Description
The custom indicator 'Adaptive Market Level' displays one of three market states: Flat, uptrend, and downtrend, and these states are defined by the slope direction of the indicator value.
This custom indicator is based on fractal smoothing and incorporates a discrete filter that removes small price movements. If the amplitude of the price movement does not exceed the square of the predefined amplitude within the specified range, then this price movement will be ignored and considered flat.
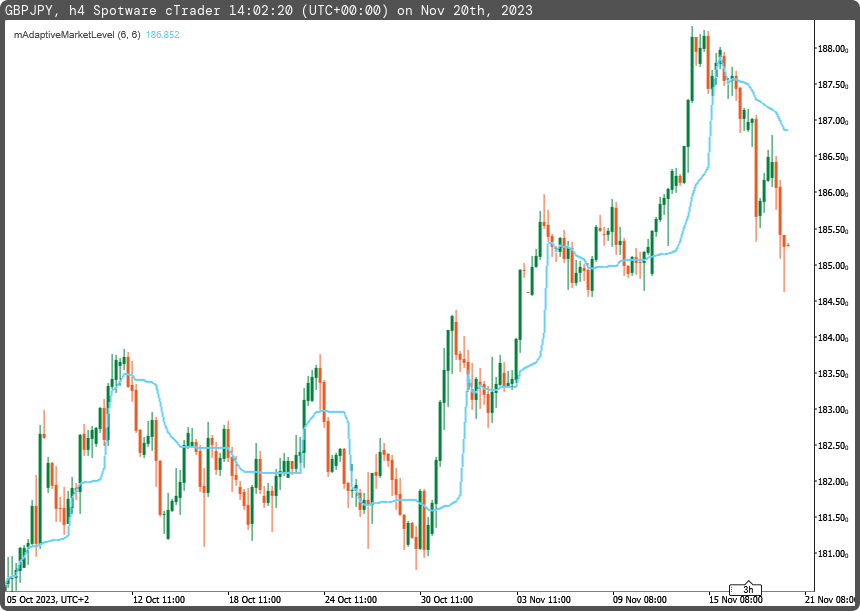
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class mAdaptiveMarketLevel : Indicator
{
[Parameter("Periods (6)", DefaultValue = 6)]
public int inpPeriods { get; set; }
[Parameter("Dimension (7)", DefaultValue = 6)]
public int inpDimension { get; set; }
[Output("Adaptive Market Level", LineColor = "DodgerBlue", PlotType = PlotType.Line, Thickness = 1)]
public IndicatorDataSeries outAML { get; set; }
private IndicatorDataSeries _range, _range3, _fr;
private int fractal, lag, period_max;
private double lag2;
protected override void Initialize()
{
fractal = (inpPeriods < 1 ? 1 : inpPeriods);
lag = (inpDimension < 1 ? 1 : inpDimension);
period_max = Math.Max(fractal, lag);
lag2 = lag * lag * Symbol.TickSize;
_range = CreateDataSeries();
_range3 = CreateDataSeries();
_fr = CreateDataSeries();
}
public override void Calculate(int i)
{
_range[i] = i > fractal ? ((Bars.HighPrices.Maximum(fractal) - Bars.LowPrices.Minimum(fractal)) / fractal) : Bars.TypicalPrices[i];
_range3[i] = i > fractal * 2 ? ((Bars.HighPrices.Maximum(fractal * 2) - Bars.LowPrices.Minimum(fractal * 2)) / (2.0 * fractal)) : Bars.MedianPrices[i];
double dim = (_range[i] + (i > 1 ? _range[i - 1] : Bars.TypicalPrices[i]) > 0 && _range3[i] > 0 ? 1.44269504088896 * (Math.Log(_range[i] + (i > 1 ? _range[i - 1] : Bars.TypicalPrices[i])) - Math.Log(_range3[i])) : 0);
double alpha = Math.Max(Math.Min(Math.Exp(-1.0 * lag * (dim - 1.0)), 1.0), 0.01);
double price = (Bars.HighPrices[i] + Bars.LowPrices[i] + 2.0 * Bars.OpenPrices[i] + 2.0 * Bars.ClosePrices[i]) / 6.0;
_fr[i] = alpha * price + (1.0 - alpha) * (i > 1 ? _fr[i - 1] : Bars.ClosePrices[i]);
outAML[i] = (Math.Abs(_fr[i] - _fr[i - lag]) < lag2 ? (i > 1 ? outAML[i - 1] : Bars.ClosePrices[i]) : _fr[i]);
}
}
}
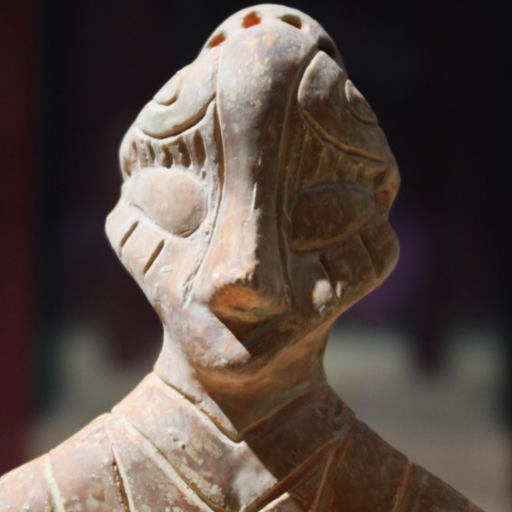
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mAdaptiveMarketLevel.algo
- Rating: 5
- Installs: 170
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.