Description
Akin to the Stochastic Oscillator, the Stochastic RSI is a technical indicator which oscillates according to change in the RSI (rather than price). It works by taking a moving average of the cumulative changes in the RSI over a given time period. The K% line is a series of values calculated by the formula:
100 x (current close - max. of RSI in given period) / (max. - min. of RSI in given period).
The D% line is a moving average over a given period of the K% line, which results in a smoother, less sensitive curve. A popular strategy is trading the crosses between the K% and D% lines according to the current market sentiment.
This is slightly different to qualitiedx2's versions (#1 and #2) of the Stochastic RSI, which are unfamiliar to me - please do let me know if I've given this an incorrect title. My implementation is similar to that described here and here (like the one on TradingView's platform).
Changelog:
27/08/2022 - added missing getters and setters to attributes.
28/08/2022 - removed deprecated 'PersentsK' and 'PersentsD' and implementation of the StochasticOscillator interface. Added cloud. Fixed code style. Added indicator attributes.
For scalping on very short timeframes (<M5), it is recommended to use a Stochastic Length of '8'.
Chartshots:
AUDUSD, M1: Settings (3, 3, 14, 8, MovingAverageType.Simple)
AUDUSD, M5: Settings (3, 3, 14, 14, MovingAverageType.Simple)
using cAlgo.API;
using cAlgo.API.Indicators;
namespace cAlgo
{
[Levels(0, 50, 100)]
[Cloud("%K", "%D", FirstColor = "Aquamarine", Opacity = 0.09, SecondColor = "Tomato")]
[Indicator(IsOverlay = false, AccessRights = AccessRights.None)]
public class StochasticRSI : Indicator {
// Indicators
private MovingAverage smoothedK { get; set; }
private MovingAverage smoothedD { get; set; }
private RelativeStrengthIndex rsi{ get; set; }
// Series for k to be updated in Calculate()
private IndicatorDataSeries kSeries { get; set; }
// Parameters
[Parameter("K Periods", DefaultValue = 3, MinValue = 1)]
public int KPeriods { get; set; }
[Parameter("D Periods", DefaultValue = 3, MinValue = 1)]
public int DPeriods { get; set; }
[Parameter("RSI Length", DefaultValue = 14, MinValue = 1)]
public int RSILength { get; set; }
//* Change to 8 for M1 scalping *//
[Parameter("Stochastic Length", DefaultValue = 14, MinValue = 1)]
public int StochLength { get; set; }
[Parameter("MA Type", DefaultValue = MovingAverageType.Simple)]
public MovingAverageType MAType { get; set; }
// Output plots
[Output("%K", Color = Colors.Aquamarine, LineStyle = LineStyle.Solid)]
public IndicatorDataSeries PercentK { get; set; }
[Output("%D", Color = Colors.Tomato, LineStyle = LineStyle.Solid)]
public IndicatorDataSeries PercentD { get; set; }
protected override void Initialize() {
rsi = Indicators.RelativeStrengthIndex(MarketSeries.Close, RSILength);
kSeries = CreateDataSeries();
}
public override void Calculate(int index) {
var lowestLow = rsi.Result.Minimum(KPeriods);
var highestHigh = rsi.Result.Maximum(KPeriods);
var currentClose = rsi.Result[index];
var kVal = 100 * (currentClose - lowestLow) / (highestHigh - lowestLow);
kSeries[index] = kVal;
smoothedK = Indicators.MovingAverage(kSeries, KPeriods, MAType);
PercentK[index] = smoothedK.Result[index];
smoothedD = Indicators.MovingAverage(PercentK, DPeriods, MAType);
PercentD[index] = smoothedD.Result[index];
}
}
}
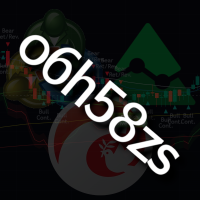
o6h58zs
Joined on 17.07.2022 Blocked
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Stochastic RSI.algo
- Rating: 0
- Installs: 1298
- Modified: 28/08/2022 12:55