Description
Hiya!
We have just released an innovative indicator exclusively designed for cTrader. It is a top-notch tool to compare market changes every day.
Features
The Daily H/L indicator can:
- Record all the peak price changes from every daily timeframe.
- Display lines of the previous day on the chart (with a period less than D1 High/Low).
- Set a specific timeframe for the past day.
- Send the alerts when the current price crosses one of the lines.
For example, the indicator allows you to see High/Low from the beginning to the end of the day, or from 8 to 12 am.
Parameters
We have equipped our indicator with more than 14 additional parameters. So, you can configure the indicator according to your needs!
Note! Try Daily H/L on your demo account first before going live.
Other Products
PSAR Strategy:
Ichimoku Strategy:
ADR Custom Indicator:
Contact Info
Contact us via support@4xdev.com
Check out our cozy Telegram blog for traders: https://t.me/Forexdev
Visit our website to find more tools and programming services: https://bit.ly/44BFRG3
Take a look at our YouTube channel: https://www.youtube.com/channel/UChsDb4Q8X2Vl5DJ7H8PzlHQ
Trading secrets are in your hands — with 4xDev.
using System;
using System.Globalization;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
using System.Windows.Forms;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class DailyHighLow : Indicator
{
[Parameter("Start Time", DefaultValue = "2:00 am", Group = "Indicator")]
public string startTime { get; set; }
[Parameter("End Time", DefaultValue = "8:00 am", Group = "Indicator")]
public string endTime { get; set; }
[Parameter("Alerts", DefaultValue = false, Group = "Alerts")]
public bool alertOn { get; set; }
[Parameter("Alerts text", DefaultValue = "Alert", Group = "Alerts")]
public string alert_text { get; set; }
//[Parameter("Email Alert On", DefaultValue = false, Group = "Alerts")]
//public bool emailAlertOn { get; set; }
//[Parameter("Email Adress", DefaultValue = "", Group = "Alerts")]
//public string email_adress { get; set; }
//[Parameter("Email Subject", DefaultValue = "", Group = "Alerts")]
//public string email_subject { get; set; }
//[Parameter("Email Text", DefaultValue = "", Group = "Alerts")]
//public string email_texts { get; set; }
private double high = 0, low = 99999999, maxDay, minDay;
private DateTime startT, endT;
private int oldDay;
[Output("High", LineColor = "Orange")]
public IndicatorDataSeries DayHigh { get; set; }
[Output("Low", LineColor = "Orange")]
public IndicatorDataSeries DayLow { get; set; }
protected override void Initialize()
{
// Initialize and create nested indicators
startT = StringToTime(startTime);
endT = StringToTime(endTime);
}
DateTime time;
public override void Calculate(int index)
{
// Calculate value at specified index
// Result[index] = ...
