Description
Instead of using the popular MA periods like 50, 100, 200, or Fibonacci numbers, I prefer to use the actual periods of the daily, weekly, monthly or even yearly to get its average price. It's just making more sense for me.
For example, I want to know what the H4 price is doing compare to its monthly average price. So I will use 120 periods on my MA, assuming that 120 bars on H4 chart equals to 1 bar of monthly chart. For weekly average price, I will use that 120 periods on H1 chart, and to get daily average price, I use 96 periods on 15 minutes chart.
However it's not that practical if I switch to lower timeframe but still want to keep looking at the monthly average price for example, since I have to change the periods to 480 on H1 chart, and 1920 on 15 mins chart. Though it can be saved as a template, I don't think that large number really represents the actual average price.
This indicator tries to solve this issue. Not only not to always change the periods when switching between timeframes, it also keeps the average price value of the selected timeframe.
The indicator also lets you clearly see the trend. If the close average line is above the open line, you are on an uptrend, and vise versa. The distance between open, close, and median lines also signs the healthy of the trend.
On exhausting trend, sideways, sometimes pullbacks, the close and open lines will be closer to each other.
The crossing of the close and open lines can be assumed as a trend reversal (you have to also confirm it by checking the price action).
Please note that if you are using the non smoothing line, the last bar of the selected timeframe is still running and not closed yet, so you will see the high, low, close, and median lines of the last periods are changing especially on minutes timeframe.
Features:
- Multi timeframes
- Available MAs: Simple, Exponential, Hull, Weighted, Wilder Smoothing, Time Series, Triangular, Vidya
- Smoothing and non smoothing lines
Cheers.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = true, AccessRights = AccessRights.None, TimeZone = TimeZones.NorthAsiaStandardTime, AutoRescale = false)]
[Cloud("High", "Low", Opacity = 0.075)]
public class MultiTimeframeMovingAverage : Indicator
{
private Bars _Series;
private int _TimeframeIndex;
private MovingAverage _MaHigh;
private MovingAverage _MaLow;
private MovingAverage _MaClose;
private MovingAverage _MaOpen;
private MovingAverage _MaMedian;
private string _Text;
[Parameter("Type", DefaultValue = MovingAverageType.Exponential)]
public MovingAverageType _MaType { get; set; }
[Parameter("Periods", DefaultValue = 20)]
public int _Periods { get; set; }
[Parameter("Timeframe", DefaultValue = "Daily")]
public TimeFrame _Timeframe { get; set; }
[Parameter("Smoothing", DefaultValue = _Smoothing.Yes)]
public _Smoothing _SmoothLine { get; set; }
public enum _Smoothing
{
Yes,
No
}
[Parameter("Info", DefaultValue = true)]
public bool _ShowInfo { get; set; }
[Parameter("Notes", DefaultValue = "")]
public string _Notes { get; set; }
[Output("Open", LineColor = "#FF02AFF1", LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries _Open { get; set; }
[Output("Close", LineColor = "#FFFF00C5")]
public IndicatorDataSeries _Close { get; set; }
[Output("High", LineColor = "#FFB3B3B3")]
public IndicatorDataSeries _High { get; set; }
[Output("Low", LineColor = "#FFB3B3B3")]
public IndicatorDataSeries _Low { get; set; }
[Output("Median", LineColor = "#FF595959", LineStyle = LineStyle.Lines, Thickness = 1)]
