Description
The Cyclic Component Oscillator is a study designed by John Ehlers. It is used to isolate the cycle component of the market from its trend counterpart. The wave of the Cyclic Component Oscillator has a variable amplitude.
The basic rule for utilizing this study is to use crossovers with the plot shifted back as buy/sell signals. However, it is recommended to benefit from applying the Inverse Fisher Transform to the oscillator.
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Levels(0)]
[Indicator(IsOverlay = false, AccessRights = AccessRights.None)]
public class mCyclicComponentOscillator : Indicator
{
[Parameter("Period (20)", DefaultValue = 20, MinValue = 2)]
public int inpPeriod { get; set; }
[Parameter("Data Source (close)", DefaultValue = enumPriceTypes.Close)]
public enumPriceTypes inpPriceType { get; set; }
[Output("Cyclic Component Oscillator", LineColor = "Black", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outCyclicComponent { get; set; }
private double _alpha;
private IndicatorDataSeries _price, _hp, _cycliccomponent;
protected override void Initialize()
{
_alpha = (1.0 - Math.Sin(2.0 * Math.PI / inpPeriod)) / Math.Cos(2.0 * Math.PI / inpPeriod);
_price = CreateDataSeries();
_hp = CreateDataSeries();
_cycliccomponent = CreateDataSeries();
}
public override void Calculate(int i)
{
switch(inpPriceType)
{
case enumPriceTypes.Open:
_price[i] = Bars.OpenPrices[i];
break;
case enumPriceTypes.Close:
_price[i] = Bars.ClosePrices[i];
break;
case enumPriceTypes.High:
_price[i] = Bars.HighPrices[i];
break;
case enumPriceTypes.Low:
_price[i] = Bars.LowPrices[i];
break;
case enumPriceTypes.Median:
_price[i] = Bars.MedianPrices[i];
break;
case enumPriceTypes.Typical:
_price[i] = Bars.TypicalPrices[i];
break;
case enumPriceTypes.Weighted:
_price[i] = Bars.WeightedPrices[i];
break;
default:
_price[i] = Bars.ClosePrices[i];
break;
}
_hp[i] = i>1 ? 0.5 * (1.0 + _alpha) * (Bars.ClosePrices[i] - Bars.ClosePrices[i-1]) + _alpha * _hp[i-1] : 0.5 * (1.0 + _alpha) * (Bars.ClosePrices[i] - Bars.TypicalPrices[i]);
_cycliccomponent[i] = i>3 ? (_hp[i] + 2.0 * _hp[i-1] + 2.0 * _hp[i-2] + _hp[i-3]) / 6.0 : _hp[i];
outCyclicComponent[i] = _cycliccomponent[i];
}
}
public enum enumPriceTypes
{
Open,
Close,
High,
Low,
Median,
Typical,
Weighted
}
}
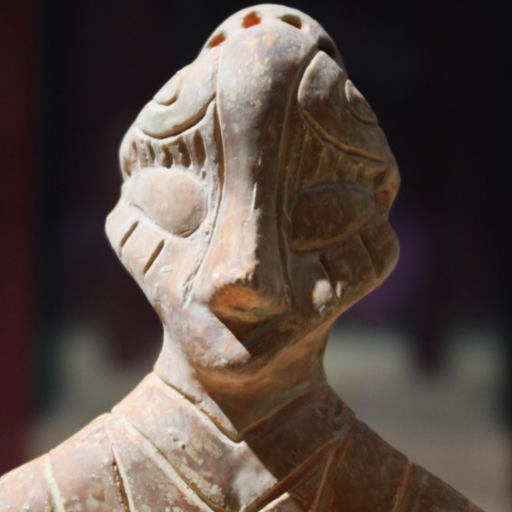
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mCyclicComponentOscillator.algo
- Rating: 5
- Installs: 406
- Modified: 09/06/2023 07:27
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.