Description
This robot is based on the martingale strategy.
This code is a cAlgo API sample.
The "Sample Martingale Robot" creates a random Sell or Buy order. If the Stop loss is hit, a new order of the same type (Buy / Sell) is created with double the Initial Volume amount. The robot will continue to double the volume amount for all orders created until one of them hits the take Profit. After a Take Profit is hit, a new random Buy or Sell order is created with the Initial Volume amount.
/
alexk
Joined on 30.09.2011
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Martingale Robot.algo
- Rating: 3
- Installs: 18338
- Modified: 13/10/2021 09:54
Comments
Hi. Please help me provide code in this cBot to change SL/TP values to system current ATR, each time a new trade is generated.
Thanks.
Hi Guys
What part of the original code do I have to change where by this bot will only go either long or short depending on what I change it to??
I do not want it to go random each time just only 1 way at a time depending on what I set it to.
Thanks
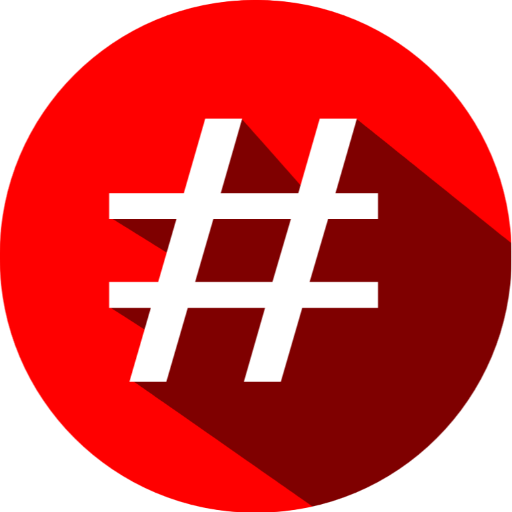
A strategy like this scalper found here Adrenaline with ctrader.guru technology inside, this week special offer, enjoy!
Hi,
could you pls make cbot like this:
price close abvove ema20 -->buy position 1. if price go down 20pip, buy position 2, go down 40pips buy 3....., TP if profit > 50u. and the volume for position 1,2,3 is 0.1, 0.2, 0.3
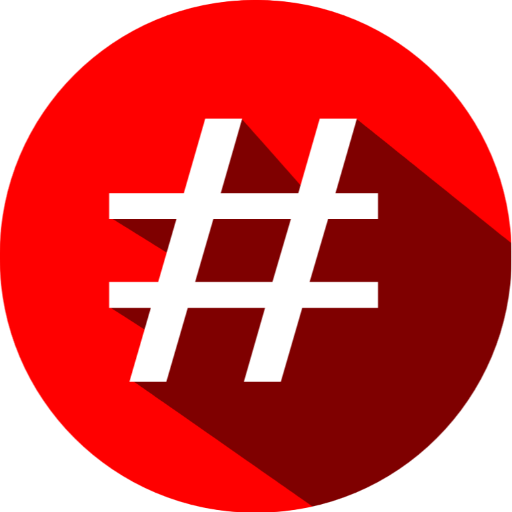
Add To Reverse on loss trade, good for trend following bad for lateral
// -------------------------------------------------------------------------------------------------
//
// This code is a cAlgo API sample.
//
// This robot is intended to be used as a sample and does not guarantee any particular outcome or
// profit of any kind. Use it at your own risk
//
// All changes to this file will be lost on next application start.
// If you are going to modify this file please make a copy using the "Duplicate" command.
//
// The "Sample Martingale Robot" creates a random Sell or Buy order. If the Stop loss is hit, a new
// order of the same type (Buy / Sell) is created with double the Initial Volume amount. The robot will
// continue to double the volume amount for all orders created until one of them hits the take Profit.
// After a Take Profit is hit, a new random Buy or Sell order is created with the Initial Volume amount.
