Description
Paginator Tool for cTrader 4.2+
Parameters
How to use: just add it as a regular indicator.
Feel free to make your suggestions to improve this indicator!
using System;
using cAlgo.API;
namespace devman
{
[Indicator(AccessRights = AccessRights.None, IsOverlay = true)]
public class Paginator : Indicator
{
[Parameter(DefaultValue = VerticalAlignment.Bottom)]
public VerticalAlignment VerticalAlignment { get; set; }
[Parameter(DefaultValue = HorizontalAlignment.Center)]
public HorizontalAlignment HorizontalAlignment { get; set; }
[Parameter(DefaultValue = 11)]
public int FontSize { get; set; }
private DateTime ActualOpenTime => Bars[Chart.FirstVisibleBarIndex].OpenTime;
protected override void Initialize()
{
Chart.AddControl(CreateNavigationPanel());
}
public override void Calculate(int index)
{
// DO NOTHING
}
private StackPanel CreateNavigationPanel()
{
var panel = new StackPanel
{
VerticalAlignment = VerticalAlignment,
HorizontalAlignment = HorizontalAlignment,
Orientation = Orientation.Horizontal,
Margin = 10,
};
var navBtnStyle = new Style(DefaultStyles.ButtonStyle);
navBtnStyle.Set(ControlProperty.Margin, new Thickness(1, 0, 1, 0));
navBtnStyle.Set(ControlProperty.FontSize, FontSize);
var b0 = new Button { Text = "◀◀", Style = navBtnStyle };
b0.Click += _ => Chart.ScrollXTo(0);
var bY = new Button { Text = "◀◀ Y", Style = navBtnStyle };
bY.Click += _ => NavigateTo(ActualOpenTime.AddYears(-1));
var bM = new Button { Text = "◀◀ M", Style = navBtnStyle };
bM.Click += _ => NavigateTo(ActualOpenTime.AddMonths(-1));
var bD = new Button { Text = "◀ D", Style = navBtnStyle };
bD.Click += _ => NavigateTo(ActualOpenTime.AddDays(-1));
var fD = new Button { Text = "D ▶", Style = navBtnStyle };
fD.Click += _ => NavigateTo(ActualOpenTime.AddDays(1));
var fM = new Button { Text = "M ▶▶", Style = navBtnStyle };
fM.Click += _ => NavigateTo(ActualOpenTime.AddMonths(1));
var fY = new Button { Text = "Y ▶▶", Style = navBtnStyle };
fY.Click += _ => NavigateTo(ActualOpenTime.AddYears(1));
var f0 = new Button { Text = "▶▶", Style = navBtnStyle };
f0.Click += _ => Chart.ScrollXTo(Bars.Count);
panel.AddChild(b0);
panel.AddChild(bY);
panel.AddChild(bM);
panel.AddChild(bD);
panel.AddChild(fD);
panel.AddChild(fM);
panel.AddChild(fY);
panel.AddChild(f0);
return panel;
}
private void NavigateTo(DateTime time)
{
var indexByTime = Bars.OpenTimes.GetIndexByTime(time);
if (indexByTime < 0)
indexByTime = 0;
Chart.ScrollXTo(indexByTime);
}
}
}
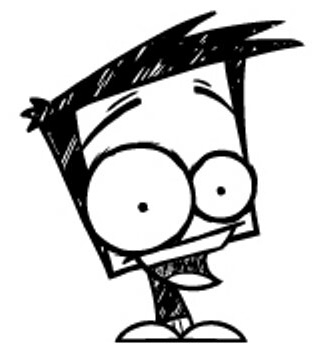
devman
Joined on 22.10.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Paginator.algo
- Rating: 5
- Installs: 913
- Modified: 21/07/2022 13:59
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
This device is a portable, mechanical, and electric device that helps you quickly turn the pages of a book without the need to move your hands. It is light and easy to carry, so you can bring it with you anywhere. It's perfect for when you're reading a book in bed and listing images amazon. It also has a built-in magnifier that allows you to read small print without having to strain your eyes. This device is also useful when you're cooking and need to read a recipe without dirtying your hands.