Description
close position in specefic equity.
it's useful for someone that couldn't control himself in live market.
support us for more free indicator and bot by sign up in LiteFinance broker from this link
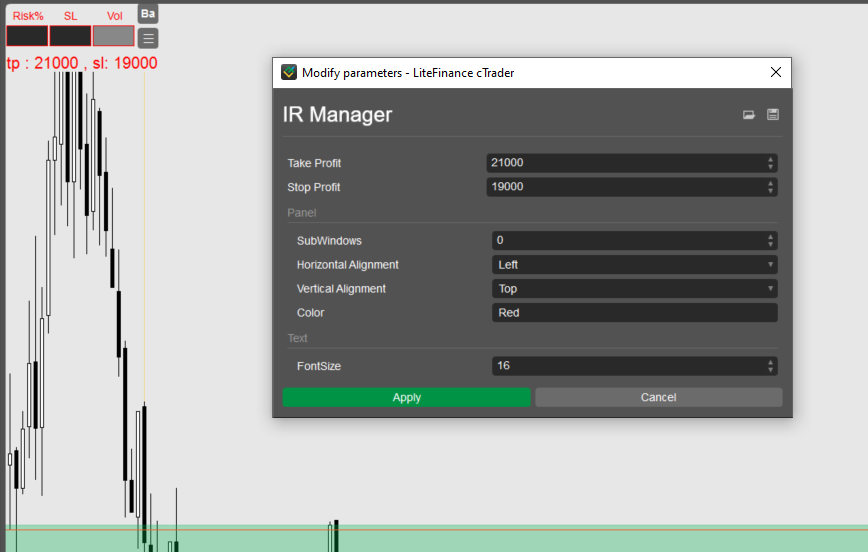
using System;
using System.Linq;
using cAlgo.API;
namespace cAlgo
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class IRManager : Robot
{
private TextBlock tb = new TextBlock();
[Parameter("Take Profit", DefaultValue = 100)]
public double tp { get; set; }
[Parameter("Stop Profit", DefaultValue = 90)]
public double sl { get; set; }
[Parameter("SubWindows", DefaultValue = 0, MinValue = 0, Group = "Panel")]
public int SubWindows { get; set; }
[Parameter("Horizontal Alignment ", DefaultValue = HorizontalAlignment.Left, Group = "Panel")]
public HorizontalAlignment HAlignment { get; set; }
[Parameter("Vertical Alignment", DefaultValue = VerticalAlignment.Top, Group = "Panel")]
public VerticalAlignment VAlignment { get; set; }
[Parameter("Color", DefaultValue = "Red", Group = "Panel")]
public string color { get; set; }
[Parameter("FontSize", DefaultValue = 16, Group = "Text")]
public int FontSize { get; set; }
private static bool Hide, ModeBE;
private static double risk, pip;
private StackPanel[] stackpanel = new StackPanel[4];
private TextBox[] textbox = new TextBox[3];
private Button[] button = new Button[2];
protected override void OnStart()
{
var MainPanel = new StackPanel
{
HorizontalAlignment = HAlignment,
VerticalAlignment = VAlignment,
BackgroundColor = Chart.ColorSettings.BackgroundColor,
Orientation = Orientation.Vertical
};
var panel = new StackPanel
{
HorizontalAlignment = HAlignment,
VerticalAlignment = VAlignment,
BackgroundColor = Chart.ColorSettings.BackgroundColor,
Orientation = Orientation.Horizontal
};
TextBlock[] textblock = new TextBlock[3];
string[] Name = new string[3]
{
"Risk%",
"SL",
"Vol"
};
for (int i = 0; i < 4; i++)
{
stackpanel[i] = new StackPanel
{
HorizontalAlignment = HorizontalAlignment.Center,
VerticalAlignment = VerticalAlignment.Center,
Orientation = Orientation.Vertical
};
if (i < 3)
{
textblock[i] = new TextBlock
{
ForegroundColor = color,
Margin = "0 0 0 3",
HorizontalAlignment = HorizontalAlignment.Center,
Text = Name[i]
};
textbox[i] = new TextBox
{
ForegroundColor = color,
Margin = "0 0 2 -1",
HorizontalAlignment = HorizontalAlignment.Center,
Width = 42,
BorderThickness = 1,
BorderColor = color,
TextAlignment = TextAlignment.Center
};
stackpanel[i].AddChild(textblock[i]);
stackpanel[i].AddChild(textbox[i]);
}
else
{
for (int j = 0; j < 2; j++)
{
button[j] = new Button
{
Width = 21,
Height = 21,
VerticalAlignment = VAlignment,
HorizontalAlignment = HAlignment,
FontWeight = FontWeight.Bold,
Margin = "2 0 4 4",
Padding = 1
};
stackpanel[i].AddChild(button[j]);
}
}
panel.AddChild(stackpanel[i]);
}
var txtblock = new TextBlock();
txtblock.Text = "tp : " + tp + " , sl: " + sl;
txtblock.ForegroundColor = color;
txtblock.FontSize = FontSize;
MainPanel.AddChild(panel);
MainPanel.AddChild(txtblock);
textbox[0].Text = risk == 0 ? "" : risk.ToString();
textbox[1].Text = pip == 0 ? "" : pip.ToString();