//
// -------------------------------------------------------------------------------------------------
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class MartingaleRobot : Robot
{
[Parameter("Initial Volume", DefaultValue = 1000, MinValue = 0)]
public int InitialVolume { get; set; }
[Parameter("Stop Loss", DefaultValue = 40)]
public int StopLoss { get; set; }
[Parameter("Take Profit", DefaultValue = 60)]
public int TakeProfit { get; set; }
[Parameter("To Reverse?", DefaultValue = true)]
public bool ToReverse { get; set; }
private Random random = new Random();
protected override void OnStart()
{
Positions.Closed += OnPositionsClosed;
ExecuteOrder(InitialVolume, GetRandomTradeType());
}
private void ExecuteOrder(long volume, TradeType tradeType)
{
var result = ExecuteMarketOrder(tradeType, Symbol, volume, "Martingale", StopLoss, TakeProfit);
if (result.Error == ErrorCode.NoMoney)
Stop();
}
private void OnPositionsClosed(PositionClosedEventArgs args)
{
Print("Closed");
var position = args.Position;
if (position.Label != "Martingale" || position.SymbolCode != Symbol.Code)
return;
if (position.NetProfit > 0)
{
ExecuteOrder(InitialVolume, position.TradeType);
}
else
{
TradeType reversed = (position.TradeType == TradeType.Sell) ? TradeType.Buy : TradeType.Sell;
ExecuteOrder((int)position.Volume * 2, (ToReverse) ? reversed : position.TradeType);
}
}
private TradeType GetRandomTradeType()
{
return random.Next(2) == 0 ? TradeType.Buy : TradeType.Sell;
}
}
}
It would be great if you put a moving average to open favorable transactions, that way it would be less risky to bust the account.
Hi, C Team,
Happy new year 2019!. I want to set up this robot to take small profits all day long with many or hundereds of trades though out the day.
When USD/EUR Closes it automatically switches to Asian markets during night trading. I want to set a automatic system with 5G connectivity to carry out my system night and day.
Kindly let me know the best way to execute it, I have all the hardwares and 5G set just need the C robots tuned to this mission.
Kind Regards
John
@amantalpur007@gmail.com you can check my code if you want to have a max number of loss in a row
https://github.com/martinfou/cAlgo/blob/master/Sources/Robots/MartingaleLimit/MartingaleLimit/MartingaleLimit.cs
[@Rog2548] and @[bhagya3udana856]
You have to round to the nearest 1000. you can BUY/SELL 1000, 2000 but not 1500
(int)(1000*Math.Floor(Position.Volume*2.5/1000))
Martingale is just an empirical method. This has nothing to do with the finance theory and science. If you want a real cBot you must find a solution for stop opening positions when the market is in range positions. This can do just if you know financial mathematics. A good example is to use just 3 indicators RSI, Simple Moving Average and Bollinger Band combined with financial mathematics. I make this with a 90% winning of trades. You don't need a lot of entries, just the good and surely entries.

this bot shows false profit because of BUG in: OnPositionsClosed
when you start new trade in OnPositionsClosed backtest just give you false win trade
thats if you use the: m1 bars from servers
in backtest settings
use: tick data from server and you will see a better results not false one from m1 bars
and the results are scary not 1k to milion but milion to zero
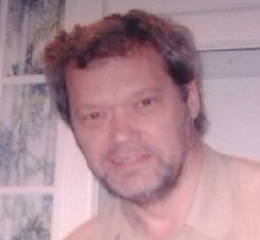
I love Martingales, in Backtesting, but not when they eventually trash my Real account, even a tiny one! However overall I have made money, just not as easily as I had hoped, but it is worth exploting, I think?
I also persevere with them, trying to get one of the many variants just right. Thanks for sharing this! ;)
Try this: 5 / 2 instead of 2.5
ExecuteOrder((int)position.Volume * 5 / 2, position.TradeType);
I want to change the Posision multiplying factor from 2 to 2.5. I don't know programming but i changed the 2 in to 2.5 in calgo and compiled after i got two error massage
1. best overloaded method match for calgo .robot.martingale robot
2. Cannot convert from double to long
Please tell me how to do that
Amazing results, but don't really like the random trades. Does anyone have a version to al least take long trades on uptrends, say combined with a MA and short trades on a downtrend?...
