Description
What Is the Average Directional Index (ADX)?
The average directional index (ADX) is a technical analysis indicator used by some traders to determine the strength of a trend.
The trend can be either up or down, and this is shown by two accompanying indicators, the negative directional indicator (-DI) and the positive directional indicator (+DI). Therefore, the ADX commonly includes three separate lines. These are used to help assess whether a trade should be taken long or short, or if a trade should be taken at all.
- Designed by Welles Wilder for commodity daily charts, the ADX is now used in several markets by technical traders to judge the strength of a trend.
- The ADX makes use of a positive (+DI) and negative (-DI) directional indicator in addition to the trendline.
- The trend has strength when ADX is above 25; the trend is weak or the price is trendless when ADX is below 20, according to Wilder.
- Non-trending doesn't mean the price isn't moving. It may not be, but the price could also be making a trend change or is too volatile for a clear direction to be present.
As an additional component, the Threshold level component is incorporated, defined and calculated automatically in a way that Wilder and all traders till now have not analyzed. This component is defined by a formula from my mentor and brother, Iba.
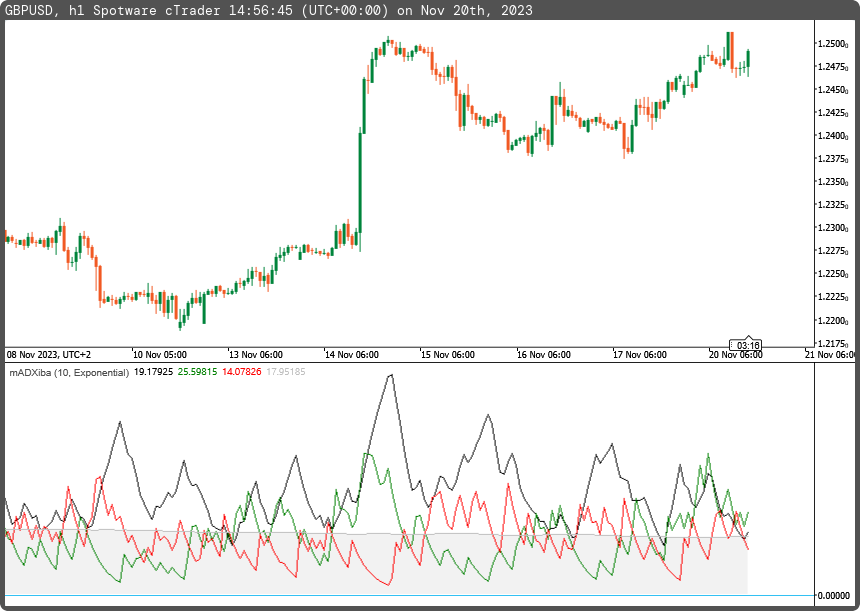
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Cloud("Threshold", "Threshold0", FirstColor = "Silver", SecondColor = "Silver", Opacity = 0.2)]
[Levels(0)]
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class mADXiba : Indicator
{
[Parameter("Period (10)", DefaultValue = 10)]
public int inpPeriod { get; set; }
[Parameter("Smooth Type (ema)", DefaultValue = MovingAverageType.Exponential)]
public MovingAverageType inpSmoothType { get; set; }
[Output("ADX", LineColor = "Black", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outADX { get; set; }
[Output("DI+", LineColor = "Green", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outDIpositive { get; set; }
[Output("DI-", LineColor = "Red", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outDInegative { get; set; }
[Output("Threshold", LineColor = "Silver", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outThreshold { get; set; }
[Output("Threshold0", LineColor = "Transparent", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outThreshold0 { get; set; }
private IndicatorDataSeries _deltahigh, _deltalow, _pos, _neg, _bulls, _bears, _tr, _pd, _nd, _sum, _rawadx;
private MovingAverage _pdsmooth, _ndsmooth, _threshold, _adx;
protected override void Initialize()
{
_deltahigh = CreateDataSeries();
_deltalow = CreateDataSeries();
_pos = CreateDataSeries();
_neg = CreateDataSeries();
_bulls = CreateDataSeries();
_bears = CreateDataSeries();
_tr = CreateDataSeries();
_pd = CreateDataSeries();
_nd = CreateDataSeries();
_pdsmooth = Indicators.MovingAverage(_pd, inpPeriod, inpSmoothType);
_ndsmooth = Indicators.MovingAverage(_nd, inpPeriod, inpSmoothType);
_sum = CreateDataSeries();
_threshold = Indicators.MovingAverage(_sum, 200, MovingAverageType.Simple);
_rawadx = CreateDataSeries();
_adx = Indicators.MovingAverage(_rawadx, inpPeriod, MovingAverageType.Exponential);
}
public override void Calculate(int i)
{
_deltahigh[i] = Bars.HighPrices[i] - (i>1 ? Bars.HighPrices[i-1] : Bars.TypicalPrices[i]);
_deltalow[i] = (i>1 ? Bars.LowPrices[i-1] : Bars.TypicalPrices[i]) - Bars.LowPrices[i];
_pos[i] = _deltahigh[i] > 0 ? _deltahigh[i] : 0;
_neg[i] = _deltalow[i] > 0 ? _deltalow[i] : 0;
_bulls[i] = _pos[i] > _neg[i] ? _pos[i] : 0;
_bears[i] = _pos[i] < _neg[i] ? _neg[i] : 0;
_tr[i] = Math.Max(Math.Max(Math.Abs(Bars.HighPrices[i] - Bars.LowPrices[i]), Math.Abs(Bars.HighPrices[i] - Bars.ClosePrices[i-(i>1 ? 1 : 0)])), Math.Abs(Bars.LowPrices[i] - Bars.ClosePrices[i-(i>1 ? 1 : 0)]));
_pd[i] = _tr[i] != 0 ? 100.0 * _bulls[i] / _tr[i] : 0;
_nd[i] = _tr[i] != 0 ? 100.0 * _bears[i] / _tr[i] : 0;
_sum[i] = (_pdsmooth.Result[i] + _ndsmooth.Result[i]) / 2;
_rawadx[i] = _pdsmooth.Result[i] + _ndsmooth.Result[i] != 0 ? 100.0 * Math.Abs((_pdsmooth.Result[i] - _ndsmooth.Result[i]) / (_pdsmooth.Result[i] + _ndsmooth.Result[i])) : 0;
outADX[i] = _adx.Result[i];
outDIpositive[i] = _pdsmooth.Result[i];
outDInegative[i] = _ndsmooth.Result[i];
outThreshold[i] = _threshold.Result[i];
outThreshold0[i] = 0;
}
}
}
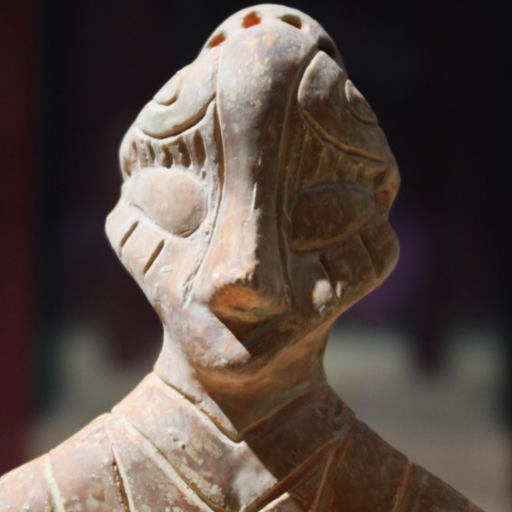
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mADXiba.algo
- Rating: 5
- Installs: 477
- Modified: 20/11/2023 14:59