Description
This tool adds Hotkeys to change the timeframe. (cTrader ver4.0 or later is required)
Other tools can be downloaded from Gumroad. (for free)
using cAlgo.API;
namespace cAlgo {
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class ChangeTimeFrameHotkey : Indicator {
[Parameter("Minute", DefaultValue = Key.D1, Group = "Key Assignment")]
public Key M1Key { get; set; }
[Parameter("Minute5", DefaultValue = Key.D2, Group = "Key Assignment")]
public Key M5Key { get; set; }
[Parameter("Minute15", DefaultValue = Key.D3, Group = "Key Assignment")]
public Key M15Key { get; set; }
[Parameter("Minute30", DefaultValue = Key.D4, Group = "Key Assignment")]
public Key M30Key { get; set; }
[Parameter("Hour", DefaultValue = Key.D5, Group = "Key Assignment")]
public Key H1Key { get; set; }
[Parameter("Hour4", DefaultValue = Key.D6, Group = "Key Assignment")]
public Key H4Key { get; set; }
[Parameter("Daily", DefaultValue = Key.D7, Group = "Key Assignment")]
public Key DailyKey { get; set; }
[Parameter("Weekly", DefaultValue = Key.D8, Group = "Key Assignment")]
public Key WeeklyKey { get; set; }
[Parameter("Monthly", DefaultValue = Key.D9, Group = "Key Assignment")]
public Key MonthlyKey { get; set; }
[Parameter("Minute", DefaultValue = false, Group = "Button in Chart")]
public bool ShowM1 { get; set; }
[Parameter("Minute5", DefaultValue = false, Group = "Button in Chart")]
public bool ShowM5 { get; set; }
[Parameter("Minute15", DefaultValue = false, Group = "Button in Chart")]
public bool ShowM15 { get; set; }
[Parameter("Minute30", DefaultValue = false, Group = "Button in Chart")]
public bool ShowM30 { get; set; }
[Parameter("Hour", DefaultValue = false, Group = "Button in Chart")]
public bool ShowH1 { get; set; }
[Parameter("Hour4", DefaultValue = false, Group = "Button in Chart")]
public bool ShowH4 { get; set; }
[Parameter("Daily", DefaultValue = false, Group = "Button in Chart")]
public bool ShowDaily { get; set; }
[Parameter("Weekly", DefaultValue = false, Group = "Button in Chart")]
public bool ShowWeekly { get; set; }
[Parameter("Monthly", DefaultValue = false, Group = "Button in Chart")]
public bool ShowMonthly { get; set; }
[Parameter(DefaultValue = "Renko10", Group = "Custom TimeFrame")]
public TimeFrame MyTimeFrame { get; set; }
[Parameter("HotKey", DefaultValue = Key.D0, Group = "Custom TimeFrame")]
public Key MyTFKey { get; set; }
[Parameter("Show Button", DefaultValue = false, Group = "Custom TimeFrame")]
public bool ShowCustom { get; set; }
protected override void Initialize() {
Chart.AddHotkey(() => Chart.TryChangeTimeFrame(TimeFrame.Minute), M1Key);
Chart.AddHotkey(() => Chart.TryChangeTimeFrame(TimeFrame.Minute5), M5Key);
Chart.AddHotkey(() => Chart.TryChangeTimeFrame(TimeFrame.Minute15), M15Key);
Chart.AddHotkey(() => Chart.TryChangeTimeFrame(TimeFrame.Minute30), M30Key);
Chart.AddHotkey(() => Chart.TryChangeTimeFrame(TimeFrame.Hour), H1Key);
Chart.AddHotkey(() => Chart.TryChangeTimeFrame(TimeFrame.Hour4), H4Key);
Chart.AddHotkey(() => Chart.TryChangeTimeFrame(TimeFrame.Daily), DailyKey);
Chart.AddHotkey(() => Chart.TryChangeTimeFrame(TimeFrame.Weekly), WeeklyKey);
Chart.AddHotkey(() => Chart.TryChangeTimeFrame(TimeFrame.Monthly), MonthlyKey);
Chart.AddHotkey(() => Chart.TryChangeTimeFrame(MyTimeFrame), MyTFKey);
var panel = new StackPanel {
HorizontalAlignment = HorizontalAlignment.Left,
VerticalAlignment = VerticalAlignment.Center,
};
if (ShowM1) AddButton(panel, TimeFrame.Minute);
if (ShowM5) AddButton(panel, TimeFrame.Minute5);
if (ShowM15) AddButton(panel, TimeFrame.Minute15);
if (ShowM30) AddButton(panel, TimeFrame.Minute30);
if (ShowH1) AddButton(panel, TimeFrame.Hour);
if (ShowH4) AddButton(panel, TimeFrame.Hour4);
if (ShowDaily) AddButton(panel, TimeFrame.Daily);
if (ShowWeekly) AddButton(panel, TimeFrame.Weekly);
if (ShowMonthly) AddButton(panel, TimeFrame.Monthly);
if (ShowCustom) AddButton(panel, MyTimeFrame);
Chart.AddControl(panel);
}
private void AddButton(Panel panel, TimeFrame tf) {
var num = System.Text.RegularExpressions.Regex.Replace(tf.ToString(), "[^0-9]", "");
if (num == "") num = "1";
var str = tf.ToString();
var sign = str.Substring(0, 1);
if (str.Contains("Minute") || str.Contains("Hour")) {
sign = sign.ToLower();
} else if (sign == "R") {
sign = str.Substring(0, 2);
}
var signText = new TextBlock {
Text = sign,
Padding = 0,
FontSize = 10,
HorizontalAlignment = HorizontalAlignment.Left,
};
var numText = new TextBlock {
Text = num,
Padding = 0,
FontSize = 8,
HorizontalAlignment = HorizontalAlignment.Right,
};
var inner = new WrapPanel {
Orientation = Orientation.Vertical,
ItemHeight = 10,
};
inner.AddChild(signText);
inner.AddChild(numText);
var button = new Button {
Content = inner,
Padding = 0,
Width = 22,
Height = 22,
Margin = 2,
};
if (tf == TimeFrame) button.IsEnabled = false;
button.Click += _ => Chart.TryChangeTimeFrame(tf);
panel.AddChild(button);
}
public override void Calculate(int index) {
}
}
}
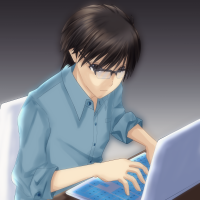
ajinori
Joined on 06.10.2020
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: ChangeTimeFrameHotkey.algo
- Rating: 5
- Installs: 1190
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.