Description
Weis Wave is a Very known indicator by Follower of Wyckoff, VSA, VPA and Order Flow Trading Style. The Indicator was created by David Weis and further developed on NinjaTrader and widely known as MBox Wave. Here it is our Experto Version Brought to Ctrader for Free, Enjoy It.
You can find me by joyining this Telegram Group http://t.me/cTraderCoders
Grupo de Telegram Para Brasileiros, Portugueses e todos aqueles que falam portugues:http://t.me/ComunidadeCtrader
Grupo CTrader en Español para Latinos: http://t.me/ComunidadCtrader
using System;
using cAlgo.API;
using System.Linq;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class WeisWavesTraderExperto : Indicator
{
public enum Multi
{
Pips = 10,
Unit = 100,
Point = 1
}
public enum devi
{
Price = 1,
ATR = 2
}
[Parameter("Deviation Size", Group = "Deviations Settings", DefaultValue = Multi.Unit)]
public Multi Multiplier { get; set; }
[Parameter("Deviation", Group = "Deviations Settings", DefaultValue = 50, MinValue = 1)]
public int Deviation { get; set; }
[Parameter("Sell Histogram Negative", Group = "Histogram Settings", DefaultValue = false)]
public bool change { get; set; }
[Output("UpVolume", LineColor = "DarkViolet", PlotType = PlotType.Histogram, Thickness = 5)]
public IndicatorDataSeries UpVolume { get; set; }
[Output("DownVolume", LineColor = "White", PlotType = PlotType.Histogram, Thickness = 5)]
public IndicatorDataSeries DownVolume { get; set; }
[Parameter("Atr Multiplier", Group = "ATR Settings", DefaultValue = 1.5, MinValue = 0, MaxValue = 10)]
public double atrMultiplier { get; set; }
[Parameter("Atr Period", Group = "ATR Settings", DefaultValue = 13, MinValue = 1)]
public int atrPeriod { get; set; }
[Parameter("Deviation Type", Group = "Deviations Settings", DefaultValue = devi.ATR)]
public devi deviationType { get; set; }
private int lastHighIndex = 0;
private int lastLowIndex = 0;
private double _point;
private DataSeries CurrentClose;
private IndicatorDataSeries Direction;
private IndicatorDataSeries DownBuffer;
private AverageTrueRange _averageTrueRange;
private double DeviationAtr;
private bool deviationPrice = true;
private double deviationValue;
protected override void Initialize()
{
Direction = CreateDataSeries();
CurrentClose = Bars.ClosePrices;
DownBuffer = CreateDataSeries();
_point = Symbol.TickSize * (double)Multiplier;
_averageTrueRange = Indicators.AverageTrueRange(atrPeriod, MovingAverageType.Weighted);
if (deviationType == devi.ATR)
{
_point = 1;
deviationPrice = false;
}
else
{
deviationPrice = true;
}
}
public override void Calculate(int index)
{
if (deviationPrice)
{
deviationValue = Deviation;
}
else
{
DeviationAtr = _averageTrueRange.Result[index] * atrMultiplier;
deviationValue = DeviationAtr;
}
int r = 0;
if (index == 1)
{
if (CurrentClose[index] >= Bars.ClosePrices[index - 1])
{
lastHighIndex = index;
lastLowIndex = index - 1;
Direction[index] = 1;
Direction[index - 1] = 1;
UpVolume[index - 1] = Bars.TickVolumes[index - 1];
UpVolume[index] = UpVolume[index - 1] + Bars.TickVolumes[index];
}
else if (CurrentClose[index] < Bars.ClosePrices[index - 1])
{
lastLowIndex = index;
lastHighIndex = index - 1;
Direction[index] = 3;
Direction[index - 1] = 3;
DownBuffer[index - 1] = Bars.TickVolumes[index - 1];
DownBuffer[index] = DownBuffer[index - 1] + Bars.TickVolumes[index];
DownVolume[index - 1] = isNegative(DownBuffer[index - 1]);
DownVolume[index] = isNegative(DownBuffer[index]);
//**********************************************************//
}
}
Direction[index] = Direction[index - 1];
if (Direction[index] == 1)
{
if (Bars.HighPrices[lastHighIndex] - CurrentClose[index] > (deviationValue * _point))
{
Direction[index] = 3;
}
}
else if (Direction[index] == 3)
{
if (Bars.ClosePrices[index] - Bars.LowPrices[lastLowIndex] > (deviationValue * _point))
{
Direction[index] = 1;
}
}
if (Direction[index] == 1)
{
if (Direction[index - 1] == 1)
{
UpVolume[index] = UpVolume[index - 1] + Bars.TickVolumes[index];
DownVolume[index] = 0;
if (CurrentClose[lastHighIndex] < CurrentClose[index])
{
lastHighIndex = index;
}
}
else if (Direction[index - 1] == 3)
{
UpVolume[lastLowIndex + 1] = Bars.TickVolumes[lastLowIndex + 1];
DownVolume[lastLowIndex + 1] = 0;
for (r = (lastLowIndex + 2); r <= index; r++)
{
UpVolume[r] = UpVolume[r - 1] + Bars.TickVolumes[r];
DownVolume[r] = 0;
}
lastHighIndex = index;
}
}
else if (Direction[index] == 3)
{
if (Direction[index - 1] == 3)
{
DownBuffer[index] = DownBuffer[index - 1] + Bars.TickVolumes[index];
DownVolume[index] = isNegative(DownBuffer[index]);
UpVolume[index] = 0;
if (CurrentClose[index] < CurrentClose[lastLowIndex])
{
lastLowIndex = index;
}
}
else if (Direction[index - 1] == 1)
{
DownBuffer[lastHighIndex + 1] = Bars.TickVolumes[lastHighIndex + 1];
DownVolume[lastHighIndex + 1] = isNegative(DownBuffer[lastHighIndex + 1]);
UpVolume[lastHighIndex + 1] = 0;
for (r = (lastHighIndex + 2); r <= index; r++)
{
DownBuffer[r] = DownBuffer[r - 1] + Bars.TickVolumes[r];
DownVolume[r] = isNegative(DownBuffer[r]);
UpVolume[r] = 0;
}
lastLowIndex = index;
}
}
}
public double isNegative(double number)
{
double val = 0;
if (!change)
{
val = number;
}
else if (change)
{
val = -number;
}
return val;
}
}
}
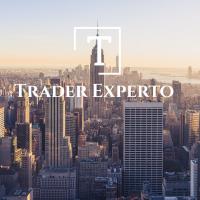
TraderExperto
Joined on 07.06.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Weis Waves Trader Experto.algo
- Rating: 5
- Installs: 3773
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Can we please get a Non repainting version?