Description
The T3 Velocity indicator is an entirely new technical study that deploys the T3 in calculating velocity, on the other hand, it does not deploy the T3 when smoothening velocity.
In as much as velocity is a considerably smoother momentum, using the T3 makes it even smoother.
This indicator includes the original Tim Tillson calculation alongside the enhanced Fulks/Mutulich calculation, in value range from -100 to +100.
using System;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Levels(0)]
[Indicator(AccessRights = AccessRights.None)]
public class mT3velocity : Indicator
{
[Parameter("Period (14)", DefaultValue = 14)]
public int inpPeriod { get; set; }
[Parameter("Factor (1.0)", DefaultValue = 1, Step = 0.01, MinValue = 0, MaxValue = 1.0)]
public double inpFactor { get; set; }
[Parameter("Original (false)", DefaultValue = false)]
public bool inpOriginal { get; set; }
[Parameter("Normalisation Period (20)", DefaultValue = 20)]
public int inpPeriodNormalisation { get; set; }
[Output("T3 Full", LineColor = "Black", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outT3n { get; set; }
private int _per, _per2;
private double _f, _c1, _c2, _c3, _c4, _alphaa, _alphab;
private IndicatorDataSeries _t30a, _t31a, _t32a, _t33a, _t34a, _t35a, _t3a, _t30b, _t31b, _t32b, _t33b, _t34b, _t35b, _t3b, _out, _smin, _smax, _sto;
protected override void Initialize()
{
_f = inpFactor;
_per = inpPeriod;
_per2 = Convert.ToInt16(inpPeriod/2);
_c1 = -_f * _f * _f;
_c2 = 3 * _f * _f + 3 * _f * _f * _f;
_c3 = -6 * _f * _f - 3 * _f - 3 * _f * _f * _f;
_c4 = 1 + 3 * _f + _f * _f * _f + 3 * _f * _f;
_alphaa = inpOriginal == true ? 2.0 / (1.0 + _per) : 2.0 / (2.0 + (_per - 1.0) / 2.0);
_alphab = inpOriginal == true ? 2.0 / (1.0 + _per2) : 2.0 / (2.0 + (_per2 - 1.0) / 2.0);
_t30a = CreateDataSeries();
_t31a = CreateDataSeries();
_t32a = CreateDataSeries();
_t33a = CreateDataSeries();
_t34a = CreateDataSeries();
_t35a = CreateDataSeries();
_t3a = CreateDataSeries();
_t30b = CreateDataSeries();
_t31b = CreateDataSeries();
_t32b = CreateDataSeries();
_t33b = CreateDataSeries();
_t34b = CreateDataSeries();
_t35b = CreateDataSeries();
_t3b = CreateDataSeries();
_out = CreateDataSeries();
_smin = CreateDataSeries();
_smax = CreateDataSeries();
_sto = CreateDataSeries();
}
public override void Calculate(int i)
{
_t30a[i] = i>1 ? _t30a[i-1] + _alphaa * (Bars.TypicalPrices[i] - _t30a[i-1]) : Bars.TypicalPrices[i];
_t31a[i] = i>1 ? _t31a[i-1] + _alphaa * _t30a[i] - _t31a[i-1] : _t30a[i];
_t32a[i] = i>1 ? _t32a[i-1] + _alphaa * _t31a[i] - _t32a[i-1] : _t31a[i];
_t33a[i] = i>1 ? _t33a[i-1] + _alphaa * _t32a[i] - _t33a[i-1] : _t32a[i];
_t34a[i] = i>1 ? _t34a[i-1] + _alphaa * _t33a[i] - _t34a[i-1] : _t33a[i];
_t35a[i] = i>1 ? _t35a[i-1] + _alphaa * _t34a[i] - _t35a[i-1] : _t34a[i];
_t3a[i] = _c1 * _t35a[i] + _c2 * _t34a[i] +_c3 * _t33a[i] + _c4 * _t32a[i];
_t30b[i] = i>1 ? _t30b[i-1] + _alphab * (Bars.TypicalPrices[i] - _t30b[i-1]) : Bars.TypicalPrices[i];
_t31b[i] = i>1 ? _t31b[i-1] + _alphab * _t30b[i] - _t31b[i-1] : _t30b[i];
_t32b[i] = i>1 ? _t32b[i-1] + _alphab * _t31b[i] - _t32b[i-1] : _t31b[i];
_t33b[i] = i>1 ? _t33b[i-1] + _alphab * _t32b[i] - _t33b[i-1] : _t32b[i];
_t34b[i] = i>1 ? _t34b[i-1] + _alphab * _t33b[i] - _t34b[i-1] : _t33b[i];
_t35b[i] = i>1 ? _t35b[i-1] + _alphab * _t34b[i] - _t35b[i-1] : _t34b[i];
_t3b[i] = _c1 * _t35b[i] + _c2 * _t34b[i] +_c3 * _t33b[i] + _c4 * _t32b[i];
_out[i] = _t3b[i] - _t3a[i];
_smin[i] = _out.Minimum(inpPeriodNormalisation);
_smax[i] = _out.Maximum(inpPeriodNormalisation);
_sto[i] = (200 * (_out[i] - _smin[i]) / (_smax[i] - _smin[i])) - 100;
outT3n[i] = _sto[i];
}
}
}
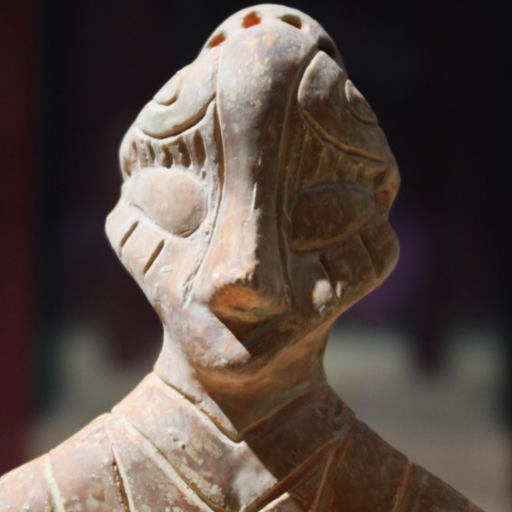
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mT3velocity.algo
- Rating: 5
- Installs: 504
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.