Description
Price Pressure Momentum (PPM) is a proprietary indicator designed by the trader and portfolio manager veteran Bob Kendall (The Kendall Report -
@PortfolioXpert) back in 80's.
PPM is quite simple indicator measuring SMA percentage change and deriving an SMA line (Brown color line) from that change.
This change SMA is compared to two derivatives/signal lines
I have written this code based on the author's explanation and the visual charts of the PPMs I have seen.
You can see more about PPM from the author himself at https://www.youtube.com/watch?v=9JJxlpG50P0
Default values for the main SMA Period are 10,21 and 40. I'm not sure about the two derived SMAs,
I have tried to visually fit them to the charts I have seen and for 10 main SMA, 10 and 21 derived SMAs (SmoothPeriod & SmoothPeriod2) seem to fit quite well.
NOTE: [Parameter("LookBack", DefaultValue = 1)]. This seems to be the one Kendal is using
I use this indicator with my manual trading as well as automated systems.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
/*
Price Pressure Momentum (PPM) is a proprietary indicator designed by Bob Kendall (The Kendall Report -
@PortfolioXpert) back in 80's.
PPM is quite simple indicator measuring SMA percentage change and deriving an SMA line (Brown color line) from that change.
This change SMA is compared to two derivatives/signal lines
I have written this code based on the author's explanation and the visual charts of the PPMs I have seen.
You can see more about PPM from the author himself at https://www.youtube.com/watch?v=9JJxlpG50P0
Default values for the main SMA Period are 10,21 and 40. I'm not sure about the two derived SMAs,
I have tried to visually fit them to the charts I have seen and for 10 main SMA, 10 and 21 derived SMAs (SmoothPeriod & SmoothPeriod2) seem to fit quite well
*/
namespace cAlgo
{
// [Levels(0)]
[Indicator(IsOverlay = false, ScalePrecision = 5, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class KendallPPM : Indicator
{
[Parameter("Source")]
public DataSeries Source { get; set; }
[Parameter(" Read New Bars Only!", Group = "--- Resource Manager --- (calculate only on new bar!)", DefaultValue = true)]
public bool ReadBars { get; set; }
[Parameter("PPM Period", DefaultValue = 10)]
public int Period { get; set; }
[Parameter("1st Derivative", DefaultValue = 10)]
public int SmoothPeriod { get; set; }
[Parameter("2nd Derivative", DefaultValue = 21)]
public int SmoothPeriod2 { get; set; }
[Parameter("LookBack", DefaultValue = 2)]
public int Lookback { get; set; }
[Parameter("MA Type", DefaultValue = MovingAverageType.Simple)]
public MovingAverageType MAType { get; set; }
[Output("PPM Line", LineColor = "Peru", Thickness = 2, PlotType = PlotType.DiscontinuousLine)]
public IndicatorDataSeries PPM { get; set; }
[Output("ZeroLine", Color = Colors.Honeydew)]
public IndicatorDataSeries zero { get; set; }
private IndicatorDataSeries Acc { get; set; }
[Output("First Derivative", LineColor = "GreenYellow", PlotType = PlotType.DiscontinuousLine)]
public IndicatorDataSeries Smooth1 { get; set; }
[Output("Second Derivative", LineColor = "Aqua", PlotType = PlotType.DiscontinuousLine)]
public IndicatorDataSeries Smooth2 { get; set; }
private MovingAverage MA;
private MovingAverage MA2;
private MovingAverage MA3;
int lastindex = 0;
protected override void Initialize()
{
MA = Indicators.MovingAverage(Source, Period, MAType);
MA2 = Indicators.MovingAverage(MA.Result, SmoothPeriod, MAType);
MA3 = Indicators.MovingAverage(MA.Result, SmoothPeriod2, MAType);
//if (RunningMode == RunningMode.RealTime)
IndicatorArea.DrawHorizontalLine("0", 0, Color.FromHex("Blue"));
}
public override void Calculate(int index)
{
if (ReadBars)
{
if (IsLastBar)
{
if (index != lastindex)
lastindex = index;
else
return;
}
}
PPM[index] = ((MA.Result[index] - MA.Result[index - Lookback]) / MA.Result[index]) * 100;
Smooth1[index] = ((MA2.Result[index] - MA2.Result[index - Lookback]) / MA2.Result[index]) * 100;
Smooth2[index] = ((MA3.Result[index] - MA3.Result[index - Lookback]) / MA3.Result[index]) * 100;
zero[index] = 0;
}
}
}
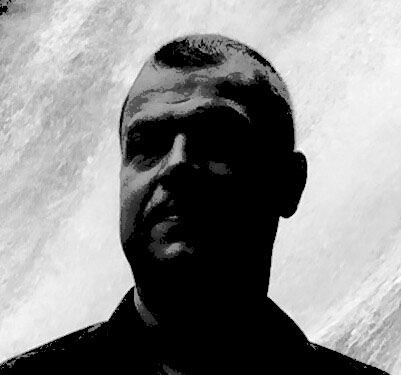
jani
Joined on 05.04.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Kendall PPM.algo
- Rating: 0
- Installs: 1110
- Modified: 01/12/2021 09:21