Description
The original aim was to use this as a stoploss that rapidly closes the gap on price if there was a potential reversal. The Waddah Attah Adaptive MA (WAMA) uses the explosion values from the Waddah Attah as an adaptive parameter that allows us to filter the price series using the smoothing of moving averages.
To see the projects (indicators, cBots and data science analysis) we're currently working on, you can join our Telegram chat here: t.me/quantFXAlgos
We also have a slight ask from people who download: we're currently looking to get renko shadows / wicks / range / tails introduced as a feature in cTrader.
You can help them be introduced by voting in the cTrader suggestions forum for this post: https://ctrader.com/forum/suggestions/29955
Cheers all
Shown here are:
- WAMA in uncut Cyan.
- 2-Period EMA in Dashed Cyan
- 30-Period EMA in Dashed Purple
Parameters:
- Fast MA Period: The period of the Fast MA that the ROC MA will follow
- Slow MA Period: The period of the Slow MA that the ROC MA will follow
- MA Type: The type of fast and slow MA to follow.
- MA To Follow: When alpha is large (in this case, when absolute momentum is large) this will be the MA that the ROC MA will follow closest.
- MACD Long Cycle, MACD Short Cycle: The two traditional MACD parameters that are used to determine the WA Explosion.
- WA Scale Type: Max, or Odds. Max scales alpha (the adaptive parameter) into the [0,1] range by using the input from ROC Max (below). Odds scales alpha using alpha = alpha / (alpha + 1).
- WA Max: The Maximum WA Explosion you expect in the period, any values large than this are scaled so that alpha = 1.
- WA-MA Balance Type: Alpha, InvAlpha, Manual. This controls the smoothing between new values and previous values. For manual, see below. If Alpha is selected, then when alpha is large new results will be favoured more heavily. If InvAlpha is selected, then when Alpha is large, the previous value is weighted more heavily (a smoother MA).
- WA-MA Balance: If Manual is selected for the balance type, then this is the smoothing between the new result and old result. Values close to 1 favour the new result and give a less-smooth MA.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class WAMA : Indicator
{
[Parameter("Fast MA Period", Group = "MA Settings", DefaultValue = 2)]
public int FastPeriod { get; set; }
[Parameter("Slow MA Period", Group = "MA Settings", DefaultValue = 30)]
public int SlowPeriod { get; set; }
[Parameter("MAType", Group = "MA Settings", DefaultValue = MovingAverageType.Exponential)]
public MovingAverageType MAType { get; set; }
[Parameter("MA To Follow", Group = "MA Settings", DefaultValue = Adherence.Fast)]
public Adherence MAtoFollow { get; set; }
public enum Adherence
{
Fast,
Slow
}
public enum ScaleType
{
Max,
Odds
}
public enum SmoothType
{
Alpha,
InvAlpha,
Manual
}
[Parameter("MACD Long Cycle", Group = "MACD Settings", DefaultValue = 26)]
public int LongCycle { get; set; }
[Parameter("MACD Short Cycle", Group = "MACD Settings", DefaultValue = 12)]
public int ShortCycle { get; set; }
[Parameter("WA Scale Type", Group = "Adaptive Settings", DefaultValue = ScaleType.Odds)]
public ScaleType ScaleSettings { get; set; }
[Parameter("WA Max", Group = "Adaptive Settings", DefaultValue = 10)]
public double WaMax { get; set; }
[Parameter("WA-MA Balance Type", Group = "Adaptive Settings", DefaultValue = SmoothType.InvAlpha)]
public SmoothType BalanceType { get; set; }
[Parameter("WA-MA Balance", Group = "Adaptive Settings", DefaultValue = 0.5, MinValue = 0, MaxValue = 1)]
public double Balance { get; set; }
[Output("Main", LineColor = "DodgerBlue")]
public IndicatorDataSeries Result { get; set; }
private MovingAverage fastMA, slowMA;
private MacdCrossOver macdCrossOver;
protected override void Initialize()
{
fastMA = Indicators.MovingAverage(Bars.ClosePrices, FastPeriod, MAType);
slowMA = Indicators.MovingAverage(Bars.ClosePrices, SlowPeriod, MAType);
macdCrossOver = Indicators.MacdCrossOver(Bars.ClosePrices, LongCycle, ShortCycle, 9);
}
public override void Calculate(int index)
{
// Take care of Nan Values:
if (double.IsNaN(Result[index - 1]))
{
Result[index] = Bars.ClosePrices[index];
return;
}
// Change macd value to explosion and then multiply by standard sensitivity:
double explosion = 100 * Math.Abs((macdCrossOver.MACD[index] - macdCrossOver.MACD[index - 1]));
if (double.IsNaN(explosion))
explosion = WaMax / 2;
// Calculate alpha:
double alpha;
if (ScaleSettings == ScaleType.Odds)
alpha = explosion / (explosion + 1);
else
alpha = explosion / WaMax;
if (alpha > 1)
alpha = 1;
// Calculate the adjustment:
double newAdjustment;
if (MAtoFollow == Adherence.Fast)
newAdjustment = alpha * fastMA.Result[index] + (1 - alpha) * slowMA.Result[index];
else
newAdjustment = (1 - alpha) * fastMA.Result[index] + alpha * slowMA.Result[index];
// Calculate the result:
if (BalanceType == SmoothType.Alpha)
Result[index] = (1 - alpha) * Result[index - 1] + alpha * newAdjustment;
else if (BalanceType == SmoothType.InvAlpha)
Result[index] = alpha * Result[index - 1] + (1 - alpha) * newAdjustment;
else
Result[index] = (1 - Balance) * Result[index - 1] + Balance * newAdjustment;
}
}
}
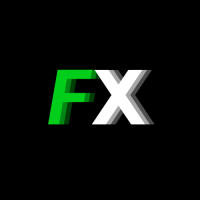
fxtradersystems
Joined on 10.09.2020
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: WAMA.algo
- Rating: 0
- Installs: 1572
- Modified: 13/10/2021 09:54