Description
This is an advanced implementation of Ichimoku Kinko Hyo.
This trading system (not indicator) takes into consideration the following theories that came along with the system;
- Wave Theory
- Time Theory
- Price Observation Theory
- The Time-Wave Theory
- Three Roles Improvement (buy signal)
- Three Roles Reversal (sell signal)
Make a Donation
- If you liked our work Consider making a Donation to help the Community Grow by Benefiting from more free Indicators and Trading Tools
Join our telegram group for more insights on how to use the trading system
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)]
public class AdvancedIchimokuAd : Indicator
{
private static string FreeVersion = "https://profitislander.gumroad.com/l/ixgth";
private static string ProVersion = "https://profitislander.gumroad.com/l/advichipro";
private static string Product = "https://profitislander.gumroad.com/l/rnpve";
private static string Contact = "kartechgh@gmail.com";
Border MainBorder;
protected override void Initialize()
{
// Initialize and create nested indicators
Init();
}
public override void Calculate(int index)
{
// Calculate value at specified index
// Result[index] = ...
}
private void Init()
{
StackPanel mainPanel = new StackPanel();
#region Header
var headerBorder = new Border
{
BorderThickness = "0 0 0 1",
Style = Styles.CreateCommonBorderStyle()
};
var headerGrid = new Grid(1, 2);
headerGrid.Columns[1].SetWidthInPixels(60);
var title = new TextBlock
{
Text = "Advanced Ichimoku Kinko Hyo Pro",
Margin = "10 7",
FontSize = 12,
Style = Styles.CreateHeaderStyle()
};
headerGrid.AddChild(title, 0, 0);
var closeBtn = new ToggleButton
{
Text = "✖",
Width = 55,
Height = 20,
FontSize = 8,
Margin = new Thickness(0, 5, 5, 5)
};
closeBtn.Click += CloseBtn_Click;
headerGrid.AddChild(closeBtn, 0, 1);
headerBorder.Child = headerGrid;
mainPanel.AddChild(headerBorder);
#endregion
#region Content Panel
var contentBorder = new Border
{
BorderThickness = "0 0 0 1",
//Height = 340,
Style = Styles.CreateCommonBorderStyle()
};
//var contentGrid = new Grid(1, 2);
StackPanel contentPanel = new StackPanel();
contentPanel.Orientation = Orientation.Vertical;
#endregion
#region Info Row
StackPanel InfoRow = new StackPanel();
InfoRow.Orientation = Orientation.Horizontal;
InfoRow.VerticalAlignment = VerticalAlignment.Center;
//InfoRow.HorizontalAlignment = HorizontalAlignment.Center;
var lbDesc = new TextBlock
{
Text = "It is not possible to download the indicator from the cTrader website. \r\n\r\nPlease visit us at \r\n\r\n" + FreeVersion,
Margin = "10 7",
Padding = "10",
FontSize = 14,
VerticalAlignment = VerticalAlignment.Center,
HorizontalAlignment = HorizontalAlignment.Center,
Style = Styles.CreateHeaderStyle()
};
InfoRow.AddChild(lbDesc);
contentPanel.AddChild(InfoRow);
#endregion
#region Button Row
StackPanel buttonRow = new StackPanel();
buttonRow.Orientation = Orientation.Horizontal;
buttonRow.HorizontalAlignment = HorizontalAlignment.Center;
buttonRow.HorizontalAlignment = HorizontalAlignment.Center;
var btn2 = new Button
{
Text = "Download",
Height = 30,
Width = 200,
FontSize = 12,
Margin = new Thickness(8, 10, 5, 10),