textbox[2].IsEnabled = false;
textbox[0].TextChanged += TextBox1_TextChanged;
textbox[1].TextChanged += TextBox2_TextChanged;
button[0].Text = ModeBE == false ? "Ba" : "Eq";
button[0].FontSize = 11;
button[1].Text = "☰";
button[1].FontSize = 13;
button[0].Click += ButtonBlance_Click;
button[1].Click += ButtonHide_Click;
if (SubWindows > Chart.IndicatorAreas.Count)
SubWindows = Chart.IndicatorAreas.Count;
if (SubWindows > 0)
Chart.IndicatorAreas[SubWindows - 1].AddControl(MainPanel);
else
Chart.AddControl(MainPanel);
if (Hide == true)
ModeHide();
}
private void ModeHide()
{
if (Hide == false)
{
stackpanel[0].IsVisible = true;
stackpanel[1].IsVisible = true;
stackpanel[2].IsVisible = true;
button[0].IsVisible = true;
}
else
{
stackpanel[0].IsVisible = false;
stackpanel[1].IsVisible = false;
stackpanel[2].IsVisible = false;
button[0].IsVisible = false;
}
}
protected override void OnTick()
{
//if (IsLastBar && Hide == false)}
// textbox[2].Text = CalcVolume();}
tb.Text = "tp : " + tp.ToString() + ", sl : " + sl.ToString();
foreach (var position in Positions)
{
if (Account.Equity > tp || Account.Equity < sl)
{
ClosePositionAsync(position);
}
}
foreach (var order in PendingOrders)
{
if (Account.Equity > tp || Account.Equity < sl)
{
CancelPendingOrder(order);
}
}
}
private void ButtonHide_Click(ButtonClickEventArgs obj)
{
Hide = Hide ? false : true;
ModeHide();
}
private void ButtonBlance_Click(ButtonClickEventArgs obj)
{
ModeBE = ModeBE ? false : true;
button[0].Text = ModeBE == false ? "Ba" : "Eq";
textbox[2].Text = CalcVolume();
}
private void TextBox1_TextChanged(TextChangedEventArgs obj)
{
risk = obj.TextBox.Text == "" ? 0 : Convert.ToDouble(obj.TextBox.Text);
textbox[2].Text = CalcVolume();
}
private void TextBox2_TextChanged(TextChangedEventArgs obj)
{
pip = obj.TextBox.Text == "" ? 0 : Convert.ToDouble(obj.TextBox.Text);
textbox[2].Text = CalcVolume();
}
private string CalcVolume()
{
var Money = ModeBE == false ? Account.Balance : Account.Equity;
return Math.Round(Symbol.VolumeInUnitsToQuantity(Money * risk / 100 / (pip * Symbol.PipValue)), 2).ToString();
}
}
}
IRCtrader
Joined on 17.06.2021
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: IR Manager.algo
- Rating: 5
- Installs: 209
- Modified: 17/08/2024 18:58
Comments
I always get what I need here. I am learning from free code camp but there are still tasks that can't be solved with external help. IE CV Writing Service is one of my coding websites. Sometimes I also like to experiment with the new codes as it makes me excited to learn.
Good post. I've been checking this blog frequently, and I'm impressed! Very helpful stuff, especially the last part I like this kind of information a lot. For a while I was looking for this particular information. Many thanks and best of luck. wordle today
Wordle Today is an online word game that challenges players to guess a five-letter word in six tries. Each time a guess is made, the game provides feedback by highlighting letters that are correctly placed in green and letters that are in the word but in the wrong position in yellow. Players must use these clues to guess the word within the allotted six tries.