Description
The Percentage Price Oscillator (PPO) is a momentum oscillator that measures the difference between two moving averages as a percentage of the larger moving average. As with its cousin, MACD, the Percentage Price Oscillator is shown with a signal line, a histogram and a centerline. Signals are generated with signal line crossovers, centerline crossovers, and divergences. Because these signals are no different than those associated with MACD, this article will focus on a few differences between the two. First, PPO readings are not subject to the price level of the security. Second, PPO readings for different securities can be compared, even when there are large differences in the price.
While MACD measures the absolute difference between two moving averages, PPO makes this a relative value by dividing the difference by the slower moving average (26-day EMA). PPO is simply the MACD value divided by the longer moving average. The result is multiplied by 100 to move the decimal place two spots.
MACD levels are affected by the price of a security. A high-priced security will have higher or lower MACD values than a low-priced security, even if volatility is basically equal. This is because MACD is based on the absolute difference in the two moving averages.
Github: GitHub - Doustzadeh/cTrader-Indicator
using cAlgo.API;
using cAlgo.API.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = false, AccessRights = AccessRights.None)]
public class PercentagePriceOscillator : Indicator
{
// Percentage Price Oscillator (PPO): {(12-day EMA - 26-day EMA)/26-day EMA} x 100
// Signal Line: 9-day EMA of PPO
// PPO Histogram: PPO - Signal Line
[Parameter("Source")]
public DataSeries Source { get; set; }
[Parameter("Long Cycle", DefaultValue = 26)]
public int LongCycle { get; set; }
[Parameter("Short Cycle", DefaultValue = 12)]
public int ShortCycle { get; set; }
[Parameter("Signal Periods", DefaultValue = 9)]
public int SignalPeriods { get; set; }
[Output("PPO", LineColor = "DodgerBlue")]
public IndicatorDataSeries PPO { get; set; }
[Output("Signal", LineColor = "Red", LineStyle = LineStyle.Lines)]
public IndicatorDataSeries Signal { get; set; }
[Output("Histogram", LineColor = "White", PlotType = PlotType.Histogram)]
public IndicatorDataSeries Histogram { get; set; }
private ExponentialMovingAverage LongEMA, ShortEMA, SignalEMA;
protected override void Initialize()
{
LongEMA = Indicators.ExponentialMovingAverage(Source, LongCycle);
ShortEMA = Indicators.ExponentialMovingAverage(Source, ShortCycle);
SignalEMA = Indicators.ExponentialMovingAverage(PPO, SignalPeriods);
}
public override void Calculate(int index)
{
PPO[index] = ((ShortEMA.Result[index] - LongEMA.Result[index]) / LongEMA.Result[index]) * 100;
Signal[index] = SignalEMA.Result[index];
Histogram[index] = PPO[index] - Signal[index];
}
}
}
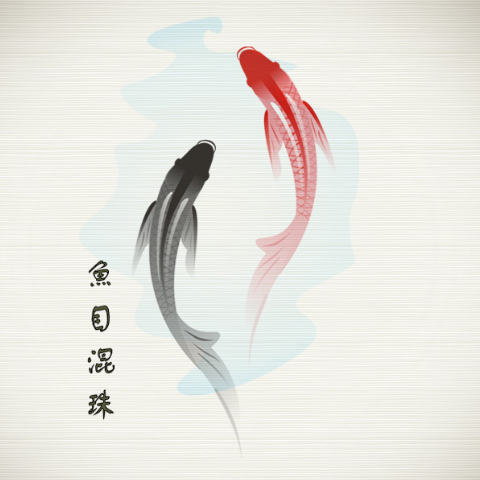
Doustzadeh
Joined on 20.03.2016
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Percentage Price Oscillator.algo
- Rating: 0
- Installs: 1137
- Modified: 06/12/2021 05:05