Description
The Relative Volatility Index (RVI) is similar to the Relative Strength Index (RSI) index. Both measure the direction of volatility, but RVI uses the standard deviation of price changes in its calculations, while RSI uses the absolute price changes. The RVI is best used as a confirmation indicator to other momentum and/or trend-following indicators.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Cloud("outRVI0", "outRVI20", FirstColor = "Red", SecondColor = "Red", Opacity = 0.1)]
[Cloud("outRVI80", "outRVI100", FirstColor = "Green", SecondColor = "Green", Opacity = 0.1)]
[Levels(20, 80, 50)]
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class RelativeVolatilityIndex : Indicator
{
[Parameter("Data Source (close)", DefaultValue = PriceTypes.Close)]
public PriceTypes inpPriceType { get; set; }
[Parameter("RVI Periods (10)", DefaultValue = 10)]
public int inpPeriods { get; set; }
[Parameter("MA Type", DefaultValue = MovingAverageType.Exponential)]
public MovingAverageType inpSmoothMaType { get; set; }
[Output("RelativeVolatilityIndex", LineColor = "Black", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outRVI { get; set; }
[Output("outRVI0", LineColor = "Transparent", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outRVI0 { get; set; }
[Output("outRVI20", LineColor = "Transparent", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outRVI20 { get; set; }
[Output("outRVI80", LineColor = "Transparent", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outRVI80 { get; set; }
[Output("outRVI100", LineColor = "Transparent", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries outRVI100 { get; set; }
private IndicatorDataSeries _rvi;
private IndicatorDataSeries _price;
private IndicatorDataSeries _fir;
private IndicatorDataSeries _changeup;
private IndicatorDataSeries _changedn;
private StandardDeviation _stddev;
private MovingAverage _emaup;
private MovingAverage _emadn;
protected override void Initialize()
{
_rvi = CreateDataSeries();
_price = CreateDataSeries();
_fir = CreateDataSeries();
_changeup = CreateDataSeries();
_changedn = CreateDataSeries();
_stddev = Indicators.StandardDeviation(_price, inpPeriods, inpSmoothMaType);
_emaup = Indicators.MovingAverage(_changeup, inpPeriods, inpSmoothMaType);
_emadn = Indicators.MovingAverage(_changedn, inpPeriods, inpSmoothMaType);
}
public override void Calculate(int i)
{
if (i <= inpPeriods)
{
_price[i] = _fir[i] = Bars.ClosePrices[i];
_rvi[i] = 50;
outRVI[i] = 50;
return;
}
_fir[i] = (Bars.ClosePrices[i] + 2.0 * Bars.ClosePrices[i - 1] + 2.0 * Bars.ClosePrices[i - 2] + Bars.ClosePrices[i - 3]) / 6.0;
switch (inpPriceType)
{
case PriceTypes.Open:
_price[i] = Bars.OpenPrices[i];
break;
case PriceTypes.Close:
_price[i] = Bars.ClosePrices[i];
break;
case PriceTypes.High:
_price[i] = Bars.HighPrices[i];
break;
case PriceTypes.Low:
_price[i] = Bars.LowPrices[i];
break;
case PriceTypes.Median:
_price[i] = Bars.MedianPrices[i];
break;
case PriceTypes.Typical:
_price[i] = Bars.TypicalPrices[i];
break;
case PriceTypes.Weighted:
_price[i] = Bars.WeightedPrices[i];
break;
case PriceTypes.FIR:
_price[i] = _fir[i];
break;
default:
_price[i] = Bars.ClosePrices[i];
break;
}
_changeup[i] = (_price[i] - _price[i - 1] <= 0 ? 0 : _stddev.Result[i]);
_changedn[i] = (_price[i] - _price[i - 1] > 0 ? 0 : _stddev.Result[i]);
//outRVI[i] = _emaup.Result[i] / (_emaup.Result[i] + _emadn.Result[i]) * 100;
_rvi[i] = (_emaup.Result[i] + _emadn.Result[i] != 0.0 ? (_emaup.Result[i] / (_emaup.Result[i] + _emadn.Result[i]) * 100) : _rvi[i - 1]);
outRVI[i] = _rvi[i];
outRVI0[i] = 0.0;
outRVI20[i] = 20.0;
outRVI80[i] = 80.0;
outRVI100[i] = 100.0;
}
}
public enum PriceTypes
{
Open,
Close,
High,
Low,
Median,
Typical,
Weighted,
FIR
}
}
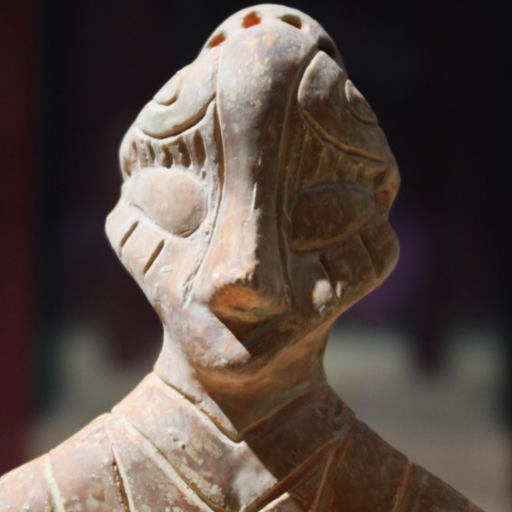
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mRelativeVolatilityIndex.algo
- Rating: 5
- Installs: 995
- Modified: 08/09/2022 19:36
Comments
When used in conjunction with other momentum and/or trend-following indicators, the RVI is most effective when utilized as a confirmation indication.
Still trying to figure out what you are trying to explain but I would like to recommend that 247examhelp is offering the best Take My Exam Service that can be best for the students as they have the best academic consultants.
Why I can´t see the EMA?