Style = Styles.CreateButtonStyle(Color.FromHex("#009345"), Color.FromHex("#10A651"))
};
btn2.Click += Btn2_Click;
buttonRow.AddChild(btn2);
var btn = new Button
{
Text = "Buy Indicator",
Height = 30,
Width = 200,
FontSize = 12,
Margin = new Thickness(8, 10, 5, 10),
Style = Styles.CreateButtonStyle(Color.FromHex("#009345"), Color.FromHex("#10A651"))
};
btn.Click += Btn_Click;
buttonRow.AddChild(btn);
contentPanel.AddChild(buttonRow);
#endregion
contentBorder.Child = contentPanel;
mainPanel.AddChild(contentBorder);
MainBorder = new Border
{
VerticalAlignment = VerticalAlignment.Center,
HorizontalAlignment = HorizontalAlignment.Center,
Style = Styles.CreatePanelBackgroundStyle(),
//Margin = "20 40 20 20",
Margin = new Thickness(20, 40, 20, 20),
Width = 430,
Height = 200,
Child = mainPanel
};
Chart.AddControl(MainBorder);
}
private void CloseBtn_Click(ToggleButtonEventArgs obj)
{
MainBorder.IsVisible = false;
}
private void Btn_Click(ButtonClickEventArgs obj)
{
System.Diagnostics.Process.Start(Product);
}
private void Btn2_Click(ButtonClickEventArgs obj)
{
System.Diagnostics.Process.Start(FreeVersion);
}
private void ContactBtn_Click(ButtonClickEventArgs obj)
{
System.Diagnostics.Process.Start(Contact);
}
}
public static class Styles
{
public static Style CreatePanelBackgroundStyle()
{
var style = new Style();
style.Set(ControlProperty.CornerRadius, 3);
style.Set(ControlProperty.BackgroundColor, GetColorWithOpacity(Color.FromHex("#292929"), 0.85m), ControlState.DarkTheme);
style.Set(ControlProperty.BackgroundColor, GetColorWithOpacity(Color.FromHex("#FFFFFF"), 0.85m), ControlState.LightTheme);
style.Set(ControlProperty.BorderColor, Color.FromHex("#3C3C3C"), ControlState.DarkTheme);
style.Set(ControlProperty.BorderColor, Color.FromHex("#C3C3C3"), ControlState.LightTheme);
style.Set(ControlProperty.BorderThickness, new Thickness(1));
return style;
}
public static Style CreateCommonBorderStyle()
{
var style = new Style();
style.Set(ControlProperty.BorderColor, GetColorWithOpacity(Color.FromHex("#FFFFFF"), 0.12m), ControlState.DarkTheme);
style.Set(ControlProperty.BorderColor, GetColorWithOpacity(Color.FromHex("#000000"), 0.12m), ControlState.LightTheme);
return style;
}
public static Style CreateHeaderStyle()
{
var style = new Style();
style.Set(ControlProperty.ForegroundColor, GetColorWithOpacity("#FFFFFF", 0.70m), ControlState.DarkTheme);
style.Set(ControlProperty.ForegroundColor, GetColorWithOpacity("#000000", 0.65m), ControlState.LightTheme);
return style;
}
public static Style CreateInputStyle()
{
var style = new Style(DefaultStyles.TextBoxStyle);
style.Set(ControlProperty.BackgroundColor, Color.FromHex("#1A1A1A"), ControlState.DarkTheme);
style.Set(ControlProperty.BackgroundColor, Color.FromHex("#111111"), ControlState.DarkTheme | ControlState.Hover);
style.Set(ControlProperty.BackgroundColor, Color.FromHex("#E7EBED"), ControlState.LightTheme);
style.Set(ControlProperty.BackgroundColor, Color.FromHex("#D6DADC"), ControlState.LightTheme | ControlState.Hover);
style.Set(ControlProperty.CornerRadius, 3);
return style;
}
public static Style CreateBuyButtonStyle()
{
