Description
VMA Bands are ATR bands with VMA as its center. For a description of options, refer to VMA: Variable Moving Average (VMA) Indicator
Github: GitHub - Doustzadeh/cTrader-Indicator
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, AutoRescale = false, AccessRights = AccessRights.None)]
public class VariableMovingAverageBands : Indicator
{
[Parameter("Source")]
public DataSeries Source { get; set; }
[Parameter("VMA Periods", DefaultValue = 6, MinValue = 2)]
public int Periods { get; set; }
[Parameter("ATR Periods", DefaultValue = 6)]
public int AtrBandPeriods { get; set; }
[Parameter("ATR MA Type", DefaultValue = MovingAverageType.Simple)]
public MovingAverageType AtrBandMaType { get; set; }
[Parameter("Band Multipler", DefaultValue = 1.5, MinValue = 0)]
public double BandMultipler { get; set; }
[Output("Top", LineColor = "DodgerBlue", LineStyle = LineStyle.Solid)]
public IndicatorDataSeries Top { get; set; }
[Output("Bottom", LineColor = "Red", LineStyle = LineStyle.Solid)]
public IndicatorDataSeries Bottom { get; set; }
[Output("VMA", LineColor = "Gold", LineStyle = LineStyle.Solid, Thickness = 2)]
public IndicatorDataSeries VMA { get; set; }
private IndicatorDataSeries pdmS, mdmS, pdiS, mdiS, iS;
private AverageTrueRange ATR;
protected override void Initialize()
{
pdmS = CreateDataSeries();
mdmS = CreateDataSeries();
pdiS = CreateDataSeries();
mdiS = CreateDataSeries();
iS = CreateDataSeries();
ATR = Indicators.AverageTrueRange(AtrBandPeriods, AtrBandMaType);
}
public override void Calculate(int index)
{
if (index < 1)
{
pdmS[index] = 0;
mdmS[index] = 0;
pdiS[index] = 0;
mdiS[index] = 0;
iS[index] = 0;
VMA[index] = 0;
return;
}
double k = 1.0 / Periods;
double pdm = Math.Max((Source[index] - Source[index - 1]), 0);
double mdm = Math.Max((Source[index - 1] - Source[index]), 0);
pdmS[index] = ((1 - k) * pdmS[index - 1]) + (k * pdm);
mdmS[index] = ((1 - k) * mdmS[index - 1]) + (k * mdm);
double s = pdmS[index] + mdmS[index];
double pdi = pdmS[index] / s;
double mdi = mdmS[index] / s;
pdiS[index] = ((1 - k) * pdiS[index - 1]) + (k * pdi);
mdiS[index] = ((1 - k) * mdiS[index - 1]) + (k * mdi);
double d = Math.Abs(pdiS[index] - mdiS[index]);
double s1 = pdiS[index] + mdiS[index];
iS[index] = ((1 - k) * iS[index - 1]) + (k * d / s1);
double hhv = iS.Maximum(Periods);
double llv = iS.Minimum(Periods);
double dif = hhv - llv;
double vI = (iS[index] - llv) / dif;
VMA[index] = ((1 - (k * vI)) * VMA[index - 1]) + (k * vI * Source[index]);
double o = BandMultipler * ATR.Result[index];
Top[index] = VMA[index] + o;
Bottom[index] = VMA[index] - o;
}
}
}
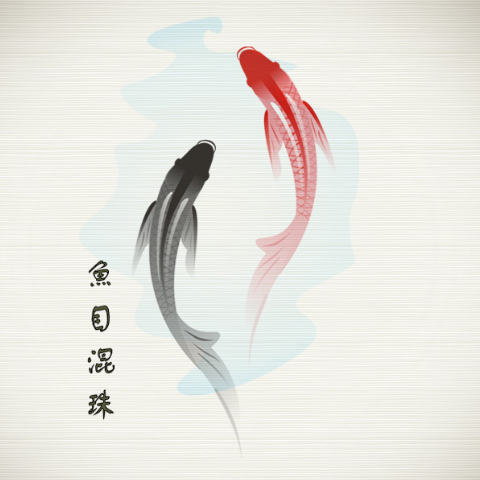
Doustzadeh
Joined on 20.03.2016
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Variable Moving Average Bands.algo
- Rating: 0
- Installs: 1131
- Modified: 12/12/2021 18:09
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.