Description
The Original formula of Balance Of Power is calculated as the ratio of the differences between the Close and the Open and between the High and the Low. It can also be smoothed with a moving average of a selected type.
The Balance Of Power is an oscillator showing strength of the bulls versus bears measured by the ability of each to push prices to extreme levels. Positive values of the oscillator signify an uptrend, while negative ones signify a downtrend.
In this version you have raw component smoothed by SMA and FIR, derived from source smoothed also by FIR (Finite Impulse Response Filter).
The Balance Of Power indicator you can download from here
the FIR (Finite Impulse Response Filter) indicator you can download from here
using System;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Levels(0)]
[Indicator(AccessRights = AccessRights.None)]
public class mBalanceOfPowerFIR : Indicator
{
[Parameter("Period (14)",DefaultValue = 14)]
public int inpPeriod { get; set; }
[Output("Blance Of Power FIR", IsHistogram = false, LineColor = "Black", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outBOPFIR { get; set; }
[Output("Blance Of Power Smooth", IsHistogram = false, LineColor = "Red", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outBOPFIRsmooth { get; set; }
private IndicatorDataSeries _firh, _firl, _firo, _firc, _bopraw, _bop;
private MovingAverage _bops;
protected override void Initialize()
{
_firh = CreateDataSeries();
_firl = CreateDataSeries();
_firo = CreateDataSeries();
_firc = CreateDataSeries();
_bopraw = CreateDataSeries();
_bop = CreateDataSeries();
_bops = Indicators.MovingAverage(_bopraw, inpPeriod, MovingAverageType.Simple);
}
public override void Calculate(int i)
{
_firh[i] = i>3 ? (Bars.HighPrices[i] + 2.0 * Bars.HighPrices[i-1] + 2.0 * Bars.HighPrices[i-2] + Bars.HighPrices[i-3]) / 6.0 : Bars.HighPrices[i];
_firl[i] = i>3 ? (Bars.LowPrices[i] + 2.0 * Bars.LowPrices[i-1] + 2.0 * Bars.LowPrices[i-2] + Bars.LowPrices[i-3]) / 6.0 : Bars.LowPrices[i];
_firo[i] = i>3 ? (Bars.OpenPrices[i] + 2.0 * Bars.OpenPrices[i-1] + 2.0 * Bars.OpenPrices[i-2] + Bars.OpenPrices[i-3]) / 6.0 : Bars.OpenPrices[i];
_firc[i] = i>3 ? (Bars.ClosePrices[i] + 2.0 * Bars.ClosePrices[i-1] + 2.0 * Bars.ClosePrices[i-2] + Bars.ClosePrices[i-3]) / 6.0 : Bars.ClosePrices[i];
_bopraw[i] = (_firc[i] - _firo[i]) / (_firh[i] - _firl[i]);
_bop[i] = i>3 ? (_bopraw[i] + 2.0 * _bopraw[i-1] + 2.0 * _bopraw[i-2] + _bopraw[i-3]) / 6.0 : _bopraw[i];
outBOPFIR[i] = _bop[i];
outBOPFIRsmooth[i] = _bops.Result[i];
}
}
}
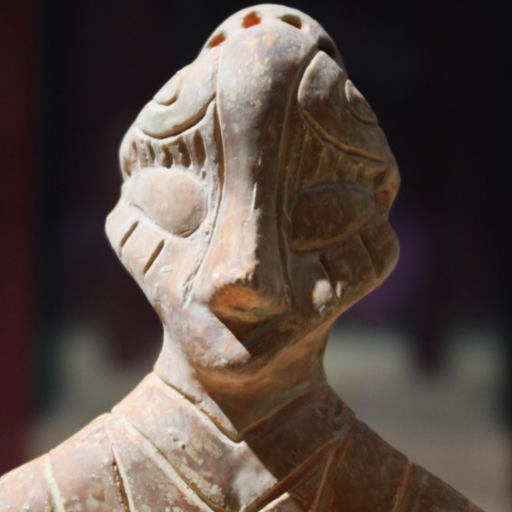
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mBalanceOfPowerFIR.algo
- Rating: 5
- Installs: 518
I just read this article https://school.stockcharts.com/doku.php?id=technical_indicators:balance_of_power trap the cat and I think it's not correct so check it out.