Description
The Ehlers MESA Adaptive Moving Average in 2 time frames, the current timeframe and a selectable higher timeframe.
Read Ehlers article about MAMA for detail, here is some text from his article:
"The alpha in MAMA is allowed to range between a maximum and minimum
value, these values being established as inputs. The suggested maximum value is
FastLimit = 0.5 and the suggested minimum is SlowLimit = 0.05. The variable alpha is
computed as the FastLimit divided by the phase rate of change. Any time there is a
negative phase rate of change the value of alpha is set to the FastLimit because the
phase rate of change can be no less than 1. If the phase rate of change is large, the
variable alpha is bounded at the SlowLimit. This keeps MAMA from reacting to the
shorter market cycles."
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using LittleTrader.Extensions;
namespace cAlgo
{
[Indicator(AccessRights = AccessRights.None, IsOverlay = true), Cloud("MamaThis", "FamaThis"), Cloud("MamaHigher", "FamaHigher")]
public class LT_Ind_EhlersMA_MultiF : Indicator
{
[Parameter(DefaultValue = "Hour")]
public TimeFrame TimeFrameHigher { get; set; }
[Parameter("Fast Limit", DefaultValue = 0.5)]
public double FastLimit { get; set; }
[Parameter("Slow Limit", DefaultValue = 0.05)]
public double SlowLimit { get; set; }
[Output("MamaThis", LineColor = "White", PlotType = PlotType.Line)]
public IndicatorDataSeries MamaThis { get; set; }
[Output("FamaThis", LineColor = "Blue", PlotType = PlotType.Line)]
public IndicatorDataSeries FamaThis { get; set; }
[Output("MamaHigher", LineColor = "Green", PlotType = PlotType.Line)]
public IndicatorDataSeries MamaHigher { get; set; }
[Output("FamaHigher", LineColor = "Red", PlotType = PlotType.Line)]
public IndicatorDataSeries FamaHigher { get; set; }
LT_Ind_EhlersMA _thisMa, _higherMa;
Bars _barsHigher;
protected override void Initialize()
{
_barsHigher = MarketData.GetBars(TimeFrameHigher);
_thisMa = Indicators.GetIndicator<LT_Ind_EhlersMA>(Bars.ClosePrices, FastLimit, SlowLimit);
_higherMa = Indicators.GetIndicator<LT_Ind_EhlersMA>(_barsHigher.ClosePrices, FastLimit, SlowLimit);
}
public override void Calculate(int index)
{
MamaThis[index] = _thisMa.Mama[index];
FamaThis[index] = _thisMa.Fama[index];
MamaHigher.ReflectHigherTF(_higherMa.Mama, Bars, _barsHigher, index);
FamaHigher.ReflectHigherTF(_higherMa.Fama, Bars, _barsHigher, index);
}
}
}
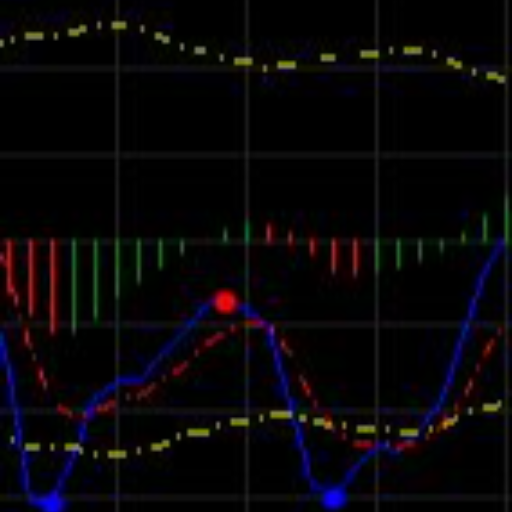
dhnhuy
Joined on 03.04.2023
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: LT_Ind_EhlersMA_MultiF.algo
- Rating: 0
- Installs: 749
- Modified: 03/04/2023 07:32