
public DataSeries Close => Bars.ClosePrices;
public TimeSeries Times => Bars.OpenTimes;
public int Index => Bars.Count - 1;
#region KoFi
private TextBlock _textBlock;
private Button _button;
private StackPanel _stack;
private int _counter;
private void SetKofi()
{
var image = new Image
{
// Logo is an icon file inside project resources
Source = Resources.my_kofi_support,
Width = 200,
//Height = 200,
};
_textBlock = new TextBlock
{
Text = ClickTheImageBelow(10),
FontSize = 12,
FontWeight = FontWeight.Bold,
ForegroundColor = Chart.ColorSettings.ForegroundColor,
HorizontalAlignment = HorizontalAlignment.Center,
VerticalAlignment = VerticalAlignment.Center,
Margin = 1,
Padding = 1,
Width = 198,
};
_button = new Button
{
Content = image,
BackgroundColor = Color.Transparent,
Margin = 2,
Padding = 0,
CornerRadius = 0
};
_stack = new StackPanel
{
HorizontalAlignment = HorizontalAlignment.Right,
VerticalAlignment = VerticalAlignment.Bottom,
};
_stack.AddChild(_textBlock);
_stack.AddChild(_button);
_button.Click += Button_Click;
Chart.AddControl(_stack);
Timer.Start(1);
}
private void Button_Click(ButtonClickEventArgs obj)
{
Process.Start(new ProcessStartInfo
{
FileName = "https://ko-fi.com/waxycodes",
UseShellExecute = true
});
}
protected override void OnTimer()
{
_counter++;
_textBlock.Text = ClickTheImageBelow(10 - _counter);
if (_counter != 10)
return;
Chart.RemoveControl(_stack);
Timer.Stop();
}
private string ClickTheImageBelow(int seconds) => $"Click the Link Below... ({seconds})";
#endregion
}
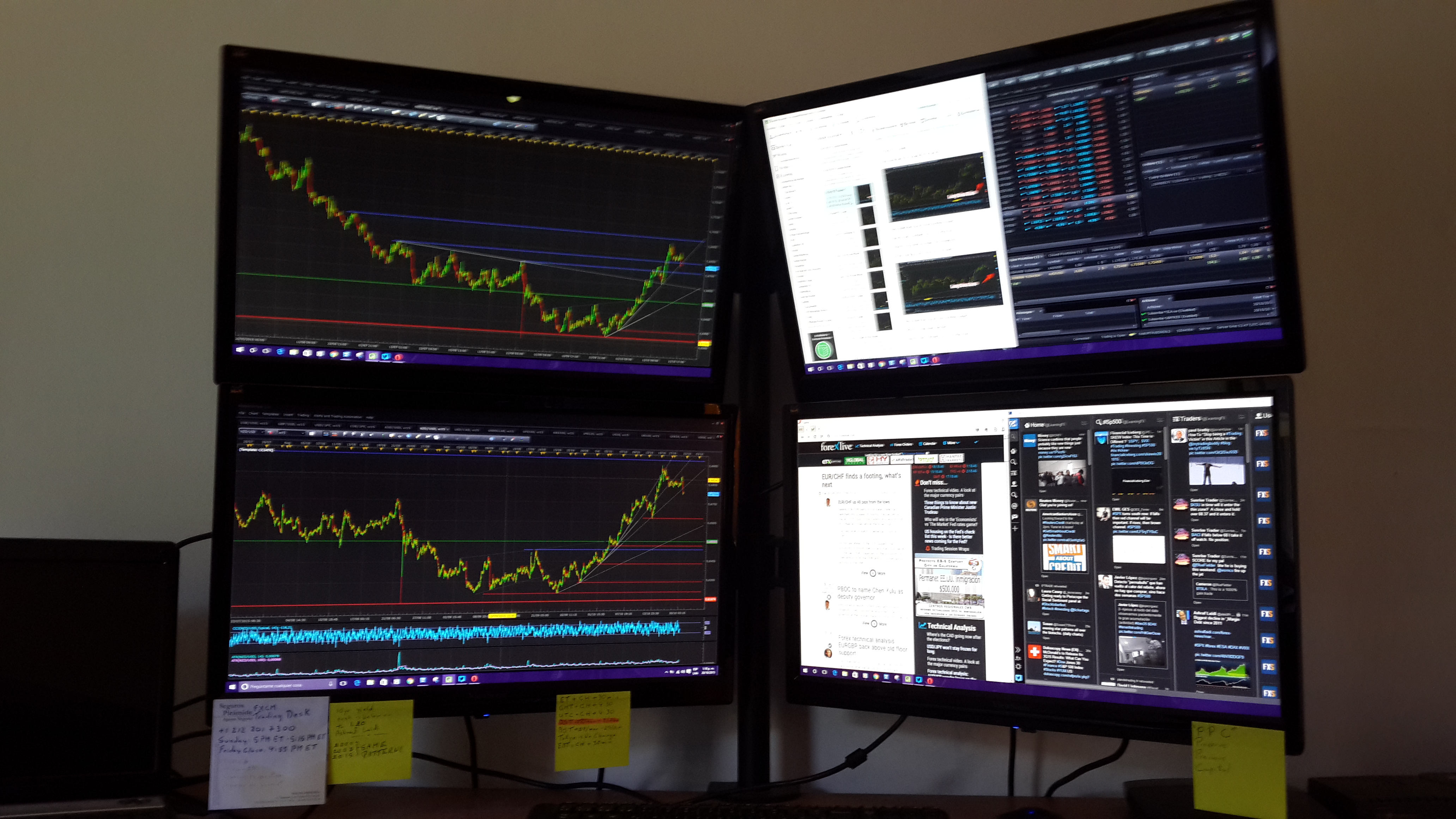
Waxy
Joined on 12.05.2015
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: RelativeMovingAverage.algo
- Rating: 0
- Installs: 555
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.