Click, care to share your version?
Thanks
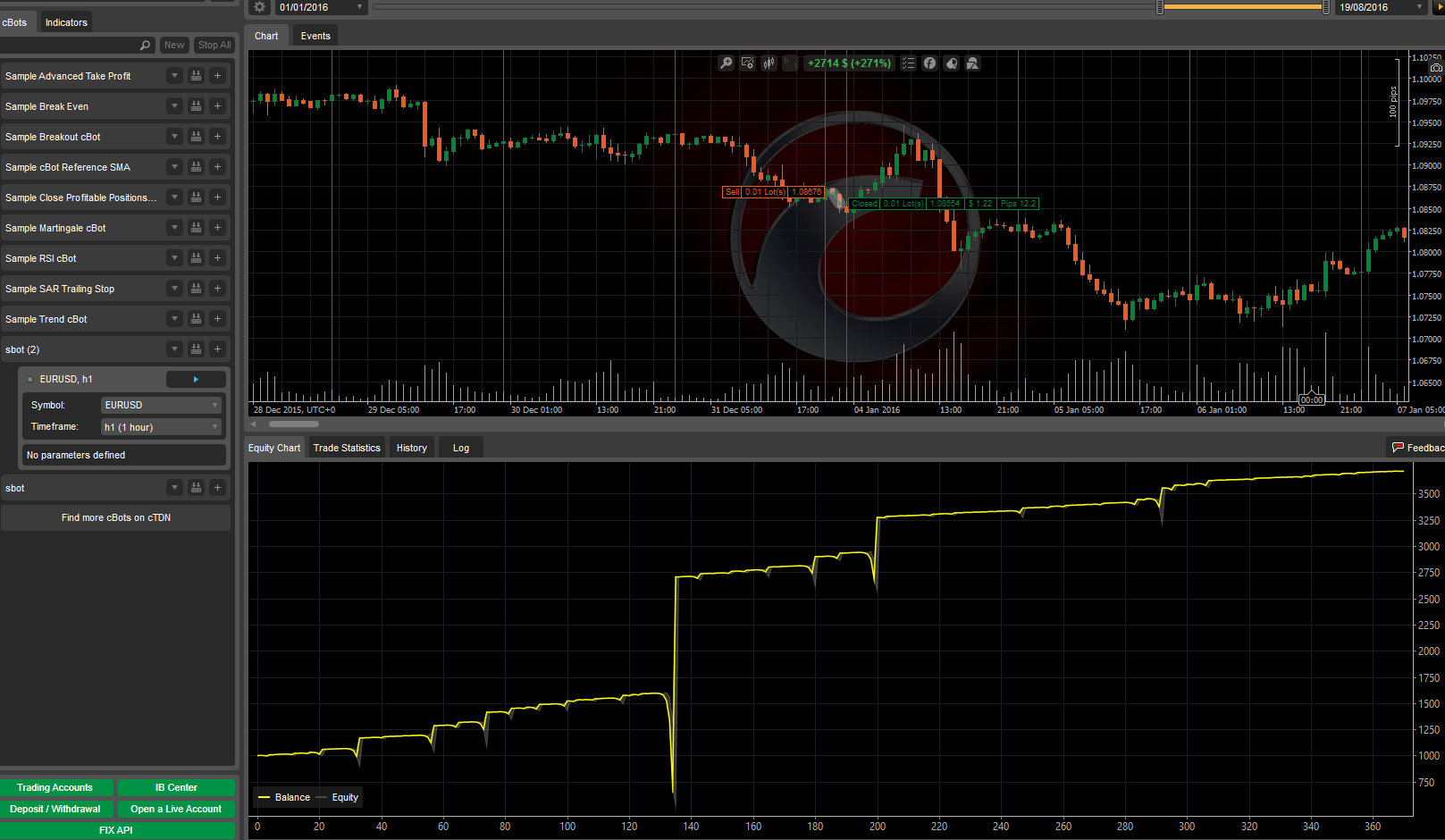
Like this bot/strategy, but probably too risky to use a basic version. Trailing stop on this bot is not a good idea I think, since the point is that if one trade goes with loss, the next one will make you go with profit by doubling the volume and so on... So it will be much like gambling :) Made another version of it and backtested it with profit from december 2012 to august 2016 on tick data with commission 30 (EURUSD in demo account starting capital 5000 USD) :) Same with 1000 USD january 2016 to august 2016 :) Still trying to make it work on other currencies with low starting capital though... :D
please add trailing stop - after many attempts of backtest some are amazing some are really crap and result in a loss
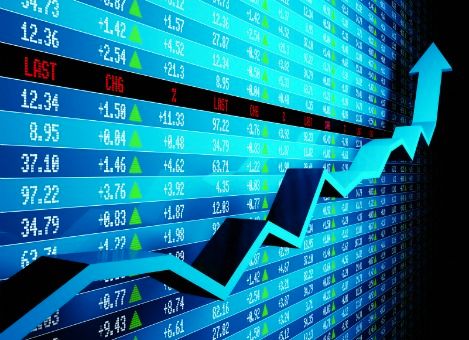
I am just starting out backtesting this one >>> very promising! <<<
Short term scalpers will love that one
I Have angrybird scalping robot converted from MT4 ea, not able to publish here due to some technical issues
similer to this ea check result here. https://www.myfxbook.com/portfolio/fxwinner/1454935 i will try this eawhen reach 1000 $
