Description
The Renko Range indicator fetches the high-low watermarks of each renko bar.
It's calculated using the values of the M1 bars between the open and close times of the renko bar, and so you will see odd results on volatile pairs who complete renko bars in under 1 minute.
The "Load to date" parameter is in the form DD/MM/YYYY and allows you to load more M1 bars to extend how far back the high-low dots load without scrolling backwards along your chart.
The "Draw as wicks?" option means that the indicator will fill in the gaps with chart trend lines like proper wicks.
The "Cut odd points?" option automatically sets the (high / low) of a completed (up / down) renko bar as the (top / bottom) of the bar respectively.
Any requests for indicators, you can email: development@fxtradersystems.com
Or can reach out via Telegram at: https://t.me/samwhitefx
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
using System.Linq;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class RenkoRange : Indicator
{
[Parameter("Load to (dd/mm/yyyy):", DefaultValue = "01/01/2019")]
public string LoadDate { get; set; }
[Parameter("Draw as wicks?", DefaultValue = true)]
public bool DrawWicks { get; set; }
[Parameter("Cut odd points?", DefaultValue = true)]
public bool CutPoints { get; set; }
private DateTime dateTime;
private Bars customBars;
[Output("High", PlotType = PlotType.Points, LineColor = "Lime", Thickness = 2)]
public IndicatorDataSeries High { get; set; }
[Output("Low", PlotType = PlotType.Points, LineColor = "Red", Thickness = 2)]
public IndicatorDataSeries Low { get; set; }
protected override void Initialize()
{
customBars = MarketData.GetBars(TimeFrame.Minute);
// Get datetime to load until:
string[] pieces = LoadDate.Split('/');
dateTime = new DateTime(int.Parse(pieces[2]), int.Parse(pieces[1]), int.Parse(pieces[0]));
Print("{0} bar on the chart. Loading 10 000 bars", Bars.Count);
while (customBars.OpenTimes.Reverse().LastOrDefault() > dateTime)
{
var loadedCount = customBars.LoadMoreHistory();
Print("Loaded {0} bars", loadedCount);
if (loadedCount == 0)
break;
}
Print("Finished, earliest open: {0}", customBars.OpenTimes.Reverse().LastOrDefault());
}
public override void Calculate(int index)
{
int startIndex = customBars.OpenTimes.GetIndexByTime(Bars.OpenTimes[index]);
// on the one minute timeframe, where do we start?
int endIndex;
if (IsLastBar)
endIndex = customBars.OpenTimes.GetIndexByTime(Server.Time);
else
endIndex = customBars.OpenTimes.GetIndexByTime(Bars.OpenTimes[index + 1]);
double high, low;
high = double.NegativeInfinity;
low = double.PositiveInfinity;
for (int count = startIndex; count <= endIndex; count++)
{
double newHigh = customBars.HighPrices[count];
double newLow = customBars.LowPrices[count];
if (newLow < low)
low = newLow;
if (newHigh > high)
high = newHigh;
}
bool isUpBar = Bars.ClosePrices[index] > Bars.OpenPrices[index];
if (!IsLastBar && CutPoints)
{
if (isUpBar)
high = Bars.ClosePrices[index];
else
low = Bars.LowPrices[index];
}
High[index] = high;
Low[index] = low;
if (DrawWicks)
{
Color color = isUpBar ? Color.Green : Color.Orange;
Chart.RemoveObject("Renko Wick " + index);
Chart.DrawTrendLine("Renko Wick " + index, index, high, index, low, color, 1);
}
}
}
}
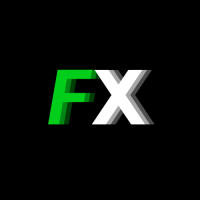
fxtradersystems
Joined on 10.09.2020
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Renko Range.algo
- Rating: 0
- Installs: 1891
- Modified: 13/10/2021 09:54
Changelog 1:
- Added the options to draw wicks
- Added the option to cut wicks at the top / bottom of the candle depending on whether it was an up / down bar