Description
This indicator helps you draw many moving averages just by typing the periods in a CSV (comma-separated values format), up to 100 moving averages are allowed.
Instead of adding moving averages one by one, you can use this handy tool to draw many with little effort.
using System;
using System.Collections.Generic;
using System.Diagnostics;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
using cAlgo.Properties;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)]
public class AlgoCornerDownloads : Indicator
{
private readonly Dictionary<string, string> _dictionary = new Dictionary<string, string>
{
{"Candlestick Pattern Finder", "https://gumroad.com/l/RPUMy"},
{"1-100 Moving Averages", "https://gumroad.com/l/NxFvIl"},
{"Trading Dashboard", "https://gumroad.com/l/thdYI"},
{"Advanced Crosshair", "https://gumroad.com/l/jjcJy"},
{"Avg Candle Segments", "https://gumroad.com/l/IEwkDD"}
};
protected override void Initialize()
{
Chart.DisplaySettings.QuickTradeButtons = false;
Chart.DisplaySettings.ChartScale = false;
var canvas = new Canvas
{
BackgroundColor = Color.White,
Width = Chart.Width * 3,
Height = Chart.Height * 3
};
Chart.AddControl(canvas);
var stackPanel = new StackPanel
{
HorizontalAlignment = HorizontalAlignment.Center,
VerticalAlignment = VerticalAlignment.Center
};
var images = new Image
{
Source = Resources.algocorner400,
Width = 300,
};
var scrollViewer = new ScrollViewer()
{
BackgroundColor = Color.Transparent,
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled,
VerticalScrollBarVisibility = ScrollBarVisibility.Auto,
};
var productsStack = new StackPanel();
foreach (var product in _dictionary)
{
var button = new Button
{
Text = string.Format("{0}", product.Key),
FontSize = 16,
Width = 300,
FontWeight = FontWeight.Bold,
HorizontalContentAlignment = HorizontalAlignment.Center,
ForegroundColor = Color.Black,
BackgroundColor = Color.WhiteSmoke,
HorizontalAlignment = HorizontalAlignment.Center,
VerticalAlignment = VerticalAlignment.Center,
Padding = 5,
Margin = 5,
};
button.Click += args => Process.Start(product.Value);
productsStack.AddChild(button);
}
scrollViewer.Content = productsStack;
stackPanel.AddChild(images);
stackPanel.AddChild(scrollViewer);
var copyrightText = new TextBlock
{
Text = string.Format("© {0} AlgoCorner. All rights reserved.", Server.Time.Year),
FontSize = 14,
ForegroundColor = Color.Black,
HorizontalAlignment = HorizontalAlignment.Center,
VerticalAlignment = VerticalAlignment.Bottom,
BackgroundColor = Color.Transparent,
FontWeight = FontWeight.Bold,
};
#region Media
var contactStack = new WrapPanel
{
HorizontalAlignment = HorizontalAlignment.Right,
VerticalAlignment = VerticalAlignment.Bottom,
};
var mailIconImage = new Image
{
Source = Resources.icons8_composing_mail_100,
Width = 70,
};
var mailContact = new Button
{
Content = mailIconImage,
ForegroundColor = Color.Black,
HorizontalAlignment = HorizontalAlignment.Right,
VerticalAlignment = VerticalAlignment.Top,
BackgroundColor = Color.Transparent,
FontWeight = FontWeight.Bold,
Margin = 10,
BorderThickness = 1,
BorderColor = Color.Black
};
mailContact.Click += args =>
{
string mailto = string.Format("mailto:{0}?Subject={1}&Body={2}", "xavier@algocorner.com", "Subject", "Message Body");
Process.Start(mailto);
};
var twitterIconImage = new Image
{
Source = Resources.twitter,
Width = 70,
};
var twitterFollow = new Button
{
Content = twitterIconImage,
ForegroundColor = Color.Black,
HorizontalAlignment = HorizontalAlignment.Right,
VerticalAlignment = VerticalAlignment.Top,
BackgroundColor = Color.Transparent,
FontWeight = FontWeight.Bold,
Margin = 10,
BorderThickness = 1,
BorderColor = Color.Black
};
twitterFollow.Click += args => Process.Start("https://twitter.com/algocornerfx");
var gumroadIconImage = new Image
{
Source = Resources.GumroadLogo,
Width = 70
};
var gumroadVisit = new Button
{
Content = gumroadIconImage,
ForegroundColor = Color.Black,
HorizontalAlignment = HorizontalAlignment.Right,
VerticalAlignment = VerticalAlignment.Top,
BackgroundColor = Color.Transparent,
FontWeight = FontWeight.Bold,
Margin = 10,
BorderThickness = 1,
BorderColor = Color.Black
};
gumroadVisit.Click += args => Process.Start("https://gumroad.com/algocorner");
contactStack.AddChild(gumroadVisit);
contactStack.AddChild(twitterFollow);
contactStack.AddChild(mailContact);
#endregion
Chart.AddControl(stackPanel);
Chart.AddControl(copyrightText);
Chart.AddControl(contactStack);
}
public override void Calculate(int index)
{
// Calculate value at specified index
// Result[index] = ...
}
}
}
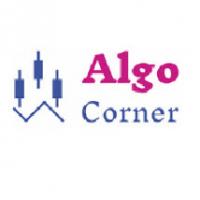
algocorner
Joined on 29.01.2021
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: AlgoCorner - Downloads.algo
- Rating: 0
- Installs: 1533
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
It would be nice if this tool is really helpful in plotting multiple moving averages. Instead of adding moving averages one at a time google feud, you can use this handy tool to draw multiple lines without much effort.