Description
The Percent Difference Oscillator (PDO) oscillator shows the difference between the smoothed price average and the current price, expressing the difference as a percentage of the moving average (above or below the smoothed price average).
It is used across different periods to consider the confluence factor. For example, periods of 10, 50, 100, and 200 are commonly used.
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Levels(0)]
[Cloud("Percent Difference Oscillator", "Zero Level", FirstColor = "Green", SecondColor = "Red", Opacity = 0.1)]
[Indicator(IsOverlay = false, AccessRights = AccessRights.None)]
public class mPercentDifferenceOscillator : Indicator
{
[Parameter("Period (13)", DefaultValue = 13, MinValue = 2)]
public int inpPeriod { get; set; }
[Parameter("Smooth Type (sma)", DefaultValue = MovingAverageType.Simple)]
public MovingAverageType inpSmoothType { get; set; }
[Parameter("Data Source (close)", DefaultValue = enumPriceTypes.Close)]
public enumPriceTypes inpPriceType { get; set; }
[Output("Percent Difference Oscillator", LineColor = "Black", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outPDO { get; set; }
[Output("Zero Level", LineColor = "Transparent", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outZeroLevel { get; set; }
private IndicatorDataSeries _price, _pdo;
private MovingAverage _smoothprice1, _smoothprice;
protected override void Initialize()
{
_price = CreateDataSeries();
_pdo = CreateDataSeries();
_smoothprice1 = Indicators.MovingAverage(_price, 1, MovingAverageType.Simple);
_smoothprice = Indicators.MovingAverage(_price, inpPeriod, inpSmoothType);
}
public override void Calculate(int i)
{
switch(inpPriceType)
{
case enumPriceTypes.Open:
_price[i] = Bars.OpenPrices[i];
break;
case enumPriceTypes.Close:
_price[i] = Bars.ClosePrices[i];
break;
case enumPriceTypes.High:
_price[i] = Bars.HighPrices[i];
break;
case enumPriceTypes.Low:
_price[i] = Bars.LowPrices[i];
break;
case enumPriceTypes.Median:
_price[i] = Bars.MedianPrices[i];
break;
case enumPriceTypes.Typical:
_price[i] = Bars.TypicalPrices[i];
break;
case enumPriceTypes.Weighted:
_price[i] = Bars.WeightedPrices[i];
break;
default:
_price[i] = Bars.ClosePrices[i];
break;
}
_pdo[i] = _smoothprice.Result[i] != 0 ? 100.0 * (_smoothprice1.Result[i] - _smoothprice.Result[i]) / _smoothprice.Result[i] : 0;
outPDO[i] = _pdo[i];
outZeroLevel[i] = 0.0;
}
}
public enum enumPriceTypes
{
Open,
Close,
High,
Low,
Median,
Typical,
Weighted
}
}
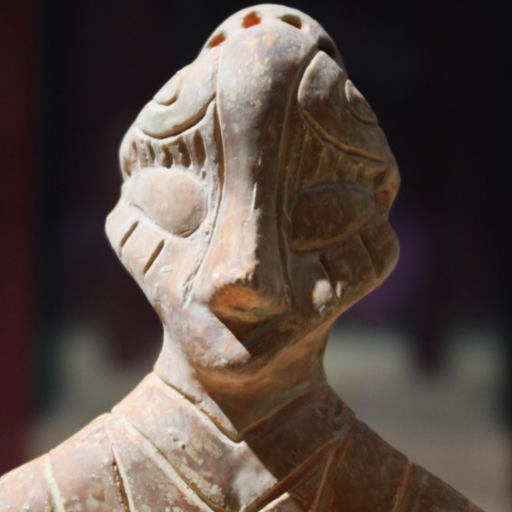
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mPercentDifferenceOscillator.algo
- Rating: 5
- Installs: 392
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.