Description
Trend Score indicator by Tushar S. Chande was described in the article "Rating Trend Strength" in S&C magazine (September 1993).
It displays both the power and direction of a trend.
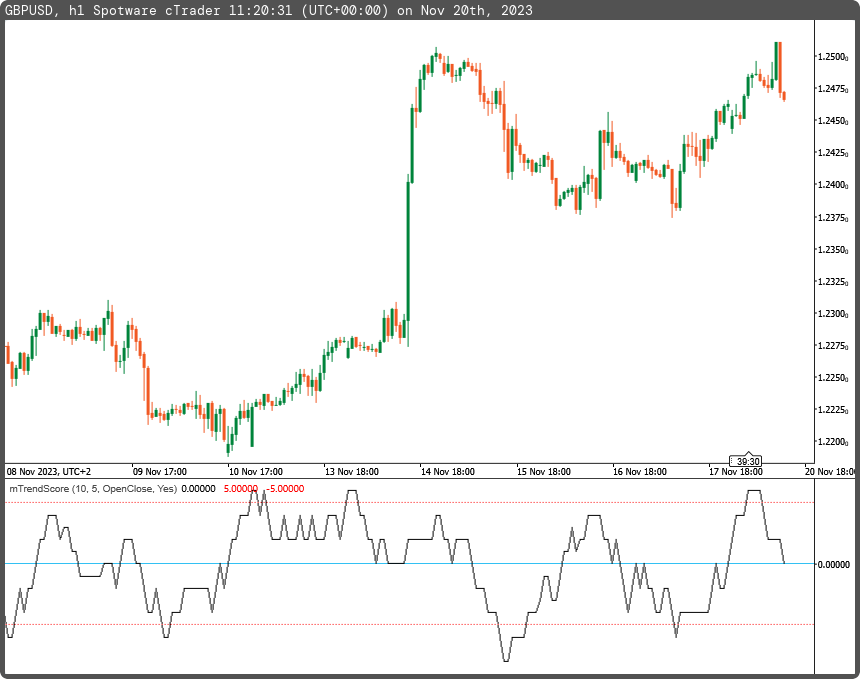
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Levels(0)]
[Indicator(IsOverlay = false, AccessRights = AccessRights.None)]
public class mTrendScore : Indicator
{
[Parameter("Period (10)", DefaultValue = 10)]
public int inpPeriod { get; set; }
[Parameter("OverboughtOversold value (5)", DefaultValue = 5)]
public double inpOverboughtOversold { get; set; }
[Parameter("Price Method (close)", DefaultValue = enumPriceMethods.OpenClose)]
public enumPriceMethods inpPriceMethod { get; set; }
[Parameter("Use Period (yes)", DefaultValue = true)]
public bool inpUsePeriod { get; set; }
[Output("TrendScore", LineColor = "Black", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outTrendScore { get; set; }
[Output("Overbought", LineColor = "Red", PlotType = PlotType.Line, LineStyle = LineStyle.Dots, Thickness = 1)]
public IndicatorDataSeries outOverbought { get; set; }
[Output("Oversold", LineColor = "Red", PlotType = PlotType.Line, LineStyle = LineStyle.Dots, Thickness = 1)]
public IndicatorDataSeries outOversold { get; set; }
private IndicatorDataSeries _score, _trendscore, _prevprice;
protected override void Initialize()
{
_score = CreateDataSeries();
_trendscore = CreateDataSeries();
_prevprice = CreateDataSeries();
}
public override void Calculate(int i)
{
_prevprice[i] = inpPriceMethod == enumPriceMethods.OpenClose ? Bars.OpenPrices[i] : (i>1 ? Bars.ClosePrices[i-1] : Bars.TypicalPrices[i]);
_score[i] = Bars.ClosePrices[i] > _prevprice[i] ? +1 : Bars.ClosePrices[i] < _prevprice[i] ? -1 : 0;
if(inpUsePeriod == true)
_trendscore[i] = _score.Sum(inpPeriod);
else
{
if(Bars.ClosePrices[i] > _prevprice[i])
_trendscore[i] = (_trendscore[i-1] >= 0 && i>1 ? _trendscore[i-1] + 1 : 1);
else
if(Bars.ClosePrices[i] < _prevprice[i])
_trendscore[i] = (_trendscore[i-1] < 0 && i>1 ? _trendscore[i-1] - 1 : -1);
else
_trendscore[i] = i>1 ? _trendscore[i-1] : 0;
}
outTrendScore[i] = _trendscore[i];
outOverbought[i+100] = inpOverboughtOversold;
outOversold[i+100] = -inpOverboughtOversold;
}
}
public enum enumPriceMethods
{
OpenClose,
CloseClose
}
}
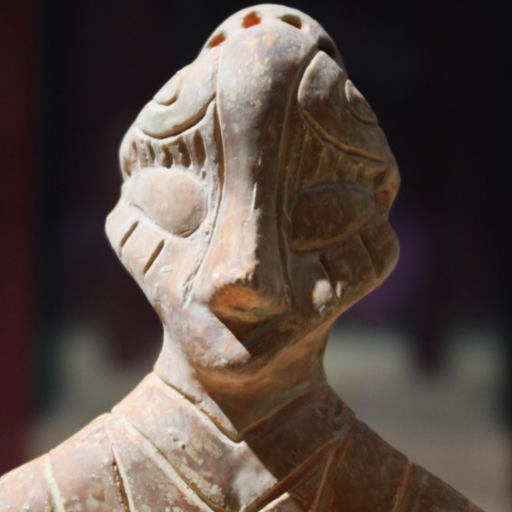
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mTrendScore.algo
- Rating: 5
- Installs: 272
- Modified: 20/11/2023 11:22
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.