Description
Have full control over the original Ichimoku!
As you know in the original Ichimoku indicator it is possible to change Tenkan-sen, Kijun-sen, and Senkou calculation range. In reality, When you are changing the default values, you are changing the foundation of logic behind the tools and you are breaking the relationship between time series and price-action interpretation. So when you want to change default values, it may be a nice idea to change the shift of elements that the original Ichimoku indicator doesn't let you do it.
Jepsian is here to let you have full control over the original Ichimoku. With this indicator, you can change the calculation range and shift the elements to where the suit of your analyzing style is. In addition, with Jepsian you have two extra curves that are usable for some special conformation and analyzing.
Have pay attention all the curves in Jepsian are customizable and you can change the visual side to what you want or disable them.
More info or contact: algo3xp3rt@gmail.com Or github.com/J-Yaghoubi
////////////////////////////////////////////////////////////////////////////////////////
/// Jepsian ///
/// (Have full control over Ichimoku cloud) ///
/// ///
/// Publish date 18-DEC-2021 ///
/// Version 1.0.0 ///
/// By Seyed Jafar Yaghoubi ///
/// License MIT ///
/// More info https://github.com/J-Yaghoubi/ ///
/// Contact algo3xp3rt@gmail.com ///
/// ///
////////////////////////////////////////////////////////////////////////////////////////
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.EasternStandardTime, AccessRights = AccessRights.None)]
[Cloud("Span A", "Span B", Opacity = 0.2)]
public class Jepsian : Indicator
{
#region parameters
[Parameter("Tenkan sen", DefaultValue = 9, MinValue = 1, Group = "Period")]
public int T_Period { get; set; }
[Parameter("Kijun sen", DefaultValue = 26, MinValue = 1, Group = "Period")]
public int K_Period { get; set; }
[Parameter("Senkou span B", DefaultValue = 52, MinValue = 1, Group = "Period")]
public int SB_Period { get; set; }
[Parameter("Quality Line", DefaultValue = 26, MinValue = 1, Group = "Period")]
public int Q_Period { get; set; }
[Parameter("Direction Line", DefaultValue = 26, MinValue = 1, Group = "Period")]
public int D_Period { get; set; }
[Parameter("Chikou Shift", DefaultValue = -26, MaxValue = -1, Group = "Shift")]
public int C_Shift { get; set; }
[Parameter("Kumo Shift", DefaultValue = 26, MinValue = 1, Group = "Shift")]
public int K_Shift { get; set; }
[Parameter("Quality Line", DefaultValue = 26, MinValue = 1, Group = "Shift")]
public int Q_Shift { get; set; }
[Parameter("Direction Line", DefaultValue = -26, MaxValue = -1, Group = "Shift")]
public int D_Shift { get; set; }
[Parameter("Show?", DefaultValue = false, Group = "Last Month H-L")]
public bool MHL { get; set; }
[Parameter("Length", DefaultValue = 26, MinValue = 1, Group = "Last Month H-L")]
public int MHL_Length { get; set; }
[Parameter("Color", DefaultValue = "Maroon", Group = "Last Month H-L")]
public string M_Color { get; set; }
[Parameter("Show?", DefaultValue = false, Group = "Last Day H-L")]
public bool DHL { get; set; }
[Parameter("Length", DefaultValue = 26, MinValue = 1, Group = "Last Day H-L")]
public int DHL_Length { get; set; }
[Parameter("Color", DefaultValue = "DD0070C0", Group = "Last Day H-L")]
public string D_Color { get; set; }
[Output("Tenkan sen", LineStyle = LineStyle.Solid, LineColor = "Red")]
public IndicatorDataSeries T_Result { get; set; }
[Output("Kijun sen", LineStyle = LineStyle.Solid, LineColor = "Blue")]
public IndicatorDataSeries K_Result { get; set; }
[Output("Chiku span", LineStyle = LineStyle.Solid, LineColor = "Green")]
public IndicatorDataSeries C_Result { get; set; }
[Output("Span A", LineStyle = LineStyle.Solid, LineColor = "Green")]
public IndicatorDataSeries SA_Result { get; set; }
[Output("Span B", LineStyle = LineStyle.Solid, LineColor = "FFFF6666")]
public IndicatorDataSeries SB_Result { get; set; }
[Output("Quality Line", LineStyle = LineStyle.Lines, LineColor = "Black")]
public IndicatorDataSeries Q_Result { get; set; }
[Output("Direction Line", LineStyle = LineStyle.Lines, LineColor = "Purple")]
public IndicatorDataSeries D_Result { get; set; }
#endregion
#region Global Variables
private IchimokuKinkoHyo _ICHI;
private Bars Monthly, Daily;
#endregion
protected override void Initialize()
{
_ICHI = Indicators.IchimokuKinkoHyo(T_Period, K_Period, SB_Period);
if (MHL)
Monthly = MarketData.GetBars(TimeFrame.Monthly);
if (DHL)
Daily = MarketData.GetBars(TimeFrame.Daily);
}
//END METHOD INITIALIZE
public override void Calculate(int index)
{
T_Result[index] = _ICHI.TenkanSen.Last(0);
K_Result[index] = _ICHI.KijunSen.Last(0);
C_Result[index + C_Shift] = _ICHI.ChikouSpan.Last(0);
SA_Result[index + K_Shift] = (_ICHI.TenkanSen.Last(0) + _ICHI.KijunSen.Last(0)) / 2;
SB_Result[index + K_Shift] = _ICHI.SenkouSpanB.Last(0);
Q_Result[index + Q_Shift] = _ICHI.KijunSen.Last(0);
D_Result[index + D_Shift] = _ICHI.KijunSen.Last(0);
if (MHL)
{
var m_high = Monthly.HighPrices.Last(1);
var m_low = Monthly.LowPrices.Last(1);
Chart.DrawTrendLine("Monthly High", Chart.LastVisibleBarIndex, m_high, Chart.LastVisibleBarIndex + MHL_Length, m_high, M_Color, 2, LineStyle.Solid);
Chart.DrawTrendLine("Monthly Low", Chart.LastVisibleBarIndex, m_low, Chart.LastVisibleBarIndex + MHL_Length, m_low, M_Color, 2, LineStyle.Solid);
}
if (DHL)
{
var d_high = Daily.HighPrices.Last(1);
var d_low = Daily.LowPrices.Last(1);
Chart.DrawTrendLine("Daily High", Chart.LastVisibleBarIndex, d_high, Chart.LastVisibleBarIndex + DHL_Length, d_high, D_Color, 2, LineStyle.Solid);
Chart.DrawTrendLine("Daily Low", Chart.LastVisibleBarIndex, d_low, Chart.LastVisibleBarIndex + DHL_Length, d_low, D_Color, 2, LineStyle.Solid);
}
Chart.DrawStaticText("Copyright", "ⒸS.J.Yaghoubi", VerticalAlignment.Bottom, HorizontalAlignment.Left, Color.Gray);
}
//END METHOD CALCULATE
}
//END class DisplayDWMPipsonCharts
}
//END namespace cAlgo
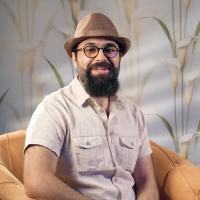
algo3xp3rt
Joined on 17.12.2021
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Jepsian.algo
- Rating: 0
- Installs: 2113
- Modified: 01/03/2022 10:37
Glad to help the community, have a nice time. tnx