Description
Ladies and gentlemen, welcome.
I present another indicator from my collection. This is a Renko chart and the calculation of its balance lines. It is only a reference since it takes values from the Renco graph to calculate the balance lines and not the value of the real tick. The size of the brick can be customized.
It is not really a Renko chart, but the calculations are based on this concept.
I do not recommend it for trading, only for market research.
Sincerely,
TF: t1 - Brick size: 0.1 pips
Take a look of the risk:
Many thanks to the Japanese people for the Renko charts.
It stay a lot of learn.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class MRRenkoOverlay : Indicator
{
[Parameter("Brick Size", DefaultValue = 0.0001, Step = 1E-05)]
public double brickSize { get; set; }
[Output("Result1", LineColor = "lightGreen")]
public IndicatorDataSeries Result1 { get; set; }
[Output("Result2", LineColor = "Red")]
public IndicatorDataSeries Result2 { get; set; }
[Output("Result3", LineColor = "DodgerBlue")]
public IndicatorDataSeries Result3 { get; set; }
[Output("Result4", LineColor = "DodgerBlue")]
public IndicatorDataSeries Result4 { get; set; }
[Output("Result5", LineColor = "DodgerBlue")]
public IndicatorDataSeries Result5 { get; set; }
[Output("Result6", LineColor = "Orange")]
public IndicatorDataSeries Result6 { get; set; }
private double price, level, previousLevel, result;
private bool bullish, bearish;
private int buyCount, sellCount;
private double buySum, sellSum;
private double buyAverage;
private double sellAverage;
private double CenterMean, UpperMean, LowerMean;
private double step;
private double max, min;
protected override void Initialize()
{
previousLevel = Math.Round(Bars.ClosePrices.LastValue, 4);
bullish = true;
bearish = false;
}
public override void Calculate(int index)
{
price = Bars.ClosePrices[index];
step = brickSize * 10000;
level = Math.Round((price / step), 4) * step;
if (level > previousLevel)
if (price < level)
level = level - brickSize;
if (level < previousLevel)
if (price > level)
level = level + brickSize;
result = Math.Round((level - previousLevel) * 10, 4) / 10;
if (bullish)
{
if (result == -brickSize)
level = level + brickSize;
if (result == -2 * brickSize || result < -2 * brickSize)
{
bullish = false;
bearish = true;
}
}
if (bearish)
{
if (result == brickSize)
level = level - brickSize;
if (result == 2 * brickSize || result > 2 * brickSize)
{
bullish = true;
bearish = false;
}
}
result = Math.Round((level - previousLevel) * 10, 4) / 10;
if (result != 0)
{
buyCount++;
buySum += level;
sellCount++;
sellSum += level;
}
buyAverage = Math.Round(buySum / buyCount, 5);
sellAverage = Math.Round(sellSum / sellCount, 5);
if (level > buyAverage)
{
buyAverage = level;
buyCount = 1;
buySum = level;
max = level;
}
if (level < sellAverage)
{
sellAverage = level;
sellCount = 1;
sellSum = level;
min = level;
}
CenterMean = (buyAverage + sellAverage) / 2;
UpperMean = (buyAverage + CenterMean) / 2;
LowerMean = (CenterMean + sellAverage) / 2;
Result1[index] = sellAverage;
Result2[index] = buyAverage;
Result3[index] = CenterMean;
Result4[index] = UpperMean;
Result5[index] = LowerMean;
Result6[index] = level;
previousLevel = level;
}
}
}
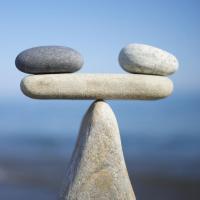
srm_bcn
Joined on 01.09.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: MRRenkoOverlay.algo
- Rating: 0
- Installs: 1214
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.