HighestNLowest(startT, endT, index, ref high, ref low);
if (maxDay != 0 && minDay != 99999999)
{
DayHigh[index] = maxDay;
DayLow[index] = minDay;
if (time != Bars[index].OpenTime)
{
if (Bars[1].Close < maxDay && Bars[0].Close > maxDay)
{
if (alertOn)
{
string message = "Daily H/L Cross High. " + alert_text;
MessageBoxButtons buttons = MessageBoxButtons.OK;
DialogResult result;
result = MessageBox.Show(message, "Alert", buttons);
Print("Daily H/L Cross High. " + alert_text);
}
time = Bars[index].OpenTime;
}
if (Bars[1].Close > maxDay && Bars[0].Close < maxDay)
{
if (alertOn)
{
string message = "Daily H/L Cross Low. " + alert_text;
MessageBoxButtons buttons = MessageBoxButtons.OK;
DialogResult result;
result = MessageBox.Show(message, "Alert", buttons);
Print("Daily H/L Cross High. " + alert_text);
}
//if (emailAlertOn)
// Print("Daily H/L Cross Low. " + alert_text);
time = Bars[index].OpenTime;
}
}
}
}
private DateTime StringToTime(string sTime)
{
if (string.IsNullOrEmpty(sTime))
return DateTime.Now;
CultureInfo provider = CultureInfo.InvariantCulture;
string format = "h:mm tt";
DateTime dTime;
dTime = DateTime.ParseExact(sTime, format, provider);
return dTime;
}
private void HighestNLowest(DateTime startT, DateTime endT, int index, ref double high, ref double low)
{
int endHour = -1;
if (endT.Hour == startT.Hour)
endHour = 24;
else
endHour = endT.Hour;
if ((Bars[index].OpenTime.Hour == 0 && Bars[index].OpenTime.Minute == 0) || Bars[index].OpenTime.Day != oldDay)
{
maxDay = high;
minDay = low;
high = 0;
low = 99999999;
oldDay = Bars[index].OpenTime.Day;
}
if ((Bars[index].OpenTime.Hour >= startT.Hour) && (Bars[index].OpenTime.Hour < endHour) && (Bars[index].OpenTime.Minute >= startT.Minute))
{
high = Math.Max(Bars[index].High, high);
low = Math.Min(Bars[index].Low, low);
}
if (Bars[index].OpenTime.Hour == endHour && Bars[index].OpenTime.Minute >= endT.Minute)
{
oldDay = Bars[index].OpenTime.Day;
}
}
}
}
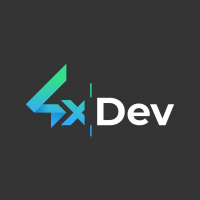
4xDev
Joined on 11.12.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Daily_High_Low.algo
- Rating: 5
- Installs: 2717
- Modified: 16/11/2021 12:45
Comments
بحلول منتصف القرن السابع عشر تم اختراع العدسات المصنوعة بدقة، وبالتالي تم استخدام حجرة التصوير من أجل تشكيل صور واقعية في العالم، كما تم استخدام الفوانيس السحرية (بالإنجليزية: Magic lanterns)، وتشبه فكرتها فكرة أجهزة العرض الحديثة، التي ساعدت في عرض صور مرسومة على شرائح زجاجية على أسطح كبيرة. استخدام المواد الكيميائية الحساسة في إنتاج الصور الفوتوغرافية من قبل العالم الألماني يوهان هاينريش في عام 1727م.
استديو نسائي
الفكرة: وهي أساس كل صورة إبداعيّة، فالصورة تحاول إيصال رسالة معينّة أو لقطة جمالية مُبهرة. زاوية الالتقاط: وهي تعتمد على الفكرة، حيث إنّ لكل فكرة زاوية التقاط توضّحها وتزيدها جمالاً. الإضاءة: وهي تعلب دوراً كبيراً في إيصال فكرة الصورة، فلكل فكرة إضاءة معيّنة، ولكل إضاءة خصائص معيّنة يجب معرفتها لتحديد ما يُناسب الفكرة. التركيز: ويعني التركيز على شيء معيّن في الصورة يُشكّل الجزء الأهم من اللقطة ويُبرز الموضوع الذي تتناوله الصورة.
يعتبر التعرف على طرق التعامل مع الكاميرا أول خطوات تعلم عن التصوير الفوتوغرافي، لهذا يجب البدء بالتعرف على الكاميرا وطريقة استعمالها وما هي إمكانياتها، من خلال قراءة دليل الاستخدام، كما يمكن أيضاً استخراج الكثير من المعلومات من الشبكة العنكبوتية من المواقع الخاصة بنوع الكاميرا المستعملة.
افكار تصوير منتجات
Excellent indicator and I really like the ability to set the start and stop times within which to calculate the highs and lows. I have two requests. Would it be possible to modify the code to add a text label to say something like D-1L, or D-1H, or a user input text label. Also, could the code be modified to be able to calculate and plot the high and low of 2 days and 3 days back using the same user defined start and stop times? Many thanks