Description
Conventional Pivot, CPR and Mid levels are optional.
Supported Day, Week and Month pivot.
PivotTimeFrame: Day, Week, Month.
ShowCPR: If Yes, also plot the Central Pivot Range levels.
ShowMids: If Yes, also plot the Mid levels.
Updated 26 Apr 2023:
- Auto enforce PivotTimeFrame to avoid slow drawing:
- If current TF > Hour: use Week pivot.
- If current TF > Hour4: use Month pivot.
- Zone: Ctrl + Click to toggle zone drawing in the dedicated pivot area. Zones are equally divided between 2 key levels.
Updated as of 08 Nov 2023:
- Removed Zone.
- Reduce noise on the screen (see parameter detail)
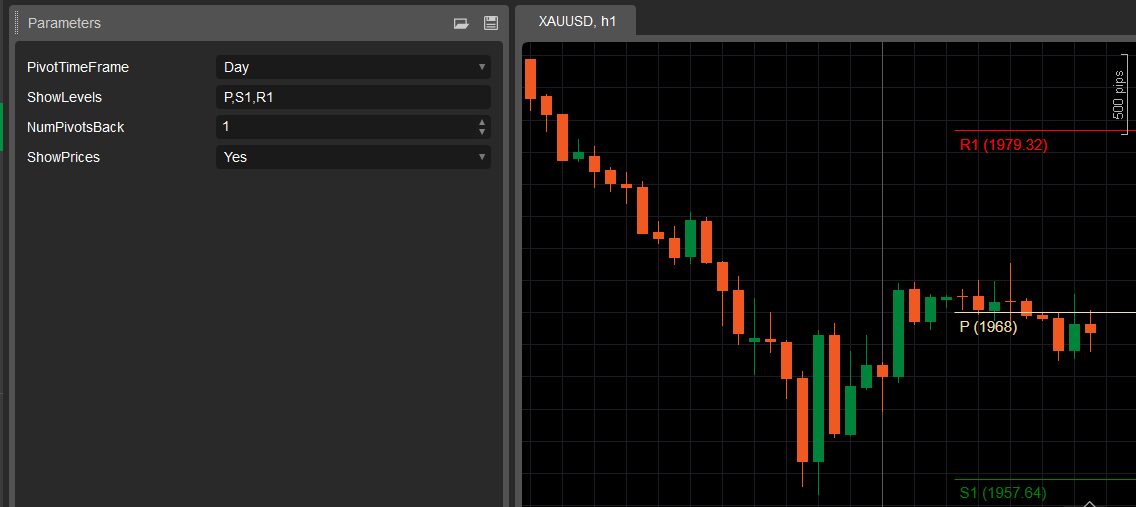
- ShowLevels: commas delimited string, default “P,S1,R1” only. Can accept from “P, S1, S2, S3, PS1, S12, S23, R1, R2, R3, PR1, R12, R23, BC, TC”.
- NumPivotsBack: number of Pivots to draw. Default is 1.
- ShowPrices: Yes (Default) to show price.
Updated as of 22 Mar 2024:
- More options.
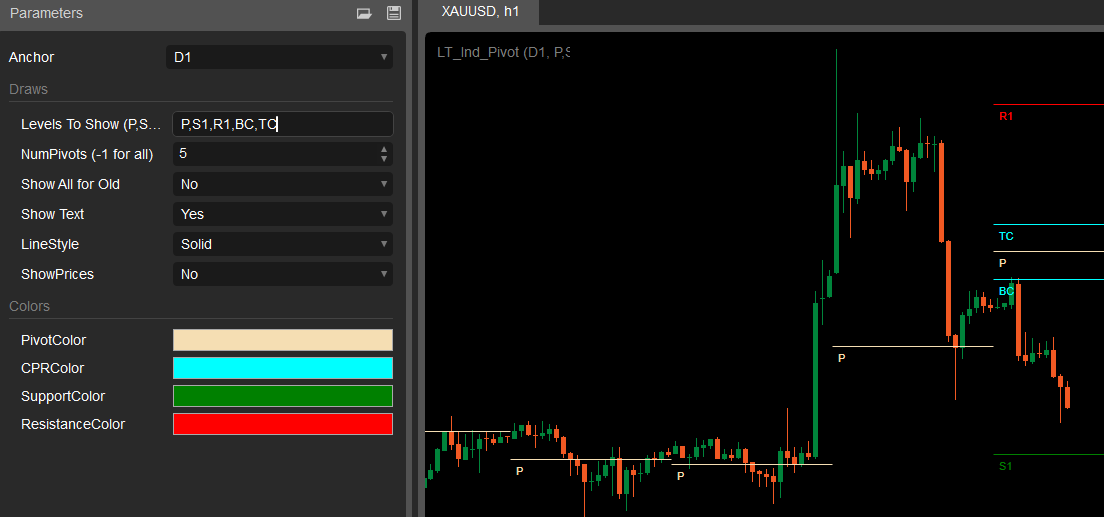
- Anchor: The Timeframe used to calculate Pivots. Auto switch same.
