Description
The Percentage Volume Oscillator (PVO) is a momentum oscillator for volume. The PVO measures the difference between two volume-based moving averages as a percentage of the larger moving average. As with MACD and the Percentage Price Oscillator (PPO), it is shown with a signal line, a histogram and a centerline. The PVO is positive when the shorter volume EMA is above the longer volume EMA and negative when the shorter volume EMA is below. This indicator can be used to define the ups and downs for volume, which can then be used to confirm or refute other signals. Typically, a breakout or support break is validated when the PVO is rising or positive.
Generally speaking, volume is above average when the PVO is positive and below average when the PVO is negative. A negative and rising PVO indicates that volume levels are increasing. A positive and falling PVO indicates that volume levels are decreasing. Chartists can use this information to confirm or refute movements on the price chart.
Even though the PVO is based on a momentum oscillator formula, it is important to remember that moving averages lag.
Github: GitHub - Doustzadeh/cTrader-Indicator
using cAlgo.API;
using cAlgo.API.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = false, AccessRights = AccessRights.None)]
public class PercentageVolumeOscillator : Indicator
{
// Percentage Volume Oscillator (PVO): ((12-day EMA of Volume - 26-day EMA of Volume) / 26-day EMA of Volume) x 100
// Signal Line: 9-day EMA of PVO
// PVO Histogram: PVO - Signal Line
[Parameter("Long Cycle", DefaultValue = 26)]
public int LongCycle { get; set; }
[Parameter("Short Cycle", DefaultValue = 12)]
public int ShortCycle { get; set; }
[Parameter("Signal Periods", DefaultValue = 9)]
public int SignalPeriods { get; set; }
[Output("PVO", LineColor = "DodgerBlue")]
public IndicatorDataSeries PVO { get; set; }
[Output("Signal", LineColor = "Red", LineStyle = LineStyle.Lines)]
public IndicatorDataSeries Signal { get; set; }
[Output("Histogram", LineColor = "White", PlotType = PlotType.Histogram)]
public IndicatorDataSeries Histogram { get; set; }
private ExponentialMovingAverage LongEMA, ShortEMA, SignalEMA;
protected override void Initialize()
{
LongEMA = Indicators.ExponentialMovingAverage(Bars.TickVolumes, LongCycle);
ShortEMA = Indicators.ExponentialMovingAverage(Bars.TickVolumes, ShortCycle);
SignalEMA = Indicators.ExponentialMovingAverage(PVO, SignalPeriods);
}
public override void Calculate(int index)
{
PVO[index] = ((ShortEMA.Result[index] - LongEMA.Result[index]) / LongEMA.Result[index]) * 100;
Signal[index] = SignalEMA.Result[index];
Histogram[index] = PVO[index] - Signal[index];
}
}
}
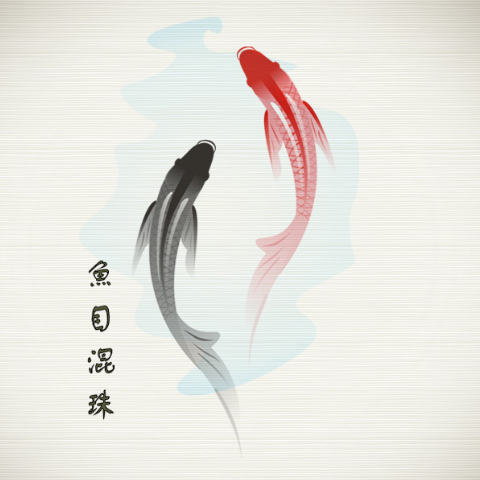
Doustzadeh
Joined on 20.03.2016
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Percentage Volume Oscillator.algo
- Rating: 0
- Installs: 1118
- Modified: 06/12/2021 05:12