return CreateButtonStyle(Color.FromHex("#009345"), Color.FromHex("#10A651"));
}
public static Style CreateSellButtonStyle()
{
return CreateButtonStyle(Color.FromHex("#F05824"), Color.FromHex("#FF6C36"));
}
public static Style CreateCloseButtonStyle()
{
return CreateButtonStyle(Color.FromHex("#F05824"), Color.FromHex("#FF6C36"));
}
public static Style CreateButtonStyle(Color color, Color hoverColor)
{
var style = new Style(DefaultStyles.ButtonStyle);
style.Set(ControlProperty.BackgroundColor, color, ControlState.DarkTheme);
style.Set(ControlProperty.BackgroundColor, color, ControlState.LightTheme);
style.Set(ControlProperty.BackgroundColor, hoverColor, ControlState.DarkTheme | ControlState.Hover);
style.Set(ControlProperty.BackgroundColor, hoverColor, ControlState.LightTheme | ControlState.Hover);
style.Set(ControlProperty.ForegroundColor, Color.FromHex("#FFFFFF"), ControlState.DarkTheme);
style.Set(ControlProperty.ForegroundColor, Color.FromHex("#FFFFFF"), ControlState.LightTheme);
return style;
}
public static Style CreateToggleButtonStyle(Color color, Color checkedColor)
{
var style = new Style(DefaultStyles.ToggleButtonStyle);
style.Set(ControlProperty.BackgroundColor, color, ControlState.DarkTheme);
style.Set(ControlProperty.BackgroundColor, color, ControlState.LightTheme);
style.Set(ControlProperty.BackgroundColor, checkedColor, ControlState.DarkTheme | ControlState.Checked);
style.Set(ControlProperty.BackgroundColor, checkedColor, ControlState.LightTheme | ControlState.Checked);
style.Set(ControlProperty.BackgroundColor, checkedColor, ControlState.DarkTheme | ControlState.Hover);
style.Set(ControlProperty.BackgroundColor, checkedColor, ControlState.LightTheme | ControlState.Hover);
style.Set(ControlProperty.ForegroundColor, Color.FromHex("#FFFFFF"), ControlState.DarkTheme);
style.Set(ControlProperty.ForegroundColor, Color.FromHex("#FFFFFF"), ControlState.LightTheme);
return style;
}
private static Color GetColorWithOpacity(Color baseColor, decimal opacity)
{
var alpha = (int)Math.Round(byte.MaxValue * opacity, MidpointRounding.AwayFromZero);
return Color.FromArgb(alpha, baseColor);
}
}
}
Profit_Islander
Joined on 04.01.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Advanced Ichimoku Ad.algo
- Rating: 5
- Installs: 2891
Comments
leonardorico.vieira check out Advanced Ichimoku Pro
rinaktariza.ro check out ProfitSense
@rinaktariza.ro check out ProfitSense
Hello kyerematics, your work is amazing, you truly spend time coding and learning all about this trade system, congrats. If i can, want ask you only one improvement, it is possible put some alerts when the price break out the cloud? Tks in advanced, and tks to this work.
Dear Kyerematics, can help to add commands for back test and autotrade?
thanks a lot. this strategy is awesome.
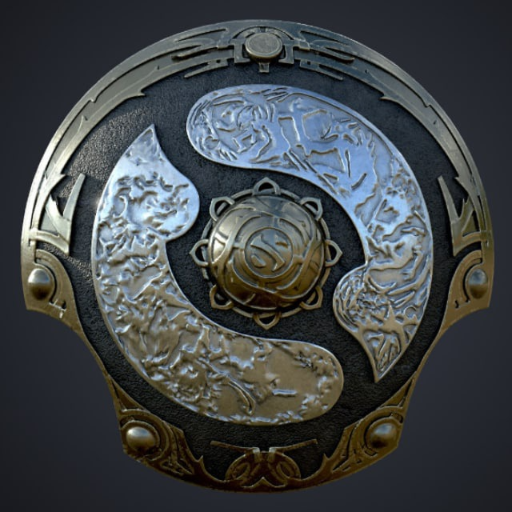
thanks a lot!
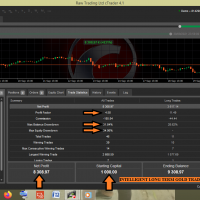
nice work.
it's a bit confusing though .
ok
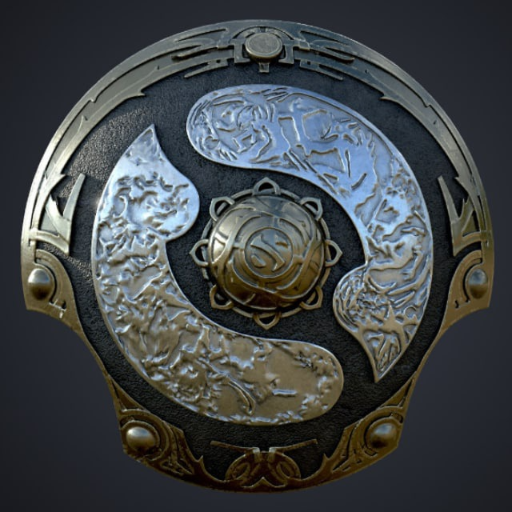
Hi,
Thank you for this system,
Would be possible to insert buy/sell arrows into it?
Thanks for the system not indicator.
It would very nice to add Kumo (Cloud) color user define option?