Warning! This section will be deprecated on February 1st 2025. Please move all your Indicators to the cTrader Store catalogue.
An update for this algorithm is currently pending moderation. Please revisit this page shortly to access the algorithm's latest version.
Description
The SZO (Sentiment Zone Oscillator) indicator shows the market sentiment (activity and direction) and zones of excessive activity (overbought/oversold zones). It can display a dynamic channel, beyond which deals are seen as undesirable because of the high probability of a change in sentiment and of reversal.
If the indicator line moves beyond the channel and at the same time enters the overbought/oversold zone, this may mean that the market trend can change soon. The indicator often warns of such a possible change in advance, so it is advisable to use it in combination with another confirmation indicator.
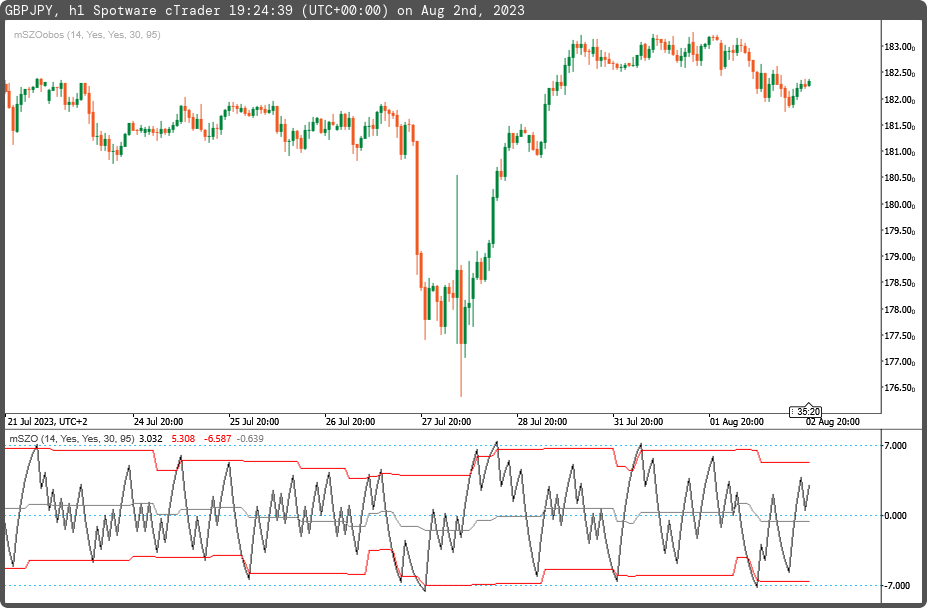
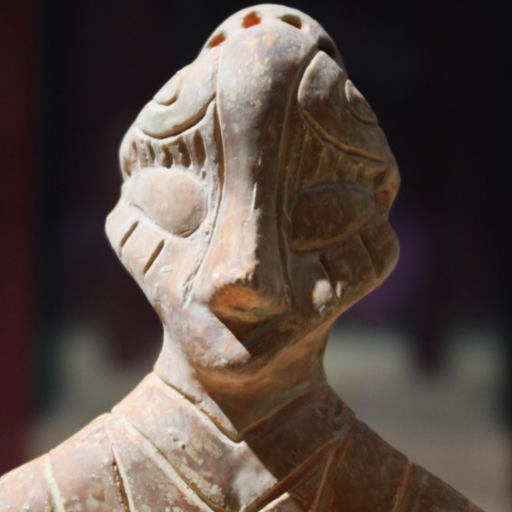
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mSZO.algo
- Rating: 5
- Installs: 444
- Modified: 02/08/2023 19:22
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
MF
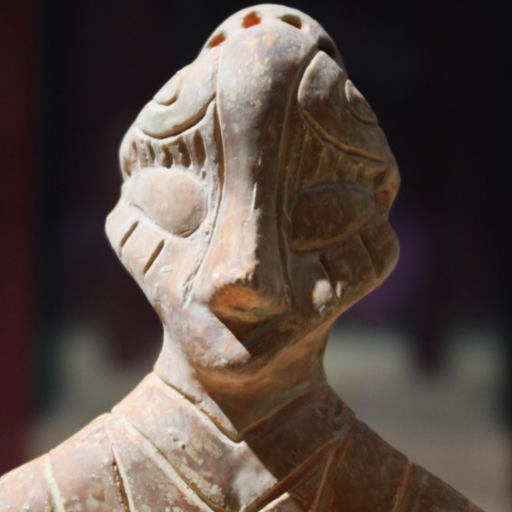
mfejza
·
1 year ago