Description
The Custom VIX Oscillator is a combination of the Average True Range (ATR) and the Standard Deviation (STD), which are both measures of volatility. The oscillator is normalized to range between 0 and 100. The interpretation of this oscillator can be based on the following points:
-
Higher values indicate higher volatility: When the Custom VIX Oscillator is at high levels (closer to 100), it indicates that the market is experiencing higher volatility. This can be a sign of potential trend reversals or increased uncertainty in the market.
-
Lower values indicate lower volatility: When the Custom VIX Oscillator is at low levels (closer to 0), it signifies that the market is experiencing lower volatility. Lower volatility periods may indicate stable trends or consolidation in the market.
-
-
Overbought/Oversold conditions: As with other oscillators, you can interpret the Custom VIX Oscillator as indicating overbought or oversold conditions. When the oscillator reaches extreme values (close to 100 or 0), it may indicate that the market is reaching an extreme in volatility, and a reversal in the current trend could be imminent.
-
Divergences: You can look for divergences between the Custom VIX Oscillator and the price action. For example, if the price makes a new high, but the oscillator fails to make a new high, this could signal a potential bearish reversal. Similarly, if the price makes a new low, but the oscillator fails to make a new low, this could signal a potential bullish reversal.
For any questions, do not hesitate to contact me: singalgolab@gmail.com
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class CustomVIXOscillator : Indicator
{
[Parameter("Period", DefaultValue = 14)]
public int Period { get; set; }
[Output("Custom VIX Oscillator", LineColor = "Blue")]
public IndicatorDataSeries CustomVIXOscillatorSeries { get; set; }
private AverageTrueRange _atr;
private StandardDeviation _stdDev;
protected override void Initialize()
{
_atr = Indicators.AverageTrueRange(Period, MovingAverageType.Exponential);
_stdDev = Indicators.StandardDeviation(Bars.ClosePrices, Period, MovingAverageType.Simple);
}
public override void Calculate(int index)
{
double atrValue = _atr.Result[index];
double stdDevValue = _stdDev.Result[index];
double customVIX = (atrValue + stdDevValue) / 2;
double normalizedCustomVIX = Normalize(customVIX, index);
CustomVIXOscillatorSeries[index] = normalizedCustomVIX;
}
private double Normalize(double value, int index)
{
double maxValue = double.MinValue;
double minValue = double.MaxValue;
for (int i = index - Period + 1; i <= index; i++)
{
double atrValue = _atr.Result[i];
double stdDevValue = _stdDev.Result[i];
double customVIX = (atrValue + stdDevValue) / 2;
if (customVIX > maxValue)
{
maxValue = customVIX;
}
if (customVIX < minValue)
{
minValue = customVIX;
}
}
return 100 * (value - minValue) / (maxValue - minValue);
}
}
}
singalgolab
Joined on 07.05.2023
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: VIX Custom.algo
- Rating: 0
- Installs: 537
Comments
This was a very interesting article. Thanks once more I will visit again.how to be a payment processor
What’s Taking place i am new to this, I stumbled upon this I have found It absolutely useful and it has aided me out loads. I hope to contribute & aid different customers like its aided me. Good job.selling payment processing
I am curious to find out what blog platform you have been using? I’m having some minor security issues with my latest website and I’d like to find something more safeguarded. Do you have any recommendations?Packers and Movers Hyderabad to Ranchi
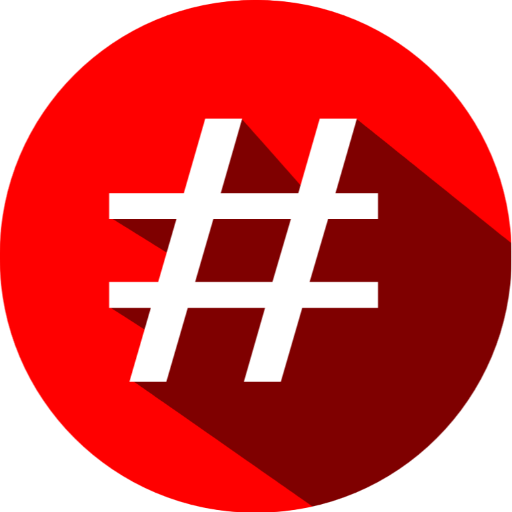
Thanks for sharing this valuable information! I completely agree with your analysis of the Custom VIX Oscillator. It's a reliable tool for assessing market volatility, and your points on higher/lower values, overbought/oversold conditions, and divergences are spot on. Great insights! ????????
That is lots of inspirational stuff. For no reason knew that opinions could be that varied. Thanks for all the enthusiasm to provide such helpful information here.how to be a credit card processor