public IndicatorDataSeries _Median { get; set; }
protected override void Initialize()
{
_Series = MarketData.GetBars(_Timeframe);
_MaOpen = Indicators.MovingAverage(_Series.OpenPrices, _Periods, _MaType);
_MaClose = Indicators.MovingAverage(_Series.ClosePrices, _Periods, _MaType);
_MaHigh = Indicators.MovingAverage(_Series.HighPrices, _Periods, _MaType);
_MaLow = Indicators.MovingAverage(_Series.LowPrices, _Periods, _MaType);
_MaMedian = Indicators.MovingAverage(_Series.MedianPrices, _Periods, _MaType);
if (_ShowInfo)
{
//MA
_Text = "MA: " + Convert.ToString(_MaType);
_Text += "\nTimeframe: " + Convert.ToString(_Timeframe);
_Text += "\nPeriods: " + Convert.ToString(Convert.ToInt32(this._Periods));
//Notes
if (!string.IsNullOrEmpty(_Notes))
{
_Text += "\nNotes: " + _Notes;
}
//Print
Chart.DrawStaticText("Info", _Text, VerticalAlignment.Top, HorizontalAlignment.Left, Color.White);
}
}
public override void Calculate(int index)
{
switch (_SmoothLine)
{
case _Smoothing.No:
_TimeframeIndex = _Series.OpenTimes.GetIndexByTime(Bars.OpenTimes[index]);
break;
default:
_TimeframeIndex = GetIndexByDate(_Series, Bars.OpenTimes[index]);
break;
}
if (_TimeframeIndex != -1)
{
_Open[index] = _MaOpen.Result[_TimeframeIndex];
_Close[index] = _MaClose.Result[_TimeframeIndex];
_High[index] = _MaHigh.Result[_TimeframeIndex];
_Low[index] = _MaLow.Result[_TimeframeIndex];
_Median[index] = _MaMedian.Result[_TimeframeIndex];
}
}
private int GetIndexByDate(Bars series, DateTime time)
{
for (int i = series.ClosePrices.Count - 1; i > 0; i--)
{
if (series.OpenTimes[i] == time)
return i;
}
return -1;
}
}
}
dadi
Joined on 17.10.2020
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Multi TImeframe Moving Average.algo
- Rating: 4.17
- Installs: 2348
- Modified: 29/03/2022 07:33
Comments
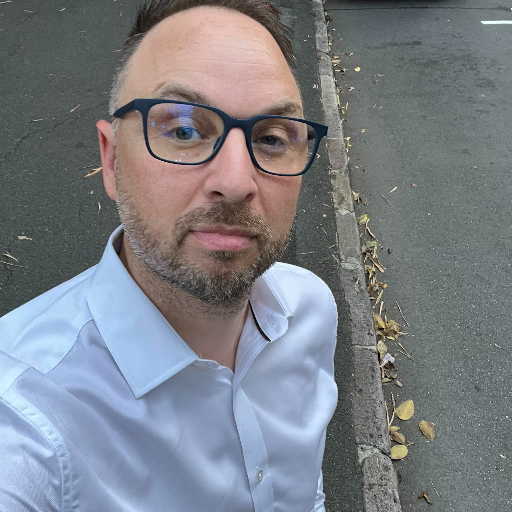
Hey cool indicator ThkU..
How do I reference it in a cBot.?
thanks a lot for information
Absolutely perfect!
Thank you so much for this, appreciate it!
Thank you, that's helping.Jadwal Dokter
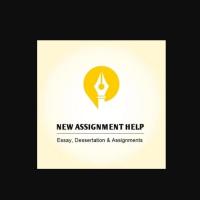
In Need of Management Assignment Writing service. The Company called new Assignment Help professional writers can help you with all your assignment needs. They can provide high-quality assistance with all aspects of your assignment writing and editing with Best Quality. so you can be sure you'll get the grade you need. Get in touch today to get started.
Do you require help with university assignment? Take advantage of the United Kingdom's most trusted writing service to solve all of your university assignment issues with the best writers in the country.
Other Services - Paper help | Thesis help | college assignment help | Assignment Editing Service
Pluralsight incorporates Microsoft Viva. This acquisition will widen and expedite Pluralsight's technical skills development.
ConvertKit is the go-to marketing hub for creators that helps you grow and monetize your audience with ease
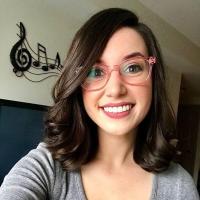
Writing service organizations promise clients of non-infringement to private details. Freelance writing service organizations only use private details for purposes of transaction hence potential clients write my essay cheap are not supposed to be threatened by publicity factor. Many countries benefit also from writing services since the activity is foreign exchange earner.
Great Indicator keep it up :)
THis is awesome!
Is there anyway to get rid of the background surrounding the MA?