Description
Ichimoku Kinko Hyo Indicator
Enhanced version with option to select a time frame of your preference.
E.g. you can draw 1H Ichimoku on 30min chart as shown on the picture:
Note: This Indicator only works with Standard time frames (Tick, Renko and Range are not supported)
Version history:
v1.0 - initial release
v1.1 - missing Functions class added
// Ichimoku Multi-TimeFrame v1.0 by VFX
// https://ctrader.com/algos/indicators/show/2766
// Version history:
// v1.0 - initial release
// v1.1 - missing Functions class added
using cAlgo.API;
using cAlgo.API.Indicators;
using System.Collections.Generic;
namespace cAlgo.Indicators
{
[Cloud("Senkou Span A", "Senkou Span B")]
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class VFX_Ichimoku_MultiTF : Indicator
{
[Parameter("Use Other TF", DefaultValue = false)]
public bool parUseTFPrice { get; set; }
[Parameter("Time Frame", DefaultValue = "Hour")]
public TimeFrame parTF { get; set; }
[Parameter("Tenkan Sen Periods", DefaultValue = 9, MinValue = 1)]
public int parTenkanSenPeriods { get; set; }
[Parameter("Kijun Sen Periods", DefaultValue = 26, MinValue = 1)]
public int parKijunSenPeriods { get; set; }
[Parameter("Senkou Span B Periods", DefaultValue = 52, MinValue = 1)]
public int parSenkouSpanBPeriods { get; set; }
[Output("Tenkan Sen", LineColor = "FF1E90FF")]
public IndicatorDataSeries TenkanSen { get; set; }
[Output("Kijun Sen", LineColor = "FFDC143C")]
public IndicatorDataSeries KijunSen { get; set; }
[Output("Chikou Span", LineColor = "FF00FA9A")]
public IndicatorDataSeries ChikouSpan { get; set; }
[Output("Senkou Span A", LineColor = "FF2E8B57")]
public IndicatorDataSeries SenkouSpanA { get; set; }
[Output("Senkou Span B", LineColor = "FFFF0000")]
public IndicatorDataSeries SenkouSpanB { get; set; }
private IchimokuKinkoHyo IKH;
private Bars TFbars;
private double TFratio;
private int indexTF, prevIndexTF, indexShift;
private Functions Fn = new Functions();
protected override void Initialize()
{
prevIndexTF = -1;
TFbars = parUseTFPrice ? MarketData.GetBars(parTF) : Bars;
TFratio = parUseTFPrice ? (double)Fn.TimeFrameInMinutes(parTF) / (double)Fn.TimeFrameInMinutes(TimeFrame) : 1;
indexShift = (int)(parKijunSenPeriods * TFratio);
IKH = Indicators.IchimokuKinkoHyo(TFbars, parTenkanSenPeriods, parKijunSenPeriods, parSenkouSpanBPeriods);
}
public override void Calculate(int index)
{
indexTF = parUseTFPrice ? TFbars.OpenTimes.GetIndexByTime(Bars.OpenTimes[index]) : index;
if (indexTF > parKijunSenPeriods)
{
if (IsLastBar)
{
