Description
The indicator represents a combination of RSI and STARC components (Stoller Average Range Channels), which is similar to indicator Bollinger Bands.
Its bands get narrower on stable markets and wider on volatile markets. STARC component does not consider standard square deviation as BBands, its is calculated using average true range (ATR), and its components are derived from RSI, for providing more detailed information on market volatility.
using System;
using System.Text;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Levels(30, 50, 70)]
[Indicator(AccessRights = AccessRights.None)]
public class mRSIstarc : Indicator
{
[Parameter("RSI Periods (14)", DefaultValue = 14)]
public int inpPeriodsRSI { get; set; }
[Parameter("ATR Periods (10)", DefaultValue = 10)]
public int inpPeriodsATR { get; set; }
[Parameter("Smooth Periods (14)", DefaultValue = 14)]
public int inpPeriodsSmooth { get; set; }
[Parameter("Multiplier Top (2.0)", DefaultValue = 2.0)]
public double inpMultiplierTop { get; set; }
[Parameter("Multiplier Bottom (2.0)", DefaultValue = 2.0)]
public double inpMultiplierBottom { get; set; }
[Output("RSI", LineColor = "Black", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outRSI { get; set; }
[Output("Top", LineColor = "Red", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outTop { get; set; }
[Output("Middle", LineColor = "Silver", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outMid { get; set; }
[Output("Bottom", LineColor = "Green", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outBottom { get; set; }
private RelativeStrengthIndex _rsi;
private MovingAverage _center, _atrma;
private IndicatorDataSeries _tr, _top, _bottom;
protected override void Initialize()
{
_rsi = Indicators.RelativeStrengthIndex(Bars.ClosePrices, inpPeriodsRSI);
_center = Indicators.MovingAverage(_rsi.Result, inpPeriodsRSI, MovingAverageType.Simple);
_tr = CreateDataSeries();
_atrma = Indicators.MovingAverage(_tr, inpPeriodsATR, MovingAverageType.Simple);
_top = CreateDataSeries();
_bottom = CreateDataSeries();
}
public override void Calculate(int i)
{
_tr[i] = i>inpPeriodsRSI ? Math.Abs(_rsi.Result[i] - _rsi.Result[i-1]) : Math.Abs(_rsi.Result[i] - 50.0);
_top[i] = _center.Result[i] + inpMultiplierTop * _atrma.Result[i];
_bottom[i] = _center.Result[i] - inpMultiplierTop * _atrma.Result[i];
outRSI[i] = _rsi.Result[i];
outTop[i] = _top[i];
outMid[i] = _center.Result[i];
outBottom[i] = _bottom[i];
}
}
}
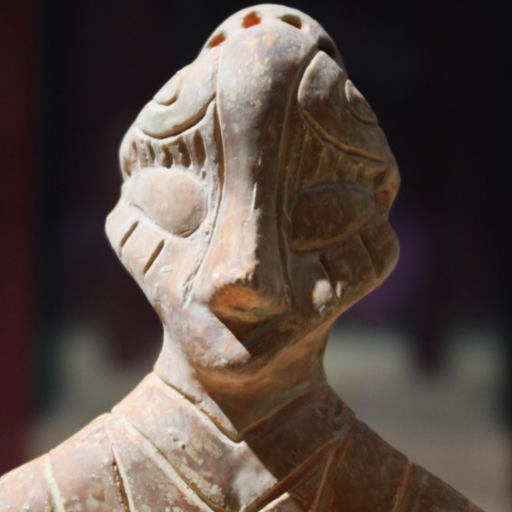
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mRSIstarc.algo
- Rating: 5
- Installs: 804
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
KE
@noone
My Gmail is kevinssendawula@gmail.com
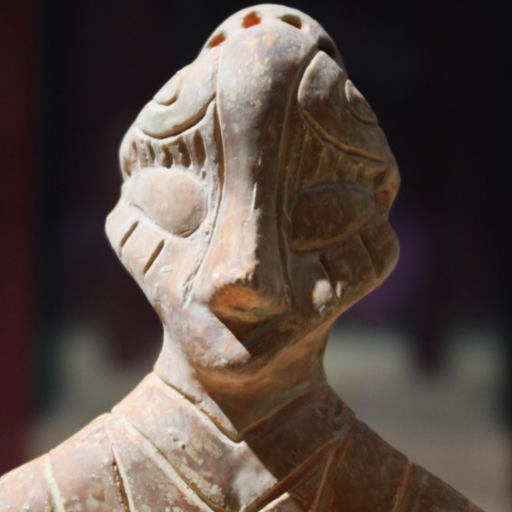
@kevinssendawula
send me a email account where to send it?
KE
this is the best indicator i hva e seen in a while.
but how do i also get the second oscillator in that image. thank you
Thank so much