Description
⏰ Original source code came from Sergio Raimí Mateos, I've only adapted it to support multiple timeframes.
⏰ Link to the original indicator: https://ctrader.com/algos/indicators/show/2257
Calculations made using a different timeframe will be shown directly in the chart
⏰ This indicator plots 3 lines:
- Mean
- Buy Avg.
- Sell Avg.
⏰ A useful tool to measure spacing between means, providing a way to identify Mean Reversion trades.
⏰ Serves as a dynamic level of support/resistance.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class MeanReversionOverlayMultiTF : Indicator
{
[Parameter()]
public TimeFrame SourceTf { get; set; }
[Output("sellAverage", LineColor = "lightGreen")]
public IndicatorDataSeries SellAvg { get; set; }
[Output("buyAverage", LineColor = "Red")]
public IndicatorDataSeries BuyAvg { get; set; }
[Output("Mean", LineColor = "Aqua")]
public IndicatorDataSeries Result { get; set; }
private Bars ctf;
private int idx;
private int previousIdx;
private int buyPeriod;
private int sellPeriod;
private double buyAverage;
private double sellAverage;
private int savedbp;
private int savedsp;
private double mean;
private int buyCount, sellCount;
protected override void Initialize()
{
ctf = MarketData.GetBars(SourceTf);
}
public override void Calculate(int index)
{
idx = ctf.OpenTimes.GetIndexByTime(Bars.OpenTimes[index]);
if (idx > previousIdx)
{
buyPeriod++;
savedbp = buyPeriod;
sellPeriod++;
savedsp = sellPeriod;
}
if (buyPeriod == 0)
buyPeriod = savedbp;
if (sellPeriod == 0)
sellPeriod = savedsp;
buyAverage = 0;
buyCount = 0;
for (int i = idx - buyPeriod + 1; i <= idx; i++)
if (ctf.ClosePrices[i] - ctf.ClosePrices[i - 1] < 0)
{
buyAverage += ctf.OpenPrices[i];
buyCount++;
}
if (buyCount > 0)
buyAverage = buyAverage / buyCount;
else
buyAverage = ctf.ClosePrices[idx];
sellAverage = 0;
sellCount = 0;
for (int i = idx - sellPeriod + 1; i <= idx; i++)
if (ctf.ClosePrices[i] - ctf.ClosePrices[i - 1] > 0)
{
sellAverage += ctf.OpenPrices[i];
sellCount++;
}
if (sellCount > 0)
sellAverage = sellAverage / sellCount;
else
sellAverage = ctf.ClosePrices[idx];
if (ctf.ClosePrices[idx] > buyAverage)
{
buyAverage = ctf.ClosePrices[idx];
buyPeriod = 0;
}
if (ctf.ClosePrices[idx] < sellAverage)
{
sellAverage = ctf.ClosePrices[idx];
sellPeriod = 0;
}
mean = (buyAverage + sellAverage) / 2;
SellAvg[index] = sellAverage;
BuyAvg[index] = buyAverage;
Result[index] = mean;
previousIdx = idx;
}
}
}
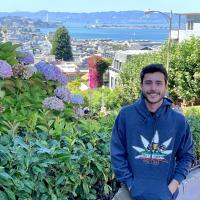
lorenzopvella
Joined on 22.08.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: MeanReversionOverlay Multi TF.algo
- Rating: 0
- Installs: 1294
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.