Description
the TDFI Trend indicator, which has a similar name as the TDI (Traders Dynamic Index) is a completly different indicator. To learn more about it, there is a lot of material online for other platforms, but until now the TDFI indicator was not available for cTrader. I would be happy if this indicator is helpful for your trading journey and if it ends up being part of your NNFX algorithm. In order to make this indicator work properly, you have to download and reference this one also (
)
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class TDFInnfxabbu : Indicator
{
[Parameter("Lookback", DefaultValue = 13, MinValue = 2, MaxValue = 30, Step = 1)]
public int lookback { get; set; }
[Parameter("MMA Length", DefaultValue = 13, MinValue = 2, MaxValue = 30, Step = 1)]
public int mmaLength { get; set; }
[Parameter("SMMA Length", DefaultValue = 13, MinValue = 2, MaxValue = 30, Step = 1)]
public int smmaLength { get; set; }
[Parameter("SMMA Mode", DefaultValue = MovingAverageType.Exponential)]
public MovingAverageType maType { get; set; }
[Parameter("N Length", DefaultValue = 3, MinValue = 1, MaxValue = 30, Step = 2)]
public int nLength { get; set; }
[Parameter("Filter Distance", DefaultValue = 0.05, MinValue = 0, MaxValue = 0.5, Step = 0.005)]
public double filterHigh { get; set; }
public MovingAverage mma;
public MovingAverage smma;
public double impetmma;
public double impetsmma;
public double divma;
public double averimpet;
public BARSCLOSE1000 source;
private IndicatorDataSeries tdf;
private IndicatorDataSeries tdfabs;
[Output("Result")]
public IndicatorDataSeries Result { get; set; }
[Output("Highfilter", LineColor = "FFB3B3B3", LineStyle = LineStyle.DotsRare, Thickness = 1)]
public IndicatorDataSeries highFilter { get; set; }
[Output("Lowfilter", LineColor = "FFB3B3B3", LineStyle = LineStyle.DotsRare, Thickness = 1)]
public IndicatorDataSeries lowFilter { get; set; }
protected override void Initialize()
{
source = Indicators.GetIndicator<BARSCLOSE1000>(0);
mma = Indicators.MovingAverage(source.Result, mmaLength, maType);
smma = Indicators.MovingAverage(mma.Result, smmaLength, maType);
tdf = CreateDataSeries();
tdfabs = CreateDataSeries();
}
public override void Calculate(int index)
{
impetmma = mma.Result.Last(0) - mma.Result.Last(1);
impetsmma = smma.Result.Last(0) - smma.Result.Last(1);
divma = Math.Abs(mma.Result.Last(0) - smma.Result.Last(0));
averimpet = (impetmma + impetsmma) / 2;
tdf[index] = (Math.Pow(divma, 1) * Math.Pow(averimpet, nLength));
tdfabs[index] = Math.Abs(tdf[index]);
Result[index] = tdf[index] / tdfabs.Maximum(lookback * nLength);
highFilter[index] = filterHigh;
lowFilter[index] = filterHigh * -1;
}
}
}
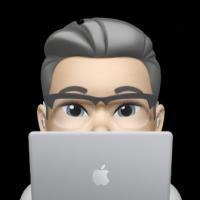
xabbu
Joined on 20.07.2020
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: TDFI.algo
- Rating: 0
- Installs: 2229
- Modified: 13/10/2021 09:54
Comments
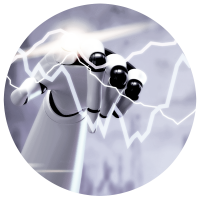
Version 2
protected override void Initialize()
{
mma = Indicators.MovingAverage(Bars.ClosePrices, mmaLength, maType);
[...]}
None of them showed any difference.
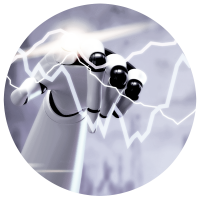
I made two versions of this indicator to see the difference the BARSCLOSE1000 indicator does by multiplying the closing price by 1000.
Version 1 (only modifications)
[...]
private IndicatorDataSeries source;
[...]
protected override void Initialize()
{
source = CreateDataSeries();
mma = Indicators.MovingAverage(source, mmaLength, maType);
smma = Indicators.MovingAverage(mma.Result, smmaLength, maType);[...]
}public override void Calculate(int index)
{
source[index] = Bars.ClosePrices[index] * 1000;
Sorry! Ive figured it out, i was being stupid! thanks for the great work!
I click on TDFI but the "Manage References" button is greyed out and the page says 'source code is not available" i assume im doing something wrong!
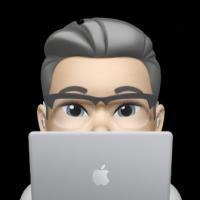
where do you struggle?
download both and than go to the TDFI and click "Manage References" on the top and find the second indicator, mark it and click "apply" and you are done
Could you help me install this?, im unable to reference the other file you have included?
Chart image: link
Do you know why is that?