// approximate and draw middle points
double indexStep = 1.0 / TFratio;
TenkanSen[index] = (1.0 - indexStep) * IKH.TenkanSen[prevIndexTF] + indexStep * IKH.TenkanSen[indexTF];
KijunSen[index] = (1.0 - indexStep) * IKH.KijunSen[prevIndexTF] + indexStep * IKH.KijunSen[indexTF];
ChikouSpan[index - indexShift] = (1.0 - indexStep) * IKH.ChikouSpan[prevIndexTF - parKijunSenPeriods] + indexStep * IKH.ChikouSpan[indexTF - parKijunSenPeriods];
SenkouSpanA[index + indexShift] = (1.0 - indexStep) * IKH.SenkouSpanA[prevIndexTF + parKijunSenPeriods] + indexStep * IKH.SenkouSpanA[indexTF + parKijunSenPeriods];
SenkouSpanB[index + indexShift] = (1.0 - indexStep) * IKH.SenkouSpanB[prevIndexTF + parKijunSenPeriods] + indexStep * IKH.SenkouSpanB[indexTF + parKijunSenPeriods];
}
if (indexTF > prevIndexTF)
{
// redraw the previous points (their final exact values)
TenkanSen[index - 1] = IKH.TenkanSen[prevIndexTF];
KijunSen[index - 1] = IKH.KijunSen[prevIndexTF];
ChikouSpan[index - 1 - indexShift] = IKH.ChikouSpan[prevIndexTF - parKijunSenPeriods];
SenkouSpanA[index - 1 + indexShift] = IKH.SenkouSpanA[prevIndexTF + parKijunSenPeriods];
SenkouSpanB[index - 1 + indexShift] = IKH.SenkouSpanB[prevIndexTF + parKijunSenPeriods];
prevIndexTF = indexTF;
}
}
}
public class Functions
{
public int TimeFrameInMinutes(TimeFrame TF)
{
int result;
if (TimeFrameMinutes.TryGetValue(TF, out result))
return result;
return 0;
}
public static readonly Dictionary<TimeFrame, int> TimeFrameMinutes;
static Functions()
{
TimeFrameMinutes = new Dictionary<TimeFrame, int>
{
{
TimeFrame.Minute,
1
},
{
TimeFrame.Minute2,
2
},
{
TimeFrame.Minute3,
3
},
{
TimeFrame.Minute4,
4
},
{
TimeFrame.Minute5,
5
},
{
TimeFrame.Minute6,
6
},
{
TimeFrame.Minute7,
7
},
{
TimeFrame.Minute8,
8
},
{
TimeFrame.Minute9,
9
},
{
TimeFrame.Minute10,
10
},
{
TimeFrame.Minute15,
15
},
{
TimeFrame.Minute20,
20
},
{
TimeFrame.Minute30,
30
},
{
TimeFrame.Minute45,
45
},
{
TimeFrame.Hour,
60
},
{
TimeFrame.Hour2,
60 * 2
},
{
TimeFrame.Hour3,
60 * 3
},
{
TimeFrame.Hour4,
60 * 4
},
{
TimeFrame.Hour6,
60 * 6
},
{
TimeFrame.Hour8,
60 * 8
},
{
TimeFrame.Hour12,
60 * 12
},
{
TimeFrame.Daily,
60 * 24
},
{
TimeFrame.Day2,
60 * 24 * 2
},
{
TimeFrame.Day3,
60 * 24 * 3
},
{
TimeFrame.Weekly,
60 * 24 * 7
},
{
TimeFrame.Monthly,
365 / 12 * 24 * 60
}
};
}
}
}
}
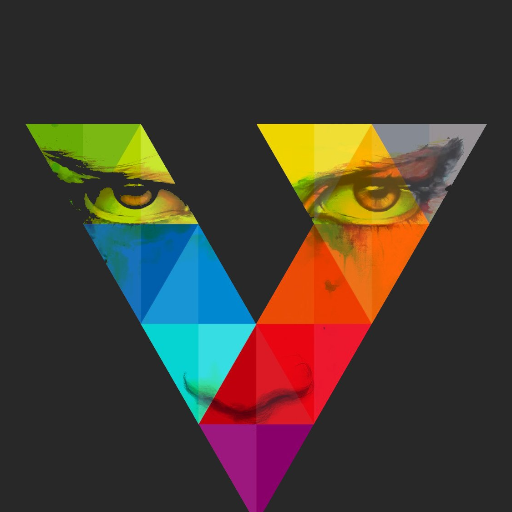
vitofx
Joined on 08.03.2021
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: VFX_Ichimoku_MultiTF_v1.1.algo
- Rating: 0
- Installs: 1521
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.