Description
NEW VERSION THIS BOT: https://ctrader.com/algos/show/4199
It uses several parameters such as Initial Volume, Stop Loss, Take Profit, Trailing Stop Loss, and Spread Limit to execute trades on the market.
The robot has a random trade type generator that can either select a buy or sell trade. The robot also has a closed position event handler that will execute a new trade if the previous one closed with a profit, or will double the volume of the previous trade if the previous one closed with a loss. Additionally, it stops the bot if it runs out of money.
Tip: Run extended optimization to get better parameters, at least 2 years. The print values are for only 1 week.
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots {
// Attribute to set the robot's time zone and access rights
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class SampleMartingaleRobot: Robot {
// Parameters for initial volume, stop loss, take profit, trailing stop loss, and spread limit
[Parameter("Initial Volume", DefaultValue = 1000, MinValue = 0)]
public int InitialVolume {
get;
set;
}
[Parameter("Stop Loss", DefaultValue = 15)]
public int StopLoss {
get;
set;
}
[Parameter("Take Profit", DefaultValue = 24)]
public int TakeProfit {
get;
set;
}
[Parameter("Trailing Stop Loss", DefaultValue = 21)]
public int TrailingStopLoss {
get;
set;
}
[Parameter("Spread Limit", DefaultValue = 0.5)]
public double SpreadLimit {
get;
set;
}
// Random number generator
private Random random = new Random();
// Function that is called when the robot starts
protected override void OnStart() {
// Event handler for when positions are closed
Positions.Closed += OnPositionsClosed;
// Check if spread is within the limit and execute an initial order
if (Symbol.Spread <= SpreadLimit) {
ExecuteOrder(InitialVolume, GetRandomTradeType());
}
}
// Function to execute an order
private void ExecuteOrder(long volume, TradeType tradeType) {
// Check if spread is within the limit
if (Symbol.Spread <= SpreadLimit) {
var result = ExecuteMarketOrder(tradeType, SymbolName, volume, "Martingale", StopLoss, TakeProfit);
// Modify stop loss if order execution is successful
if (result.IsSuccessful) {
result.Position.ModifyStopLossPips(TrailingStopLoss);
}
// If error is not enough money, stop the robot
if (result.Error == ErrorCode.NoMoney)
Stop();
}
}
// Event handler function for when positions are closed
private void OnPositionsClosed(PositionClosedEventArgs args) {
Print("Closed");
var position = args.Position;
// Check if the closed position has the correct label and symbol
if (position.Label != "Martingale" || position.SymbolName != SymbolName)
return;
// If the position has a positive gross profit, execute another order with the initial volume
if (position.GrossProfit > 0) {
if (Symbol.Spread <= SpreadLimit) {
ExecuteOrder(InitialVolume, GetRandomTradeType());
}
}
// If the position has a negative gross profit, execute another order with double the previous volume
else {
if (Symbol.Spread <= SpreadLimit) {
ExecuteOrder((int) position.VolumeInUnits * 2, position.TradeType);
}
}
}
// Function to generate a random trade type (buy or sell)
private TradeType GetRandomTradeType() {
return random.Next(2) == 0 ? TradeType.Buy : TradeType.Sell;
}
}
}
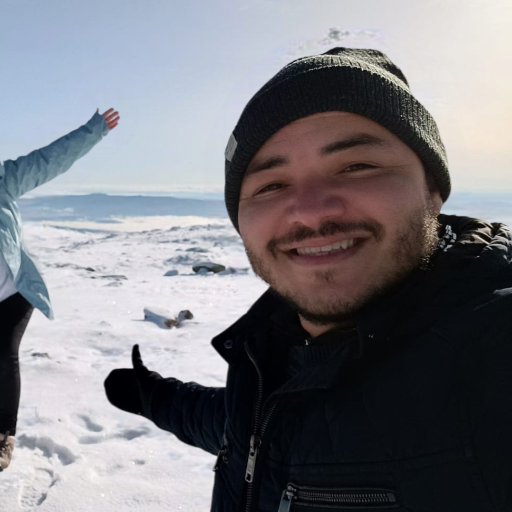
WillBS
Joined on 05.08.2021
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Martingale Trailling.algo
- Rating: 0
- Installs: 1755
- Modified: 13/01/2023 01:31
Comments
Good
It is known as the Martingale strategy, which in essence, is based on probability theory. It has a near 100% success rate if your pocket is deep enough. This strategy is based on the premise that one good bet or trade is able to turn your fortunes around. This is because it relies on the theory of mean reversion.
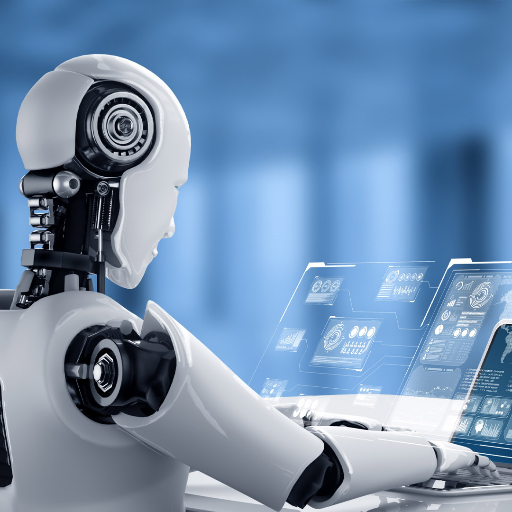
Hello!
can you please modifi some parameters so we can use smaller volumes? like minimum to be 0.01.
Thanks a lot!
The Martingale with Trailing Stop is a trading strategy that combines the Martingale money management system with the use of a trailing stop. This is an effective way to limit risk and maximize profits as the trader can scale out of a position as the price moves in their favor. The trailing stop is used to lock in profits and protect the trader from unfavorable market movements. https://keepnetworth.com/
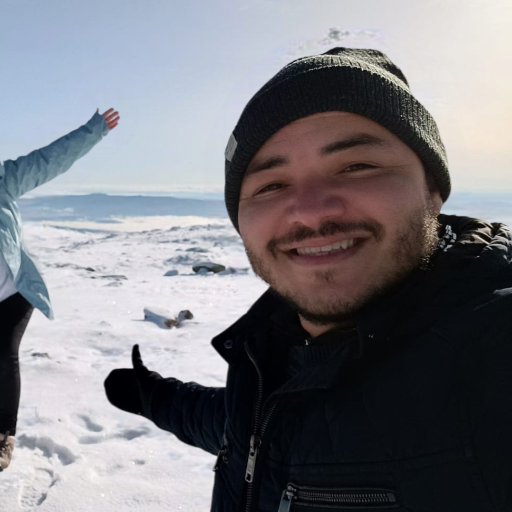
cdc1918, can you do something similar using ChatGPT?
I change the code on the losing trade. Make the bot totally randoms buy/sell. It helps reducing Max consecutive Losing trade a lot. It works great in the long rally bull/bear. (original bot Max consec = 12-14 times, Modified bot Max consec = 8-9 times) back test data from 1-Jan-23 to 15-Sep-23.
I will try another idea of placing opposite side of the previous losing trade and I will update later.
// If the position has a negative gross profit, execute another order with double the previous volume
else {
if (Symbol.Spread <= SpreadLimit) {
ExecuteOrder((int) position.VolumeInUnits * 2, GetRandomTradeType());