- Levels To Show: P,S1,R1,Sx,Rx,PSy,SySz,TC,BC x is up to 5, yz up to 3
- NumPivots: Number of Pivots to show, -1 for all available.
- Show All for Old: If Yes, Levels To Show is also applied to old Pivots, otherwise only P.
- The rest: says itself.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
using LittleTrader;
using LittleTrader.Models.PriceActions;
using LittleTrader.Extensions;
using MoreLinq;
namespace cAlgo
{
[Levels(0)]
[Indicator(IsOverlay = true, AutoRescale = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class LT_Ind_Pivot : Indicator
{
[Parameter(DefaultValue = Anchors.D1)]
public Anchors Anchor { get; set; }
[Parameter("Levels To Show (P,S1,R1,Sx,Rx,PSy,SySz,TC,BC x is up to 5, yz up to 3)", DefaultValue = "P,S1,R1", Group = "Draws")]
public string ShowLevels { get; set; }
[Parameter("NumPivots (-1 for all)",DefaultValue = 1, Group = "Draws")]
public int NumPivotsBack { get; set; }
[Parameter("Show All for Old",DefaultValue = false, Group = "Draws")]
public bool ShowAllForOldPivots { get; set; }
[Parameter("Show Text", DefaultValue = true, Group = "Draws")]
public bool ShowText { get; set; }
[Parameter(DefaultValue = LineStyle.Solid, Group = "Draws")]
public LineStyle LineStyle { get; set; }
[Parameter(DefaultValue = false, Group = "Draws")]
public bool ShowPrices { get; set; }
[Parameter(DefaultValue = "Wheat", Group = "Colors")]
public Color PivotColor { get; set; }
[Parameter(DefaultValue = "Cyan", Group = "Colors")]
public Color CPRColor { get; set; }
[Parameter(DefaultValue = "Green", Group = "Colors")]
public Color SupportColor { get; set; }
[Parameter(DefaultValue = "Red", Group = "Colors")]
public Color ResistanceColor { get; set; }
Pivot2 _pivot;
Bars _hiBars;
protected override void Initialize()
{
_hiBars = MarketData.GetBars(TimeFrame.Parse(AutoAnchor().ToString()));
_pivot = new Pivot2(_hiBars);
}
int _lastIndexHigher = -1;
public override void Calculate(int index)
{
var indexHigher = _hiBars.OpenTimes.GetIndexByTime(Bars.OpenTimes[index]);
if (_lastIndexHigher != indexHigher)
{
if (NumPivotsBack == -1 || indexHigher >= _hiBars.Count - NumPivotsBack)
{
var start = _hiBars.OpenTimes[indexHigher];
var end = start.AddHours(HoursSpan(AutoAnchor()));
ShowLevels.CommaSplit().ForEach(x =>
{
if (x == "P" || ShowAllForOldPivots || indexHigher == _hiBars.Count - 1)
DrawLevel(indexHigher - 1, x, start, end);
});
}
_lastIndexHigher = indexHigher;
}
}
void DrawLevel(int indexHigher, string name, DateTime start, DateTime end)