Some " for dummies" to installl from github to Calgo..?
have clone from github to visual studio..then my knowledge ended:/
conny
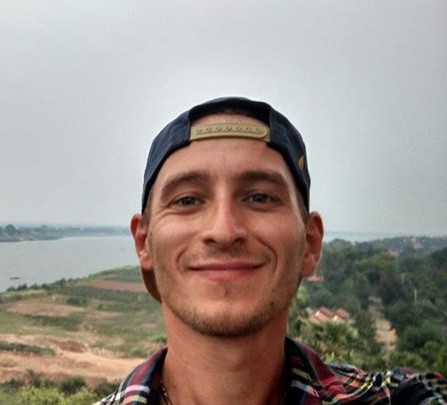
In backtesting this robot works good but in live test sometimes the robot close a trade and somehow doesent open a new one so i need to stop the bot and start it from new.
could you manage to add "trailing stop" to this bot?
astoundingly good results (to my opinion) with GPDJPY m20, Initial Volume 10000, Stop Loss and Take Profit 40 40, Starting Balance USD 2000 (on a DEMO Spotware cAlgo). Net Profit starting from 02/03/2015 to 27/03/2015 USD 124,53 means about 6% in less than a month.
And that bot is free! What do you think?
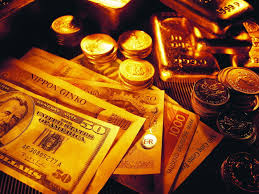
can anyone put trailling stop in this bot?
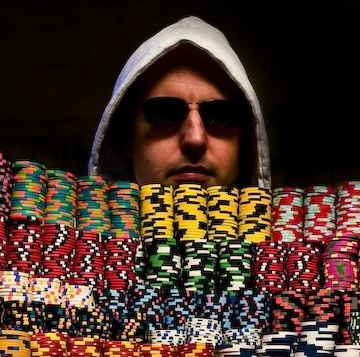
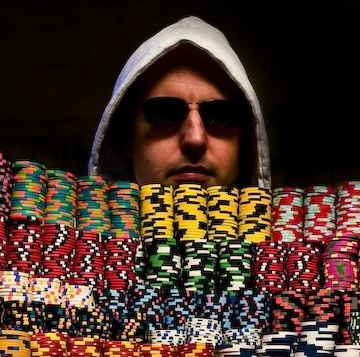
I made a backtest on...
- EURCHF M5,
- 2014 + jan15. 10000 eur
- Net profit: 7054 eur.
- Balance DD 30%.
Straight line up. I like it.
But how to upload pictures here??
Can you the martingale simultineously buy only instead of random?