Description
Hello,
This indicator filters out price noise using the Heiken Ashi technique and time noise when applied to the Renko chart.
The purpose of Exponential Moving Averages is to correct the lag of simple moving averages.
I would like it to be a source of inspiration to help you to develop your own ideas.
Thanks for your kind interest.
Dedicated to the Beach Boys.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class Waves : Indicator
{
[Parameter("slowPeriods", DefaultValue = 14)]
public int slowPeriods { get; set; }
[Parameter("fastPeriods", DefaultValue = 14)]
public int fastPeriods { get; set; }
[Output("Result1", LineColor = "Red")]
public IndicatorDataSeries Result1 { get; set; }
[Output("Result2", LineColor = "Green")]
public IndicatorDataSeries Result2 { get; set; }
[Output("Result3", LineColor = "Aqua")]
public IndicatorDataSeries Result3 { get; set; }
private IndicatorDataSeries haOpen, haClose, result;
private ExponentialMovingAverage openEma, closeEma;
private double open, close, high, low;
private double max, min;
protected override void Initialize()
{
haOpen = CreateDataSeries();
haClose = CreateDataSeries();
result = CreateDataSeries();
openEma = Indicators.ExponentialMovingAverage(haOpen, slowPeriods);
closeEma = Indicators.ExponentialMovingAverage(haClose, slowPeriods);
}
public override void Calculate(int index)
{
open = Bars.OpenPrices[index];
high = Bars.HighPrices[index];
low = Bars.LowPrices[index];
close = Bars.ClosePrices[index];
haClose[index] = (open + high + low + close) / 4;
if (index > 0)
haOpen[index] = (haOpen[index - 1] + haClose[index - 1]) / 2;
else
haOpen[index] = (open + close) / 2;
result[index] = closeEma.Result[index] - openEma.Result[index];
max = 0;
min = 0;
for (int i = 1; i <= fastPeriods; i++)
{
max += result.Maximum(i);
min += result.Minimum(i);
}
max = max / fastPeriods;
min = min / fastPeriods;
Result1[index] = max;
Result2[index] = min;
Result3[index] = result[index];
}
}
}
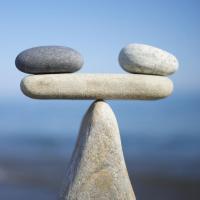
srm_bcn
Joined on 01.09.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Waves.algo
- Rating: 0
- Installs: 1318
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
AC
Brilliant mate! And it perfectly shows flat periods and future directions!
I don't know why did you call it waves, because it seems close to bollinger band, or may be you've uploaded the wrong algo file because of downloaded indicator doesn't seems as show off in the description. Anyway I must appreciate your efforts