{
var key = $"{this.GetHashCode()}_{name}_{start.Ticks}";
var color = PivotColor;
if (name.Contains('S')) color = SupportColor;
else if (name.Contains('R')) color = ResistanceColor;
else if (name.Contains("C")) color = CPRColor;
double val = _pivot.Level(name, indexHigher);
if (ShowText)
{
string pstr = ShowPrices ? $"({val.MathRound(Symbol.Digits)})" : string.Empty;
Chart.DrawText($"{key}_t", $"{name} {pstr}", start, val, color).FontSize = 8.75;
}
var line = Chart.DrawTrendLine(key, start, val, end, val, color);
line.LineStyle = LineStyle;
if (name.Length > 2)
line.LineStyle = LineStyle.DotsRare;
}
Anchors AutoAnchor()
{
if (TimeFrame > TimeFrame.Daily) return Anchors.Month1;
else if (TimeFrame > TimeFrame.Hour) return Anchors.W1;
else return Anchor;
}
int HoursSpan(Anchors anchor)
{
switch (anchor)
{
case Anchors.h1:
return 1;
case Anchors.h4:
return 4;
case Anchors.h8:
return 8;
case Anchors.h12:
return 12;
case Anchors.D1:
return 24;
case Anchors.W1:
return 24 * 7;
case Anchors.Month1:
return 24 * 30;
default:
return 24;
}
}
}
public enum Anchors { h1, h4, h8, h12, D1, W1, Month1 };
}
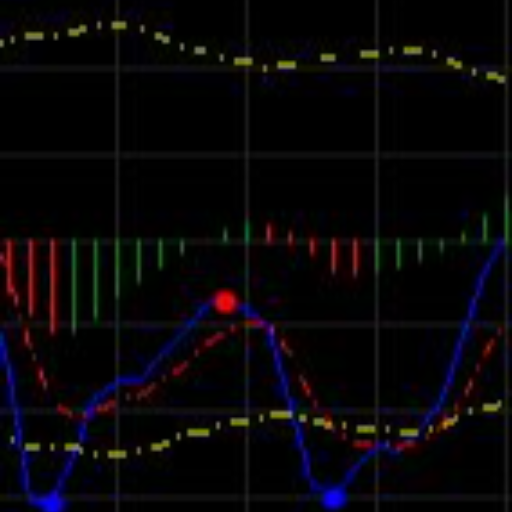
dhnhuy
Joined on 03.04.2023
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: LT_Ind_Pivot.algo
- Rating: 5
- Installs: 519
- Modified: 22/03/2024 09:31
Comments
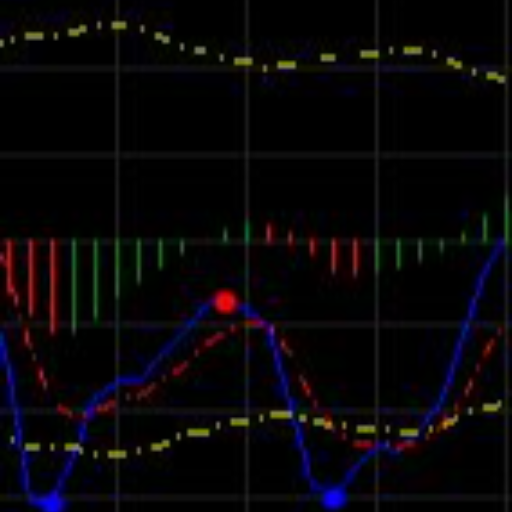
Hi w.b.z,
Sorry for the late reply, I did come here for a couple months, and it did not drop me email either.
The custom reference should be embedded in the *.algo file. You should not have problem when build from ctrader provided compiler. Are you building it from Visual Studio?
Thanks,
Huy
Hi dhnhuy,
Thanks for all your great work and Discriptions!
One Question:
In the code you added:
using LittleTrader;
using LittleTrader.Models.PriceActions;
are those your selfmade references?
I have errors building you codes.
Please tell me how to get all you GREAT work to compile withou errors.
Cheers
thanks
It works like a charm.
Thank you.
Плавна та безпечна їзда починається з правильних шин та дисків. Я знайшов чудове джерело для цих важливих компонентів на сайті https://goroshina.ua/ . Широкий вибір, конкурентоспроможні ціни та простий у користуванні сайт роблять покупку шин і дисків безтурботним процесом. Я дуже задоволений своєю покупкою і настійно рекомендую цей сайт для всіх ваших потреб у шинах і колесах.
Hi dhnhuy,
Can you add the function of hide Show Label for customize.
Thank you.