Description
Forecast oscillator was developed by Tushar Chande and is the continuation of his "Time Frame Forecast" method.
Interpretation: Positive indicator values predict price growth, while negative values suggest the price may fall. Tushar Chande also recommends using a 3-day smoothing line as a signal line. When the oscillator line is accelerated relative to the signal line or crosses it, this indicates either a stopping of the price movement (reversal or intersection opposite to the signal line movement), or a continuation of movement in the same direction (reversal or intersection in the direction of the signal line movement), or a forthcoming trend change (which needs to be confirmed by the intersection of the oscillator's zero value). Data interpretation depends on the position of the oscillator line, i.e., whether it is above or below zero.
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Indicator(IsOverlay = false, AccessRights = AccessRights.None)]
public class mForecast : Indicator
{
[Parameter("Main Period (20)", DefaultValue = 20)]
public int inpPeriod { get; set; }
[Parameter("Data Source (close)", DefaultValue = enumPriceTypes.Close)]
public enumPriceTypes inpPriceType { get; set; }
[Output("Forecast oscillator", LineColor = "Black", PlotType = PlotType.Line, Thickness = 1)]
public IndicatorDataSeries outForecast { get; set; }
[Output("Forecast signal", LineColor = "Red", PlotType = PlotType.Line, Thickness = 1)]
public IndicatorDataSeries outSignal { get; set; }
private IndicatorDataSeries _price, _fosc, _signal;
private LinearRegressionForecast _lrf;
protected override void Initialize()
{
_price = CreateDataSeries();
_fosc = CreateDataSeries();
_signal = CreateDataSeries();
_lrf = Indicators.LinearRegressionForecast(_price, inpPeriod);
}
public override void Calculate(int i)
{
switch (inpPriceType)
{
case enumPriceTypes.Open:
_price[i] = Bars.OpenPrices[i];
break;
case enumPriceTypes.Close:
_price[i] = Bars.ClosePrices[i];
break;
case enumPriceTypes.High:
_price[i] = Bars.HighPrices[i];
break;
case enumPriceTypes.Low:
_price[i] = Bars.LowPrices[i];
break;
case enumPriceTypes.Median:
_price[i] = Bars.MedianPrices[i];
break;
case enumPriceTypes.Typical:
_price[i] = Bars.TypicalPrices[i];
break;
case enumPriceTypes.Weighted:
_price[i] = Bars.WeightedPrices[i];
break;
default:
_price[i] = Bars.ClosePrices[i];
break;
}
_fosc[i] = (_price[i] != 0 ? 100.0 * (_price[i] - _lrf.Result[i]) / _price[i] : 0);
_signal[i] = i> 3 ? (_fosc[i] + 2.0 * _fosc[i-1] + 2.0 * _fosc[i-2] + _fosc[i-3]) / 6.0 : _fosc[i];
outForecast[i] = _fosc[i];
outSignal[i] = _signal[i];
}
}
public enum enumPriceTypes
{
Open,
Close,
High,
Low,
Median,
Typical,
Weighted
}
}
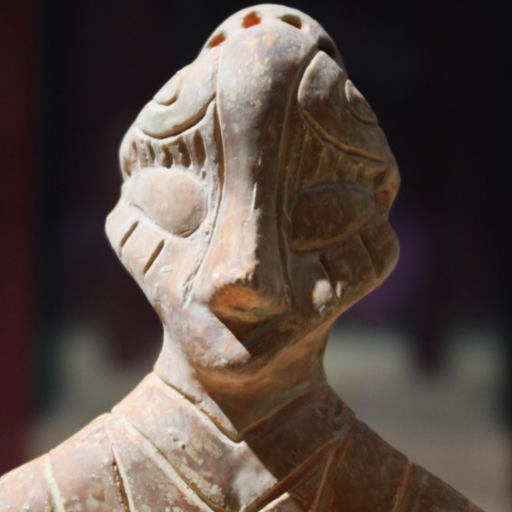
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mForecast.algo
- Rating: 5
- Installs: 602
- Modified: 08/05/2023 20:39
Comments
Youre so cool! I dont suppose Ive learn something like this before. So good to search out anyone with some original ideas on this subject. realy thank you for beginning this up. this web site is something that is wanted on the web, someone with just a little originality. helpful job for bringing one thing new to the web!how to become a credit card processor
power tools can really save you from a lot of headache, specially when the job is very hard-how to be a payment processor
You produced some decent points there. I looked on the web for that issue and found most people will go as well as with all your site.how to sell credit card processing
When Apple didn’t make computers as well as Local cafe did not help make coffee, how arrive acquired they will become thus prospering? The journey to offer the state-of-the-art and most interesting goods, even though intoxicating, is often a fairly packed location. If possible, Apple and Starbucks built their own niche.Packers and Movers Chennai to Dehradun
I like this post!